CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_mat_trans_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_trans_q15.c 00009 * 00010 * Description: Q15 matrix transpose. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupMatrix 00037 */ 00038 00039 /** 00040 * @addtogroup MatrixTrans 00041 * @{ 00042 */ 00043 00044 /* 00045 * @brief Q15 matrix transpose. 00046 * @param[in] *pSrc points to the input matrix 00047 * @param[out] *pDst points to the output matrix 00048 * @return The function returns either <code>ARM_MATH_SIZE_MISMATCH</code> 00049 * or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00050 */ 00051 00052 arm_status arm_mat_trans_q15( 00053 const arm_matrix_instance_q15 * pSrc, 00054 arm_matrix_instance_q15 * pDst) 00055 { 00056 q15_t *pSrcA = pSrc->pData; /* input data matrix pointer */ 00057 q15_t *pOut = pDst->pData; /* output data matrix pointer */ 00058 // q15_t *pDst = pDst->pData; /* output data matrix pointer */ 00059 uint16_t nRows = pSrc->numRows; /* number of nRows */ 00060 uint16_t nColumns = pSrc->numCols; /* number of nColumns */ 00061 uint16_t col, row = nRows, i = 0u; /* row and column loop counters */ 00062 q31_t in; /* variable to hold temporary output */ 00063 arm_status status; /* status of matrix transpose */ 00064 00065 00066 #ifdef ARM_MATH_MATRIX_CHECK 00067 /* Check for matrix mismatch condition */ 00068 if((pSrc->numRows != pDst->numCols) || (pSrc->numCols != pDst->numRows)) 00069 { 00070 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00071 status = ARM_MATH_SIZE_MISMATCH; 00072 } 00073 else 00074 #endif 00075 { 00076 /* Matrix transpose by exchanging the rows with columns */ 00077 /* row loop */ 00078 do 00079 { 00080 /* Apply loop unrolling and exchange the columns with row elements */ 00081 col = nColumns >> 2u; 00082 00083 /* The pointer pOut is set to starting address of the column being processed */ 00084 pOut = pDst->pData + i; 00085 00086 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00087 ** a second loop below computes the remaining 1 to 3 samples. */ 00088 while(col > 0u) 00089 { 00090 /* Read two elements from the row */ 00091 in = *__SIMD32(pSrcA)++; 00092 00093 /* Unpack and store one element in the destination */ 00094 *pOut = (q15_t) in; 00095 00096 /* Update the pointer pOut to point to the next row of the transposed matrix */ 00097 pOut += nRows; 00098 00099 /* Unpack and store the second element in the destination */ 00100 *pOut = (q15_t) ((in & (q31_t) 0xffff0000) >> 16); 00101 00102 /* Update the pointer pOut to point to the next row of the transposed matrix */ 00103 pOut += nRows; 00104 00105 /* Read two elements from the row */ 00106 in = *__SIMD32(pSrcA)++; 00107 00108 /* Unpack and store one element in the destination */ 00109 *pOut = (q15_t) in; 00110 00111 /* Update the pointer pOut to point to the next row of the transposed matrix */ 00112 pOut += nRows; 00113 00114 /* Unpack and store the second element in the destination */ 00115 *pOut = (q15_t) ((in & (q31_t) 0xffff0000) >> 16); 00116 00117 /* Update the pointer pOut to point to the next row of the transposed matrix */ 00118 pOut += nRows; 00119 00120 /* Decrement the column loop counter */ 00121 col--; 00122 } 00123 00124 /* Perform matrix transpose for last 3 samples here. */ 00125 col = nColumns % 0x4u; 00126 00127 while(col > 0u) 00128 { 00129 /* Read and store the input element in the destination */ 00130 *pOut = *pSrcA++; 00131 00132 /* Update the pointer pOut to point to the next row of the transposed matrix */ 00133 pOut += nRows; 00134 00135 /* Decrement the column loop counter */ 00136 col--; 00137 } 00138 00139 i++; 00140 00141 /* Decrement the row loop counter */ 00142 row--; 00143 00144 } while(row > 0u); 00145 00146 /* set status as ARM_MATH_SUCCESS */ 00147 status = ARM_MATH_SUCCESS; 00148 } 00149 /* Return to application */ 00150 return (status); 00151 } 00152 00153 /** 00154 * @} end of MatrixTrans group 00155 */
Generated on Tue Jul 12 2022 14:13:53 by
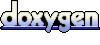