
Example to show how priority can be used to ensure timing critical task can be run
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Example running the mbed Tickers at a lower priority 00002 00003 #include "mbed.h" 00004 00005 volatile int counter = 0; 00006 void timing_critical() { 00007 counter++; 00008 } 00009 00010 void long_event() { 00011 wait_ms(50); 00012 } 00013 00014 PwmOut out(p25); 00015 InterruptIn in(p26); 00016 Ticker tick; 00017 00018 int main() { 00019 out.period_ms(10); 00020 out.pulsewidth_ms(5); 00021 in.rise(&timing_critical); 00022 00023 printf("1) InterruptIn only...\n"); 00024 for(int i=0; i<5; i++) { 00025 counter = 0; 00026 wait(1); 00027 printf("counts/sec = %d\n", counter); 00028 } 00029 00030 tick.attach(&long_event, 0.1); 00031 00032 printf("2) InterruptIn plus long running occasional ticker event...\n"); 00033 for(int i=0; i<5; i++) { 00034 counter = 0; 00035 wait(1); 00036 printf("count/sec = %d\n", counter); 00037 } 00038 00039 printf("3) InterruptIn plus long running occasional ticker event at lower priority...\n"); 00040 NVIC_SetPriority(TIMER3_IRQn, 255); // set mbed tickers to lower priority than other things 00041 for(int i=0; i<5; i++) { 00042 counter = 0; 00043 wait(1); 00044 printf("counter = %d\n", counter); 00045 } 00046 }
Generated on Tue Jul 12 2022 13:33:55 by
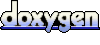