
トランジスタ技術2011年9月号「mbed30分クッキング」のプログラムです。
Dependencies: mbed TextLCD SDFileSystem
ports.c
00001 /*******************************************************/ 00002 /* file: ports.c */ 00003 /* abstract: This file contains the routines to */ 00004 /* output values on the JTAG ports, to read */ 00005 /* the TDO bit, and to read a byte of data */ 00006 /* from the prom */ 00007 /* Revisions: */ 00008 /* 12/01/2008: Same code as before (original v5.01). */ 00009 /* Updated comments to clarify instructions.*/ 00010 /* Add print in setPort for xapp058_example.exe.*/ 00011 /*******************************************************/ 00012 #include "ports.h" 00013 #include "mbed.h" 00014 00015 typedef struct work { 00016 FILE *fp; 00017 int done; 00018 int total; 00019 void (*cbfunc_progress)(int done, int total); 00020 void (*cbfunc_waittime)(int microsec); 00021 } work_t; 00022 00023 work_t work; 00024 DigitalOut g_iTCK(p15); /* For mbed */ 00025 DigitalOut g_iTMS(p16); /* For mbed */ 00026 DigitalOut g_iTDI(p17); /* For mbed */ 00027 DigitalIn g_iTDO(p18); /* For mbed */ 00028 00029 void initPort(FILE *fp, void (*cbfunc_progress)(int done, int total), void (*cbfunc_waittime)(int microsec)) { 00030 work.fp = fp; 00031 fseek(fp, 0, SEEK_END); 00032 work.total = ftell(fp); 00033 work.done = 0; 00034 fseek(fp, 0, SEEK_SET); 00035 work.cbfunc_progress = cbfunc_progress; 00036 work.cbfunc_waittime = cbfunc_waittime; 00037 } 00038 00039 /* setPort: Implement to set the named JTAG signal (p) to the new value (v).*/ 00040 /* if in debugging mode, then just set the variables */ 00041 void setPort(short p,short val) { 00042 /* 00043 * Modified for mbed. 00044 */ 00045 /* Printing code for the xapp058_example.exe. You must set the specified 00046 JTAG signal (p) to the new value (v). See the above, old Win95 code 00047 as an implementation example. */ 00048 if (p == TCK) { 00049 g_iTCK = val; 00050 // printf( "TCK = %d; TMS = %d; TDI = %d\n", g_iTCK.read(), g_iTMS.read(), g_iTDI.read() ); 00051 return; 00052 } 00053 if (p == TMS) { 00054 g_iTMS = val; 00055 return; 00056 } 00057 if (p == TDI) { 00058 g_iTDI = val; 00059 return; 00060 } 00061 } 00062 00063 /* toggle tck LH. No need to modify this code. It is output via setPort. */ 00064 void pulseClock() { 00065 setPort(TCK,0); /* set the TCK port to low */ 00066 setPort(TCK,1); /* set the TCK port to high */ 00067 } 00068 00069 /* readByte: Implement to source the next byte from your XSVF file location */ 00070 /* read in a byte of data from the prom */ 00071 void readByte(unsigned char *data) { 00072 /* 00073 * Modified for mbed. 00074 */ 00075 *data = (unsigned char)fgetc( work.fp ); 00076 00077 work.done++; 00078 if (work.cbfunc_progress != NULL) { 00079 work.cbfunc_progress(work.done, work.total); 00080 } 00081 } 00082 00083 /* readTDOBit: Implement to return the current value of the JTAG TDO signal.*/ 00084 /* read the TDO bit from port */ 00085 unsigned char readTDOBit() { 00086 /* 00087 * Modified for mbed. 00088 */ 00089 return( (unsigned char) g_iTDO ); 00090 } 00091 00092 /* waitTime: Implement as follows: */ 00093 /* REQUIRED: This function must consume/wait at least the specified number */ 00094 /* of microsec, interpreting microsec as a number of microseconds.*/ 00095 /* REQUIRED FOR SPARTAN/VIRTEX FPGAs and indirect flash programming: */ 00096 /* This function must pulse TCK for at least microsec times, */ 00097 /* interpreting microsec as an integer value. */ 00098 /* RECOMMENDED IMPLEMENTATION: Pulse TCK at least microsec times AND */ 00099 /* continue pulsing TCK until the microsec wait */ 00100 /* requirement is also satisfied. */ 00101 void waitTime(long microsec) { 00102 /* 00103 * Modified for mbed. 00104 */ 00105 00106 static long tckCyclesPerMicrosec = 2; /* must be at least 1 */ 00107 long tckCycles = microsec * tckCyclesPerMicrosec; 00108 long i; 00109 00110 if (work.cbfunc_waittime != NULL) { 00111 work.cbfunc_waittime(microsec); 00112 } 00113 00114 /* This implementation is highly recommended!!! */ 00115 /* This implementation requires you to tune the tckCyclesPerMicrosec 00116 variable (above) to match the performance of your embedded system 00117 in order to satisfy the microsec wait time requirement. */ 00118 for ( i = 0; i < tckCycles; ++i ) { 00119 pulseClock(); 00120 } 00121 }
Generated on Tue Jul 12 2022 20:42:46 by
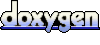