
トランジスタ技術2011年9月号「mbed30分クッキング」のプログラムです。
Dependencies: mbed TextLCD SDFileSystem
main.cpp
00001 00002 #include "mbed.h" 00003 #include "micro.h" 00004 #include "TextLCD.h" 00005 #include "SDFileSystem.h" 00006 00007 TextLCD lcd(p24, p26, p27, p28, p29, p30); 00008 SDFileSystem fs_sd(p5, p6, p7, p8, "sd"); 00009 char *filename = "/sd/target.xsv"; 00010 00011 /** 00012 * Callback function. 00013 * 00014 * @param done Done. 00015 * @param total Total. 00016 */ 00017 void cbfunc_progress(int done, int total) { 00018 if (total != 0) { 00019 static int n = 0; 00020 int tmp = done * 100 / total; 00021 if (tmp != n) { 00022 n = tmp; 00023 lcd.locate(0, 1); 00024 lcd.printf("Progress: %3d%%", n); 00025 } 00026 } 00027 } 00028 00029 /** 00030 * Callback function. 00031 * 00032 * @param microsec Micro seconds. 00033 */ 00034 void cbfunc_waittime(int microsec) { 00035 int n = microsec / 1000 / 1000; 00036 if (n > 0) { 00037 lcd.locate(0, 1); 00038 lcd.printf("Wait %d sec.", n); 00039 } 00040 } 00041 00042 /** 00043 * Entry point. 00044 */ 00045 int main() { 00046 lcd.cls(); 00047 lcd.locate(0, 0); 00048 lcd.printf("mbed XSVF Player"); 00049 wait(2); 00050 00051 lcd.cls(); 00052 lcd.locate(0, 0); 00053 lcd.printf("mbed XSVF Player"); 00054 int r = execute_micro(filename, &cbfunc_progress, &cbfunc_waittime); 00055 lcd.locate(0, 1); 00056 lcd.printf("Done with code %d", r); 00057 00058 return 0; 00059 }
Generated on Tue Jul 12 2022 20:42:46 by
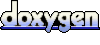