CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_float_to_q15.c
00001 /* ---------------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_float_to_q15.c 00009 * 00010 * Description: Processing function for the Conversion from float to Q15 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * ---------------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupSupport 00031 */ 00032 00033 /** 00034 * @addtogroup float_to_x 00035 * @{ 00036 */ 00037 00038 /** 00039 * @brief Converts the elements of the floating-point vector to Q15 vector. 00040 * @param[in] *pSrc points to the floating-point input vector 00041 * @param[out] *pDst points to the Q15 output vector 00042 * @param[in] blockSize length of the input vector 00043 * @return none. 00044 * 00045 * \par Description: 00046 * \par 00047 * The equation used for the conversion process is: 00048 * <pre> 00049 * pDst[n] = (q15_t)(pSrc[n] * 32768); 0 <= n < blockSize. 00050 * </pre> 00051 * \par Scaling and Overflow Behavior: 00052 * \par 00053 * The function uses saturating arithmetic. 00054 * Results outside of the allowable Q15 range [0x8000 0x7FFF] will be saturated. 00055 * \note 00056 * In order to apply rounding, the library should be rebuilt with the ROUNDING macro 00057 * defined in the preprocessor section of project options. 00058 * 00059 */ 00060 00061 00062 void arm_float_to_q15( 00063 float32_t * pSrc, 00064 q15_t * pDst, 00065 uint32_t blockSize) 00066 { 00067 float32_t *pIn = pSrc; /* Src pointer */ 00068 uint32_t blkCnt; /* loop counter */ 00069 00070 #ifdef ARM_MATH_ROUNDING 00071 00072 float32_t in; 00073 00074 #endif 00075 00076 /*loop Unrolling */ 00077 blkCnt = blockSize >> 2u; 00078 00079 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00080 ** a second loop below computes the remaining 1 to 3 samples. */ 00081 while(blkCnt > 0u) 00082 { 00083 00084 #ifdef ARM_MATH_ROUNDING 00085 /* C = A * 32768 */ 00086 /* convert from float to q15 and then store the results in the destination buffer */ 00087 in = *pIn++; 00088 in = (in * 32768.0f); 00089 in += in > 0 ? 0.5 : -0.5; 00090 *pDst++ = (q15_t) (__SSAT((q31_t) (in), 16)); 00091 00092 in = *pIn++; 00093 in = (in * 32768.0f); 00094 in += in > 0 ? 0.5 : -0.5; 00095 *pDst++ = (q15_t) (__SSAT((q31_t) (in), 16)); 00096 00097 in = *pIn++; 00098 in = (in * 32768.0f); 00099 in += in > 0 ? 0.5 : -0.5; 00100 *pDst++ = (q15_t) (__SSAT((q31_t) (in), 16)); 00101 00102 in = *pIn++; 00103 in = (in * 32768.0f); 00104 in += in > 0 ? 0.5 : -0.5; 00105 *pDst++ = (q15_t) (__SSAT((q31_t) (in), 16)); 00106 00107 #else 00108 00109 /* C = A * 32768 */ 00110 /* convert from float to q15 and then store the results in the destination buffer */ 00111 *pDst++ = (q15_t) __SSAT((q31_t) (*pIn++ * 32768.0f), 16); 00112 *pDst++ = (q15_t) __SSAT((q31_t) (*pIn++ * 32768.0f), 16); 00113 *pDst++ = (q15_t) __SSAT((q31_t) (*pIn++ * 32768.0f), 16); 00114 *pDst++ = (q15_t) __SSAT((q31_t) (*pIn++ * 32768.0f), 16); 00115 00116 #endif 00117 00118 /* Decrement the loop counter */ 00119 blkCnt--; 00120 } 00121 00122 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00123 ** No loop unrolling is used. */ 00124 blkCnt = blockSize % 0x4u; 00125 00126 while(blkCnt > 0u) 00127 { 00128 00129 #ifdef ARM_MATH_ROUNDING 00130 /* C = A * 32768 */ 00131 /* convert from float to q15 and then store the results in the destination buffer */ 00132 in = *pIn++; 00133 in = (in * 32768LL); 00134 in += in > 0 ? 0.5 : -0.5; 00135 *pDst++ = (q15_t) (__SSAT((q31_t) (in), 16)); 00136 00137 #else 00138 00139 /* C = A * 32768 */ 00140 /* convert from float to q15 and then store the results in the destination buffer */ 00141 *pDst++ = (q15_t) __SSAT((q31_t) (*pIn++ * 32768.0f), 16); 00142 00143 #endif 00144 00145 /* Decrement the loop counter */ 00146 blkCnt--; 00147 } 00148 } 00149 00150 /** 00151 * @} end of float_to_x group 00152 */
Generated on Tue Jul 12 2022 14:13:53 by
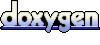