Temp and Humidity sensor device driver.
Dependents: temp_hum IAC_send Smart_Home_sensors
Fork of DHT by
DHT.h
00001 /* 00002 * DHT Library for Digital-output Humidity and Temperature sensors 00003 * 00004 * Works with DHT11, DHT21, DHT22 00005 * SEN11301P, Grove - Temperature&Humidity Sensor (Seeed Studio) 00006 * SEN51035P, Grove - Temperature&Humidity Sensor Pro (Seeed Studio) 00007 * AM2302 , temperature-humidity sensor 00008 * RHT01,RHT02, RHT03 , Humidity and Temperature Sensor (Sparkfun) 00009 * 00010 * Copyright (C) Wim De Roeve 00011 * based on DHT22 sensor library by HO WING KIT 00012 * Arduino DHT11 library 00013 * 00014 * Permission is hereby granted, free of charge, to any person obtaining a copy 00015 * of this software and associated documnetation files (the "Software"), to deal 00016 * in the Software without restriction, including without limitation the rights 00017 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00018 * copies of the Software, and to permit persons to whom the Software is 00019 * furished to do so, subject to the following conditions: 00020 * 00021 * The above copyright notice and this permission notice shall be included in 00022 * all copies or substantial portions of the Software. 00023 * 00024 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00025 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00026 * FITNESS OR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00027 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00028 * LIABILITY WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00029 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00030 * THE SOFTWARE. 00031 */ 00032 00033 #ifndef MBED_DHT_H 00034 #define MBED_DHT_H 00035 00036 #include "mbed.h" 00037 00038 typedef enum eType eType; 00039 enum eType { 00040 DHT11 = 11, 00041 SEN11301P = 11, 00042 RHT01 = 11, 00043 DHT22 = 22, 00044 AM2302 = 22, 00045 SEN51035P = 22, 00046 RHT02 = 22, 00047 RHT03 = 22 00048 }; 00049 00050 typedef enum eError eError; 00051 enum eError { 00052 ERROR_NONE = 0, 00053 BUS_BUSY, 00054 ERROR_NOT_PRESENT, 00055 ERROR_ACK_TOO_LONG, 00056 ERROR_SYNC_TIMEOUT, 00057 ERROR_DATA_TIMEOUT, 00058 ERROR_CHECKSUM, 00059 ERROR_NO_PATIENCE 00060 }; 00061 00062 typedef enum eScale eScale; 00063 enum eScale { 00064 CELCIUS = 0, 00065 FARENHEIT, 00066 KELVIN 00067 }; 00068 00069 00070 class DHT 00071 { 00072 00073 public: 00074 00075 DHT(PinName pin, eType DHTtype); 00076 ~DHT(); 00077 eError readData(void); 00078 float ReadHumidity(void); 00079 float ReadTemperature(eScale const Scale); 00080 float CalcdewPoint(float const celsius, float const humidity); 00081 float CalcdewPointFast(float const celsius, float const humidity); 00082 00083 private: 00084 time_t _lastReadTime; 00085 float _lastTemperature; 00086 float _lastHumidity; 00087 PinName _pin; 00088 bool _firsttime; 00089 eType _DHTtype; 00090 uint8_t DHT_data[5]; 00091 float CalcTemperature(); 00092 float CalcHumidity(); 00093 float ConvertCelciustoFarenheit(float const); 00094 float ConvertCelciustoKelvin(float const); 00095 eError stall(DigitalInOut &io, int const level, int const max_time); 00096 00097 }; 00098 00099 #endif
Generated on Wed Jul 13 2022 09:36:27 by
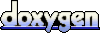