
トランジスタ技術2011年9月号「mbed30分クッキング」のプログラムです。
Dependencies: mbed TextLCD SDFileSystem
lenval.c
00001 /*******************************************************/ 00002 /* file: lenval.c */ 00003 /* abstract: This file contains routines for using */ 00004 /* the lenVal data structure. */ 00005 /*******************************************************/ 00006 #include "lenval.h" 00007 #include "ports.h" 00008 00009 /***************************************************************************** 00010 * Function: value 00011 * Description: Extract the long value from the lenval array. 00012 * Parameters: plvValue - ptr to lenval. 00013 * Returns: long - the extracted value. 00014 *****************************************************************************/ 00015 long value( lenVal* plvValue ) 00016 { 00017 long lValue; /* result to hold the accumulated result */ 00018 short sIndex; 00019 00020 lValue = 0; 00021 for ( sIndex = 0; sIndex < plvValue->len ; ++sIndex ) 00022 { 00023 lValue <<= 8; /* shift the accumulated result */ 00024 lValue |= plvValue->val[ sIndex]; /* get the last byte first */ 00025 } 00026 00027 return( lValue ); 00028 } 00029 00030 /***************************************************************************** 00031 * Function: initLenVal 00032 * Description: Initialize the lenval array with the given value. 00033 * Assumes lValue is less than 256. 00034 * Parameters: plv - ptr to lenval. 00035 * lValue - the value to set. 00036 * Returns: void. 00037 *****************************************************************************/ 00038 void initLenVal( lenVal* plv, 00039 long lValue ) 00040 { 00041 plv->len = 1; 00042 plv->val[0] = (unsigned char)lValue; 00043 } 00044 00045 /***************************************************************************** 00046 * Function: EqualLenVal 00047 * Description: Compare two lenval arrays with an optional mask. 00048 * Parameters: plvTdoExpected - ptr to lenval #1. 00049 * plvTdoCaptured - ptr to lenval #2. 00050 * plvTdoMask - optional ptr to mask (=0 if no mask). 00051 * Returns: short - 0 = mismatch; 1 = equal. 00052 *****************************************************************************/ 00053 short EqualLenVal( lenVal* plvTdoExpected, 00054 lenVal* plvTdoCaptured, 00055 lenVal* plvTdoMask ) 00056 { 00057 short sEqual; 00058 short sIndex; 00059 unsigned char ucByteVal1; 00060 unsigned char ucByteVal2; 00061 unsigned char ucByteMask; 00062 00063 sEqual = 1; 00064 sIndex = plvTdoExpected->len; 00065 00066 while ( sEqual && sIndex-- ) 00067 { 00068 ucByteVal1 = plvTdoExpected->val[ sIndex ]; 00069 ucByteVal2 = plvTdoCaptured->val[ sIndex ]; 00070 if ( plvTdoMask ) 00071 { 00072 ucByteMask = plvTdoMask->val[ sIndex ]; 00073 ucByteVal1 &= ucByteMask; 00074 ucByteVal2 &= ucByteMask; 00075 } 00076 if ( ucByteVal1 != ucByteVal2 ) 00077 { 00078 sEqual = 0; 00079 } 00080 } 00081 00082 return( sEqual ); 00083 } 00084 00085 00086 /***************************************************************************** 00087 * Function: RetBit 00088 * Description: return the (byte, bit) of lv (reading from left to right). 00089 * Parameters: plv - ptr to lenval. 00090 * iByte - the byte to get the bit from. 00091 * iBit - the bit number (0=msb) 00092 * Returns: short - the bit value. 00093 *****************************************************************************/ 00094 short RetBit( lenVal* plv, 00095 int iByte, 00096 int iBit ) 00097 { 00098 /* assert( ( iByte >= 0 ) && ( iByte < plv->len ) ); */ 00099 /* assert( ( iBit >= 0 ) && ( iBit < 8 ) ); */ 00100 return( (short)( ( plv->val[ iByte ] >> ( 7 - iBit ) ) & 0x1 ) ); 00101 } 00102 00103 /***************************************************************************** 00104 * Function: SetBit 00105 * Description: set the (byte, bit) of lv equal to val 00106 * Example: SetBit("00000000",byte, 1) equals "01000000". 00107 * Parameters: plv - ptr to lenval. 00108 * iByte - the byte to get the bit from. 00109 * iBit - the bit number (0=msb). 00110 * sVal - the bit value to set. 00111 * Returns: void. 00112 *****************************************************************************/ 00113 void SetBit( lenVal* plv, 00114 int iByte, 00115 int iBit, 00116 short sVal ) 00117 { 00118 unsigned char ucByteVal; 00119 unsigned char ucBitMask; 00120 00121 ucBitMask = (unsigned char)(1 << ( 7 - iBit )); 00122 ucByteVal = (unsigned char)(plv->val[ iByte ] & (~ucBitMask)); 00123 00124 if ( sVal ) 00125 { 00126 ucByteVal |= ucBitMask; 00127 } 00128 plv->val[ iByte ] = ucByteVal; 00129 } 00130 00131 /***************************************************************************** 00132 * Function: AddVal 00133 * Description: add val1 to val2 and store in resVal; 00134 * assumes val1 and val2 are of equal length. 00135 * Parameters: plvResVal - ptr to result. 00136 * plvVal1 - ptr of addendum. 00137 * plvVal2 - ptr of addendum. 00138 * Returns: void. 00139 *****************************************************************************/ 00140 void addVal( lenVal* plvResVal, 00141 lenVal* plvVal1, 00142 lenVal* plvVal2 ) 00143 { 00144 unsigned char ucCarry; 00145 unsigned short usSum; 00146 unsigned short usVal1; 00147 unsigned short usVal2; 00148 short sIndex; 00149 00150 plvResVal->len = plvVal1->len; /* set up length of result */ 00151 00152 /* start at least significant bit and add bytes */ 00153 ucCarry = 0; 00154 sIndex = plvVal1->len; 00155 while ( sIndex-- ) 00156 { 00157 usVal1 = plvVal1->val[ sIndex ]; /* i'th byte of val1 */ 00158 usVal2 = plvVal2->val[ sIndex ]; /* i'th byte of val2 */ 00159 00160 /* add the two bytes plus carry from previous addition */ 00161 usSum = (unsigned short)( usVal1 + usVal2 + ucCarry ); 00162 00163 /* set up carry for next byte */ 00164 ucCarry = (unsigned char)( ( usSum > 255 ) ? 1 : 0 ); 00165 00166 /* set the i'th byte of the result */ 00167 plvResVal->val[ sIndex ] = (unsigned char)usSum; 00168 } 00169 } 00170 00171 /***************************************************************************** 00172 * Function: readVal 00173 * Description: read from XSVF numBytes bytes of data into x. 00174 * Parameters: plv - ptr to lenval in which to put the bytes read. 00175 * sNumBytes - the number of bytes to read. 00176 * Returns: void. 00177 *****************************************************************************/ 00178 void readVal( lenVal* plv, 00179 short sNumBytes ) 00180 { 00181 unsigned char* pucVal; 00182 00183 plv->len = sNumBytes; /* set the length of the lenVal */ 00184 for ( pucVal = plv->val; sNumBytes; --sNumBytes, ++pucVal ) 00185 { 00186 /* read a byte of data into the lenVal */ 00187 readByte( pucVal ); 00188 } 00189 } 00190
Generated on Tue Jul 12 2022 20:42:46 by
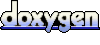