
トランジスタ技術2011年9月号「mbed30分クッキング」のプログラムです。
Dependencies: mbed TextLCD SDFileSystem
lenval.h
00001 /*******************************************************/ 00002 /* file: lenval.h */ 00003 /* abstract: This file contains a description of the */ 00004 /* data structure "lenval". */ 00005 /*******************************************************/ 00006 00007 #ifndef lenval_dot_h 00008 #define lenval_dot_h 00009 00010 /* the lenVal structure is a byte oriented type used to store an */ 00011 /* arbitrary length binary value. As an example, the hex value */ 00012 /* 0x0e3d is represented as a lenVal with len=2 (since 2 bytes */ 00013 /* and val[0]=0e and val[1]=3d. val[2-MAX_LEN] are undefined */ 00014 00015 /* maximum length (in bytes) of value to read in */ 00016 /* this needs to be at least 4, and longer than the */ 00017 /* length of the longest SDR instruction. If there is, */ 00018 /* only 1 device in the chain, MAX_LEN must be at least */ 00019 /* ceil(27/8) == 4. For 6 devices in a chain, MAX_LEN */ 00020 /* must be 5, for 14 devices MAX_LEN must be 6, for 20 */ 00021 /* devices MAX_LEN must be 7, etc.. */ 00022 /* You can safely set MAX_LEN to a smaller number if you*/ 00023 /* know how many devices will be in your chain. */ 00024 /* #define MAX_LEN (Actual #define is below this comment block) 00025 This #define defines the maximum length (in bytes) of predefined 00026 buffers in which the XSVF player stores the current shift data. 00027 This length must be greater than the longest shift length (in bytes) 00028 in the XSVF files that will be processed. 7000 is a very conservative 00029 number. The buffers are stored on the stack and if you have limited 00030 stack space, you may decrease the MAX_LEN value. 00031 00032 How to find the "shift length" in bits? 00033 Look at the ASCII version of the XSVF (generated with the -a option 00034 for the SVF2XSVF translator) and search for the XSDRSIZE command 00035 with the biggest parameter. XSDRSIZE is equivalent to the SVF's 00036 SDR length plus the lengths of applicable HDR and TDR commands. 00037 Remember that the MAX_LEN is defined in bytes. Therefore, the 00038 minimum MAX_LEN = ceil( max( XSDRSIZE ) / 8 ); 00039 00040 The following MAX_LEN values have been tested and provide relatively 00041 good margin for the corresponding devices: 00042 00043 DEVICE MAX_LEN Resulting Shift Length Max (in bits) 00044 --------- ------- ---------------------------------------------- 00045 XC9500/XL/XV 32 256 00046 00047 CoolRunner/II 256 2048 - actual max 1 device = 1035 bits 00048 00049 FPGA 128 1024 - svf2xsvf -rlen 1024 00050 00051 XC18V00/XCF00 00052 1100 8800 - no blank check performed (default) 00053 - actual max 1 device = 8192 bits verify 00054 - max 1 device = 4096 bits program-only 00055 00056 XC18V00/XCF00 when using the optional Blank Check operation 00057 2500 20000 - required for blank check 00058 - blank check max 1 device = 16384 bits 00059 */ 00060 // #define MAX_LEN 7000 /* This is the default value. But it's huge for mbed. */ 00061 #define MAX_LEN 2048 /* Modified for mbed. */ 00062 00063 00064 typedef struct var_len_byte 00065 { 00066 short len; /* number of chars in this value */ 00067 unsigned char val[MAX_LEN+1]; /* bytes of data */ 00068 } lenVal; 00069 00070 00071 /* return the long representation of a lenVal */ 00072 extern long value(lenVal *x); 00073 00074 /* set lenVal equal to value */ 00075 extern void initLenVal(lenVal *x, long value); 00076 00077 /* check if expected equals actual (taking the mask into account) */ 00078 extern short EqualLenVal(lenVal *expected, lenVal *actual, lenVal *mask); 00079 00080 /* add val1+val2 and put the result in resVal */ 00081 extern void addVal(lenVal *resVal, lenVal *val1, lenVal *val2); 00082 00083 /* return the (byte, bit) of lv (reading from left to right) */ 00084 extern short RetBit(lenVal *lv, int byte, int bit); 00085 00086 /* set the (byte, bit) of lv equal to val (e.g. SetBit("00000000",byte, 1) 00087 equals "01000000" */ 00088 extern void SetBit(lenVal *lv, int byte, int bit, short val); 00089 00090 /* read from XSVF numBytes bytes of data into x */ 00091 extern void readVal(lenVal *x, short numBytes); 00092 00093 #endif 00094
Generated on Tue Jul 12 2022 20:42:46 by
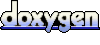