CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_mat_add_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_add_q31.c 00009 * 00010 * Description: Q31 matrix addition 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupMatrix 00037 */ 00038 00039 /** 00040 * @addtogroup MatrixAdd 00041 * @{ 00042 */ 00043 00044 /** 00045 * @brief Q31 matrix addition. 00046 * @param[in] *pSrcA points to the first input matrix structure 00047 * @param[in] *pSrcB points to the second input matrix structure 00048 * @param[out] *pDst points to output matrix structure 00049 * @return The function returns either 00050 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00051 * 00052 * <b>Scaling and Overflow Behavior:</b> 00053 * \par 00054 * The function uses saturating arithmetic. 00055 * Results outside of the allowable Q31 range [0x80000000 0x7FFFFFFF] will be saturated. 00056 */ 00057 00058 arm_status arm_mat_add_q31( 00059 const arm_matrix_instance_q31 * pSrcA, 00060 const arm_matrix_instance_q31 * pSrcB, 00061 arm_matrix_instance_q31 * pDst) 00062 { 00063 q31_t *pIn1 = pSrcA->pData; /* input data matrix pointer A */ 00064 q31_t *pIn2 = pSrcB->pData; /* input data matrix pointer B */ 00065 q31_t *pOut = pDst->pData; /* output data matrix pointer */ 00066 uint32_t numSamples; /* total number of elements in the matrix */ 00067 uint32_t blkCnt; /* loop counters */ 00068 arm_status status; /* status of matrix addition */ 00069 00070 #ifdef ARM_MATH_MATRIX_CHECK 00071 /* Check for matrix mismatch condition */ 00072 if((pSrcA->numRows != pSrcB->numRows) || 00073 (pSrcA->numCols != pSrcB->numCols) || 00074 (pSrcA->numRows != pDst->numRows) || (pSrcA->numCols != pDst->numCols)) 00075 { 00076 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00077 status = ARM_MATH_SIZE_MISMATCH; 00078 } 00079 else 00080 #endif 00081 { 00082 /* Total number of samples in the input matrix */ 00083 numSamples = (uint32_t) pSrcA->numRows * pSrcA->numCols; 00084 00085 /* Loop Unrolling */ 00086 blkCnt = numSamples >> 2u; 00087 00088 00089 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00090 ** a second loop below computes the remaining 1 to 3 samples. */ 00091 while(blkCnt > 0u) 00092 { 00093 /* C(m,n) = A(m,n) + B(m,n) */ 00094 /* Add, saturate and then store the results in the destination buffer. */ 00095 *pOut++ = __QADD(*pIn1++, *pIn2++); 00096 *pOut++ = __QADD(*pIn1++, *pIn2++); 00097 *pOut++ = __QADD(*pIn1++, *pIn2++); 00098 *pOut++ = __QADD(*pIn1++, *pIn2++); 00099 00100 /* Decrement the loop counter */ 00101 blkCnt--; 00102 } 00103 00104 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00105 ** No loop unrolling is used. */ 00106 blkCnt = numSamples % 0x4u; 00107 00108 while(blkCnt > 0u) 00109 { 00110 /* C(m,n) = A(m,n) + B(m,n) */ 00111 /* Add, saturate and then store the results in the destination buffer. */ 00112 *pOut++ = __QADD(*pIn1++, *pIn2++); 00113 00114 /* Decrement the loop counter */ 00115 blkCnt--; 00116 } 00117 00118 /* set status as ARM_MATH_SUCCESS */ 00119 status = ARM_MATH_SUCCESS; 00120 } 00121 00122 /* Return to application */ 00123 return (status); 00124 } 00125 00126 /** 00127 * @} end of MatrixAdd group 00128 */
Generated on Tue Jul 12 2022 14:13:53 by
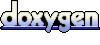