
Simple PID example for LabVIEW
Embed:
(wiki syntax)
Show/hide line numbers
Motor.h
00001 /* mbed simple H-bridge motor controller 00002 * Copyright (c) 2007-2010, sford 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef MBED_MOTOR_H 00024 #define MBED_MOTOR_H 00025 00026 #include "mbed.h" 00027 00028 /** Interface to control a standard DC motor 00029 * with an H-bridge using a PwmOut and 2 DigitalOuts 00030 */ 00031 class Motor { 00032 public: 00033 00034 /** Create a motor control interface 00035 * 00036 * @param pwm A PwmOut pin, driving the H-bridge enable line to control the speed 00037 * @param fwd A DigitalOut, set high when the motor should go forward 00038 * @param rev A DigitalOut, set high when the motor should go backwards 00039 */ 00040 Motor(PinName pwm, PinName fwd, PinName rev); 00041 00042 /** Set the speed of the motor 00043 * 00044 * @param speed The speed of the motor as a normalised value between -1.0 and 1.0 00045 */ 00046 void speed(float speed); 00047 00048 protected: 00049 PwmOut _pwm; 00050 DigitalOut _fwd; 00051 DigitalOut _rev; 00052 00053 }; 00054 00055 #endif
Generated on Tue Jul 12 2022 11:13:38 by
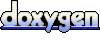