CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_mean_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mean_f32.c 00009 * 00010 * Description: Mean value of two floating point arrays. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * ---------------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupStats 00031 */ 00032 00033 /** 00034 * @defgroup mean Mean 00035 * 00036 * Calculates the mean of the input vector. Mean is defined as the average of the elements in the vector. 00037 * The underlying algorithm is used: 00038 * 00039 * <pre> 00040 * Result = (pSrc[0] + pSrc[1] + pSrc[2] + ... + pSrc[blockSize-1]) / blockSize; 00041 * </pre> 00042 * 00043 * There are separate functions for floating-point, Q31, Q15, and Q7 data types. 00044 */ 00045 00046 /** 00047 * @addtogroup mean 00048 * @{ 00049 */ 00050 00051 00052 /** 00053 * @brief Mean value of a floating-point vector. 00054 * @param[in] *pSrc points to the input vector 00055 * @param[in] blockSize length of the input vector 00056 * @param[out] *pResult mean value returned here 00057 * @return none. 00058 */ 00059 00060 00061 void arm_mean_f32( 00062 float32_t * pSrc, 00063 uint32_t blockSize, 00064 float32_t * pResult) 00065 { 00066 float32_t sum = 0.0f; /* Temporary result storage */ 00067 uint32_t blkCnt; /* loop counter */ 00068 00069 /*loop Unrolling */ 00070 blkCnt = blockSize >> 2u; 00071 00072 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00073 ** a second loop below computes the remaining 1 to 3 samples. */ 00074 while(blkCnt > 0u) 00075 { 00076 /* C = (A[0] + A[1] + A[2] + ... + A[blockSize-1]) */ 00077 sum += *pSrc++; 00078 sum += *pSrc++; 00079 sum += *pSrc++; 00080 sum += *pSrc++; 00081 00082 /* Decrement the loop counter */ 00083 blkCnt--; 00084 } 00085 00086 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00087 ** No loop unrolling is used. */ 00088 blkCnt = blockSize % 0x4u; 00089 00090 while(blkCnt > 0u) 00091 { 00092 /* C = (A[0] + A[1] + A[2] + ... + A[blockSize-1]) */ 00093 sum += *pSrc++; 00094 00095 /* Decrement the loop counter */ 00096 blkCnt--; 00097 } 00098 00099 /* C = (A[0] + A[1] + A[2] + ... + A[blockSize-1]) / blockSize */ 00100 /* Store the result to the destination */ 00101 *pResult = sum / (float32_t) blockSize; 00102 } 00103 00104 /** 00105 * @} end of mean group 00106 */
Generated on Tue Jul 12 2022 14:13:54 by
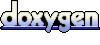