CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_fir_init_q7.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_fir_init_q7.c 00009 * 00010 * Description: Q7 FIR filter initialization function. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * ------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupFilters 00037 */ 00038 00039 /** 00040 * @addtogroup FIR 00041 * @{ 00042 */ 00043 /** 00044 * @param[in,out] *S points to an instance of the Q7 FIR filter structure. 00045 * @param[in] numTaps Number of filter coefficients in the filter. 00046 * @param[in] *pCoeffs points to the filter coefficients buffer. 00047 * @param[in] *pState points to the state buffer. 00048 * @param[in] blockSize number of samples that are processed per call. 00049 * @return none 00050 * 00051 * <b>Description:</b> 00052 * \par 00053 * <code>pCoeffs</code> points to the array of filter coefficients stored in time reversed order: 00054 * <pre> 00055 * {b[numTaps-1], b[numTaps-2], b[N-2], ..., b[1], b[0]} 00056 * </pre> 00057 * \par 00058 * <code>pState</code> points to the array of state variables. 00059 * <code>pState</code> is of length <code>numTaps+blockSize-1</code> samples, where <code>blockSize</code> is the number of input samples processed by each call to <code>arm_fir_q7()</code>. 00060 */ 00061 00062 void arm_fir_init_q7( 00063 arm_fir_instance_q7 * S, 00064 uint16_t numTaps, 00065 q7_t * pCoeffs, 00066 q7_t * pState, 00067 uint32_t blockSize) 00068 { 00069 00070 /* Assign filter taps */ 00071 S->numTaps = numTaps; 00072 00073 /* Assign coefficient pointer */ 00074 S->pCoeffs = pCoeffs; 00075 00076 /* Clear the state buffer. The size is always (blockSize + numTaps - 1) */ 00077 memset(pState, 0, (numTaps + (blockSize - 1u)) * sizeof(q7_t)); 00078 00079 /* Assign state pointer */ 00080 S->pState = pState; 00081 00082 } 00083 00084 /** 00085 * @} end of FIR group 00086 */
Generated on Tue Jul 12 2022 14:13:53 by
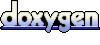