CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
Partial Convolution
[Filtering Functions]
Partial Convolution is equivalent to Convolution except that a subset of the output samples is generated. More...
Functions | |
arm_status | arm_conv_partial_f32 (float32_t *pSrcA, uint32_t srcALen, float32_t *pSrcB, uint32_t srcBLen, float32_t *pDst, uint32_t firstIndex, uint32_t numPoints) |
Partial convolution of floating-point sequences. | |
arm_status | arm_conv_partial_fast_q15 (q15_t *pSrcA, uint32_t srcALen, q15_t *pSrcB, uint32_t srcBLen, q15_t *pDst, uint32_t firstIndex, uint32_t numPoints) |
Partial convolution of Q15 sequences (fast version). | |
arm_status | arm_conv_partial_fast_q31 (q31_t *pSrcA, uint32_t srcALen, q31_t *pSrcB, uint32_t srcBLen, q31_t *pDst, uint32_t firstIndex, uint32_t numPoints) |
Partial convolution of Q31 sequences (fast version). | |
arm_status | arm_conv_partial_q15 (q15_t *pSrcA, uint32_t srcALen, q15_t *pSrcB, uint32_t srcBLen, q15_t *pDst, uint32_t firstIndex, uint32_t numPoints) |
Partial convolution of Q15 sequences. | |
arm_status | arm_conv_partial_q31 (q31_t *pSrcA, uint32_t srcALen, q31_t *pSrcB, uint32_t srcBLen, q31_t *pDst, uint32_t firstIndex, uint32_t numPoints) |
Partial convolution of Q31 sequences. | |
arm_status | arm_conv_partial_q7 (q7_t *pSrcA, uint32_t srcALen, q7_t *pSrcB, uint32_t srcBLen, q7_t *pDst, uint32_t firstIndex, uint32_t numPoints) |
Partial convolution of Q7 sequences. |
Detailed Description
Partial Convolution is equivalent to Convolution except that a subset of the output samples is generated.
Each function has two additional arguments. firstIndex
specifies the starting index of the subset of output samples. numPoints
is the number of output samples to compute. The function computes the output in the range [firstIndex, ..., firstIndex+numPoints-1]
. The output array pDst
contains numPoints
values.
The allowable range of output indices is [0 srcALen+srcBLen-2]. If the requested subset does not fall in this range then the functions return ARM_MATH_ARGUMENT_ERROR. Otherwise the functions return ARM_MATH_SUCCESS.
- Note:
- Refer arm_conv_f32() for details on fixed point behavior.
Function Documentation
arm_status arm_conv_partial_f32 | ( | float32_t * | pSrcA, |
uint32_t | srcALen, | ||
float32_t * | pSrcB, | ||
uint32_t | srcBLen, | ||
float32_t * | pDst, | ||
uint32_t | firstIndex, | ||
uint32_t | numPoints | ||
) |
Partial convolution of floating-point sequences.
- Parameters:
-
[in] *pSrcA points to the first input sequence. [in] srcALen length of the first input sequence. [in] *pSrcB points to the second input sequence. [in] srcBLen length of the second input sequence. [out] *pDst points to the location where the output result is written. [in] firstIndex is the first output sample to start with. [in] numPoints is the number of output points to be computed.
- Returns:
- Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2].
Definition at line 71 of file arm_conv_partial_f32.c.
arm_status arm_conv_partial_fast_q15 | ( | q15_t * | pSrcA, |
uint32_t | srcALen, | ||
q15_t * | pSrcB, | ||
uint32_t | srcBLen, | ||
q15_t * | pDst, | ||
uint32_t | firstIndex, | ||
uint32_t | numPoints | ||
) |
Partial convolution of Q15 sequences (fast version).
- Parameters:
-
[in] *pSrcA points to the first input sequence. [in] srcALen length of the first input sequence. [in] *pSrcB points to the second input sequence. [in] srcBLen length of the second input sequence. [out] *pDst points to the location where the output result is written. [in] firstIndex is the first output sample to start with. [in] numPoints is the number of output points to be computed.
