CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_mat_sub_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_sub_f32.c 00009 * 00010 * Description: Floating-point matrix subtraction. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupMatrix 00037 */ 00038 00039 /** 00040 * @defgroup MatrixSub Matrix Subtraction 00041 * 00042 * Subtract two matrices. 00043 * \image html MatrixSubtraction.gif "Subraction of two 3 x 3 matrices" 00044 * 00045 * The functions check to make sure that 00046 * <code>pSrcA</code>, <code>pSrcB</code>, and <code>pDst</code> have the same 00047 * number of rows and columns. 00048 */ 00049 00050 /** 00051 * @addtogroup MatrixSub 00052 * @{ 00053 */ 00054 00055 /** 00056 * @brief Floating-point matrix subtraction 00057 * @param[in] *pSrcA points to the first input matrix structure 00058 * @param[in] *pSrcB points to the second input matrix structure 00059 * @param[out] *pDst points to output matrix structure 00060 * @return The function returns either 00061 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00062 */ 00063 00064 arm_status arm_mat_sub_f32( 00065 const arm_matrix_instance_f32 * pSrcA, 00066 const arm_matrix_instance_f32 * pSrcB, 00067 arm_matrix_instance_f32 * pDst) 00068 { 00069 float32_t *pIn1 = pSrcA->pData; /* input data matrix pointer A */ 00070 float32_t *pIn2 = pSrcB->pData; /* input data matrix pointer B */ 00071 float32_t *pOut = pDst->pData; /* output data matrix pointer */ 00072 uint32_t numSamples; /* total number of elements in the matrix */ 00073 uint32_t blkCnt; /* loop counters */ 00074 arm_status status; /* status of matrix subtraction */ 00075 00076 #ifdef ARM_MATH_MATRIX_CHECK 00077 /* Check for matrix mismatch condition */ 00078 if((pSrcA->numRows != pSrcB->numRows) || 00079 (pSrcA->numCols != pSrcB->numCols) || 00080 (pSrcA->numRows != pDst->numRows) || (pSrcA->numCols != pDst->numCols)) 00081 { 00082 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00083 status = ARM_MATH_SIZE_MISMATCH; 00084 } 00085 else 00086 #endif 00087 { 00088 /* Total number of samples in the input matrix */ 00089 numSamples = (uint32_t) pSrcA->numRows * pSrcA->numCols; 00090 00091 /* Loop Unrolling */ 00092 blkCnt = numSamples >> 2u; 00093 00094 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00095 ** a second loop below computes the remaining 1 to 3 samples. */ 00096 while(blkCnt > 0u) 00097 { 00098 /* C(m,n) = A(m,n) - B(m,n) */ 00099 /* Subtract and then store the results in the destination buffer. */ 00100 *pOut++ = (*pIn1++) - (*pIn2++); 00101 *pOut++ = (*pIn1++) - (*pIn2++); 00102 *pOut++ = (*pIn1++) - (*pIn2++); 00103 *pOut++ = (*pIn1++) - (*pIn2++); 00104 00105 /* Decrement the loop counter */ 00106 blkCnt--; 00107 } 00108 00109 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00110 ** No loop unrolling is used. */ 00111 blkCnt = numSamples % 0x4u; 00112 00113 while(blkCnt > 0u) 00114 { 00115 /* C(m,n) = A(m,n) - B(m,n) */ 00116 /* Subtract and then store the results in the destination buffer. */ 00117 *pOut++ = (*pIn1++) - (*pIn2++); 00118 00119 /* Decrement the loop counter */ 00120 blkCnt--; 00121 } 00122 00123 /* Set status as ARM_MATH_SUCCESS */ 00124 status = ARM_MATH_SUCCESS; 00125 } 00126 00127 /* Return to application */ 00128 return (status); 00129 } 00130 00131 /** 00132 * @} end of MatrixSub group 00133 */
Generated on Tue Jul 12 2022 14:13:53 by
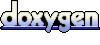