Version of http://mbed.org/cookbook/NetServicesTribute with setting set the same for LPC2368
Dependents: UDPSocketExample 24LCxx_I2CApp WeatherPlatform_pachube HvZServerLib ... more
RPCHandler.cpp
00001 #pragma diag_remark 1293 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "RPCHandler.h" 00025 #include "rpc.h" 00026 #include "url.h" 00027 00028 //#define __DEBUG 00029 #include "dbg/dbg.h" 00030 00031 #define RPC_DATA_LEN 128 00032 00033 RPCHandler::RPCHandler(const char* rootPath, const char* path, TCPSocket* pTCPSocket) : HTTPRequestHandler(rootPath, path, pTCPSocket) 00034 {} 00035 00036 RPCHandler::~RPCHandler() 00037 { 00038 DBG("\r\nHandler destroyed\r\n"); 00039 } 00040 00041 void RPCHandler::doGet() 00042 { 00043 DBG("\r\nIn RPCHandler::doGet()\r\n"); 00044 char resp[RPC_DATA_LEN] = {0}; 00045 char req[RPC_DATA_LEN] = {0}; 00046 00047 DBG("\r\nPath : %s\r\n", path().c_str()); 00048 DBG("\r\nRoot Path : %s\r\n", rootPath().c_str()); 00049 00050 //Remove path 00051 strncpy(req, path().c_str(), RPC_DATA_LEN-1); 00052 DBG("\r\nRPC req : %s\r\n", req); 00053 00054 //Remove %20, +, from req 00055 cleanReq(req); 00056 DBG("\r\nRPC req : %s\r\n", req); 00057 00058 //Do RPC Call 00059 mbed::rpc(req, resp); //FIXME: Use bool result 00060 00061 //Response 00062 setContentLen( strlen(resp) ); 00063 00064 //Make sure that the browser won't cache this request 00065 respHeaders()["Cache-control"]="no-cache;no-store"; 00066 // respHeaders()["Cache-control"]="no-store"; 00067 respHeaders()["Pragma"]="no-cache"; 00068 respHeaders()["Expires"]="0"; 00069 00070 //Write data 00071 respHeaders()["Connection"] = "close"; 00072 writeData(resp, strlen(resp)); 00073 DBG("\r\nExit RPCHandler::doGet()\r\n"); 00074 } 00075 00076 void RPCHandler::doPost() 00077 { 00078 00079 } 00080 00081 void RPCHandler::doHead() 00082 { 00083 00084 } 00085 00086 00087 void RPCHandler::onReadable() //Data has been read 00088 { 00089 00090 } 00091 00092 void RPCHandler::onWriteable() //Data has been written & buf is free 00093 { 00094 DBG("\r\nRPCHandler::onWriteable() event\r\n"); 00095 close(); //Data written, we can close the connection 00096 } 00097 00098 void RPCHandler::onClose() //Connection is closing 00099 { 00100 //Nothing to do 00101 } 00102 00103 void RPCHandler::cleanReq(char* data) 00104 { 00105 char* decoded = url_decode(data); 00106 strcpy(data, decoded); 00107 free(decoded); 00108 /* 00109 char* p; 00110 static const char* lGarbage[2] = {"%20", "+"}; 00111 for(int i = 0; i < 2; i++) 00112 { 00113 while( p = strstr(data, lGarbage[i]) ) 00114 { 00115 memset((void*) p, ' ', strlen(lGarbage[i])); 00116 } 00117 } 00118 */ 00119 } 00120 00121
Generated on Tue Jul 12 2022 14:29:26 by
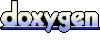