- Returns:
- Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2].
See arm_conv_partial_q15()
for a slower implementation of this function which uses a 64-bit accumulator to avoid wrap around distortion.
Definition at line 53 of file arm_conv_partial_fast_q15.c.
arm_status arm_conv_partial_fast_q31 | ( | q31_t * | pSrcA, |
uint32_t | srcALen, | ||
q31_t * | pSrcB, | ||
uint32_t | srcBLen, | ||
q31_t * | pDst, | ||
uint32_t | firstIndex, | ||
uint32_t | numPoints | ||
) |
Partial convolution of Q31 sequences (fast version).
- Parameters:
-
[in] *pSrcA points to the first input sequence. [in] srcALen length of the first input sequence. [in] *pSrcB points to the second input sequence. [in] srcBLen length of the second input sequence. [out] *pDst points to the location where the output result is written. [in] firstIndex is the first output sample to start with. [in] numPoints is the number of output points to be computed.
- Returns:
- Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2].
- See
arm_conv_partial_q31()
for a slower implementation of this function which uses a 64-bit accumulator to provide higher precision.
Definition at line 53 of file arm_conv_partial_fast_q31.c.
arm_status arm_conv_partial_q15 | ( | q15_t * | pSrcA, |
uint32_t | srcALen, | ||
q15_t * | pSrcB, | ||
uint32_t | srcBLen, | ||
q15_t * | pDst, | ||
uint32_t | firstIndex, | ||
uint32_t | numPoints | ||
) |
Partial convolution of Q15 sequences.
- Parameters:
-
[in] *pSrcA points to the first input sequence. [in] srcALen length of the first input sequence. [in] *pSrcB points to the second input sequence. [in] srcBLen length of the second input sequence. [out] *pDst points to the location where the output result is written. [in] firstIndex is the first output sample to start with. [in] numPoints is the number of output points to be computed.
- Returns:
- Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2].
Refer to arm_conv_partial_fast_q15()
for a faster but less precise version of this function.
Definition at line 57 of file arm_conv_partial_q15.c.
arm_status arm_conv_partial_q31 | ( | q31_t * | pSrcA, |
uint32_t | srcALen, | ||
q31_t * | pSrcB, | ||
uint32_t | srcBLen, | ||
q31_t * | pDst, | ||
uint32_t | firstIndex, | ||
uint32_t | numPoints | ||
) |
Partial convolution of Q31 sequences.
- Parameters:
-
[in] *pSrcA points to the first input sequence. [in] srcALen length of the first input sequence. [in] *pSrcB points to the second input sequence. [in] srcBLen length of the second input sequence. [out] *pDst points to the location where the output result is written. [in] firstIndex is the first output sample to start with. [in] numPoints is the number of output points to be computed.
- Returns:
- Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2].
See arm_conv_partial_fast_q31()
for a faster but less precise implementation of this function.
Definition at line 56 of file arm_conv_partial_q31.c.
arm_status arm_conv_partial_q7 | ( | q7_t * | pSrcA, |
uint32_t | srcALen, | ||
q7_t * | pSrcB, | ||
uint32_t | srcBLen, | ||
q7_t * | pDst, | ||
uint32_t | firstIndex, | ||
uint32_t | numPoints | ||
) |
Partial convolution of Q7 sequences.
- Parameters:
-
[in] *pSrcA points to the first input sequence. [in] srcALen length of the first input sequence. [in] *pSrcB points to the second input sequence. [in] srcBLen length of the second input sequence. [out] *pDst points to the location where the output result is written. [in] firstIndex is the first output sample to start with. [in] numPoints is the number of output points to be computed.
- Returns:
- Returns either ARM_MATH_SUCCESS if the function completed correctly or ARM_MATH_ARGUMENT_ERROR if the requested subset is not in the range [0 srcALen+srcBLen-2].
Definition at line 55 of file arm_conv_partial_q7.c.
Generated on Tue Jul 12 2022 14:13:55 by
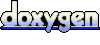