
Example of a simple website hits/signup traffic monitor to drive a display (e.g. servo or panel meter) and indicator (e.g. bell, solenoid) based on responses from a webserver
Dependencies: EthernetNetIf mbed Servo
WebTrafficMonitor.cpp
00001 // Example of a simple website hits/signup traffic monitor to drive 00002 // a display (e.g. servo or panel meter) and indicator (e.g. bell, solenoid) 00003 // based on responses from a webserver 00004 // http://mbed.org 00005 00006 #include "mbed.h" 00007 #include "EthernetNetIf.h" 00008 #include "HTTPClient.h" 00009 #include "Servo.h" 00010 00011 #define SIGNUPS_URL "http://url-returning-signups-int" // number of signups since last checked 00012 #define HITS_URL "http://url-returning-hits-int" // number of hits/sec since last checked 00013 #define HITS_MAX 1000 // scale max hits/sec 00014 00015 EthernetNetIf eth; 00016 HTTPClient http; 00017 00018 DigitalOut bell(p12); 00019 Servo meter(p21); 00020 00021 // ding a bell (fire a digital out driving a solenoid) 00022 void ding() { 00023 bell = 1; 00024 wait(0.25); 00025 bell = 0; 00026 } 00027 00028 // fetch a integer from a url 00029 int get_result(char *url) { 00030 HTTPText txt; 00031 HTTPResult r = http.get(url, &txt); 00032 00033 if(r != HTTP_OK) { 00034 printf("Error [%d] fetching url [%s]\n", r, url); 00035 return -1; 00036 } 00037 00038 printf("Response [%s] from url [%s]\n", txt.gets(), url); 00039 int v = 0; 00040 if(sscanf(txt.gets(), "%d", &v) != 1) { 00041 printf("Could not convert value to int\n"); 00042 } 00043 00044 return v; 00045 } 00046 00047 int main() { 00048 printf("Setup the network...\n"); 00049 EthernetErr ethErr = eth.setup(); 00050 if (ethErr) { 00051 error("Error %d in setup\n", ethErr); 00052 } 00053 printf("OK!\n"); 00054 00055 while (1) { 00056 int hits = get_result(HITS_URL); 00057 int signups = get_result(SIGNUPS_URL); 00058 00059 printf("hits = %d, signups = %d\n", hits, signups); 00060 00061 // ding for every signup since last request 00062 for(int i=0; i<signups; i++) { 00063 ding(); 00064 wait(0.5); 00065 } 00066 00067 // set the servo based on hits/sec 00068 meter = (float)hits / (float)HITS_MAX; 00069 } 00070 }
Generated on Tue Jul 12 2022 19:20:43 by
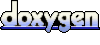