CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_biquad_cascade_df1_init_q15.c
00001 /*----------------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_biquad_cascade_df1_init_q15.c 00009 * 00010 * Description: Q15 Biquad cascade DirectFormI(DF1) filter initialization function. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * ---------------------------------------------------------------------------*/ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupFilters 00037 */ 00038 00039 /** 00040 * @addtogroup BiquadCascadeDF1 00041 * @{ 00042 */ 00043 00044 /** 00045 * @details 00046 * 00047 * @param[in,out] *S points to an instance of the Q15 Biquad cascade structure. 00048 * @param[in] numStages number of 2nd order stages in the filter. 00049 * @param[in] *pCoeffs points to the filter coefficients. 00050 * @param[in] *pState points to the state buffer. 00051 * @param[in] postShift Shift to be applied to the accumulator result. Varies according to the coefficients format 00052 * @return none 00053 * 00054 * <b>Coefficient and State Ordering:</b> 00055 * 00056 * \par 00057 * The coefficients are stored in the array <code>pCoeffs</code> in the following order: 00058 * <pre> 00059 * {b10, 0, b11, b12, a11, a12, b20, 0, b21, b22, a21, a22, ...} 00060 * </pre> 00061 * where <code>b1x</code> and <code>a1x</code> are the coefficients for the first stage, 00062 * <code>b2x</code> and <code>a2x</code> are the coefficients for the second stage, 00063 * and so on. The <code>pCoeffs</code> array contains a total of <code>6*numStages</code> values. 00064 * The zero coefficient between <code>b1</code> and <code>b2</code> facilities use of 16-bit SIMD instructions on the Cortex-M4. 00065 * 00066 * \par 00067 * The state variables are stored in the array <code>pState</code>. 00068 * Each Biquad stage has 4 state variables <code>x[n-1], x[n-2], y[n-1],</code> and <code>y[n-2]</code>. 00069 * The state variables are arranged in the <code>pState</code> array as: 00070 * <pre> 00071 * {x[n-1], x[n-2], y[n-1], y[n-2]} 00072 * </pre> 00073 * The 4 state variables for stage 1 are first, then the 4 state variables for stage 2, and so on. 00074 * The state array has a total length of <code>4*numStages</code> values. 00075 * The state variables are updated after each block of data is processed; the coefficients are untouched. 00076 */ 00077 00078 void arm_biquad_cascade_df1_init_q15( 00079 arm_biquad_casd_df1_inst_q15 * S, 00080 uint8_t numStages, 00081 q15_t * pCoeffs, 00082 q15_t * pState, 00083 int8_t postShift) 00084 { 00085 /* Assign filter stages */ 00086 S->numStages = numStages; 00087 00088 /* Assign postShift to be applied to the output */ 00089 S->postShift = postShift; 00090 00091 /* Assign coefficient pointer */ 00092 S->pCoeffs = pCoeffs; 00093 00094 /* Clear state buffer and size is always 4 * numStages */ 00095 memset(pState, 0, (4u * (uint32_t) numStages) * sizeof(q15_t)); 00096 00097 /* Assign state pointer */ 00098 S->pState = pState; 00099 } 00100 00101 /** 00102 * @} end of BiquadCascadeDF1 group 00103 */
Generated on Tue Jul 12 2022 14:13:52 by
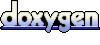