
http://mbed.org/users/shintamainjp/notebook/starboard_expbrd-one_ex1_en/
Dependencies: mbed RemoteIR SuperTweet ConfigFile EthernetNetIf
main.cpp
00001 /** 00002 * ============================================================================= 00003 * Expansion Board One - Example application No.1 (Version 0.0.1) 00004 * http://mbed.org/users/shintamainjp/notebook/starboard_expbrd-one_ex1_en/ 00005 * ============================================================================= 00006 * Copyright (c) 2010 Shinichiro Nakamura (CuBeatSystems) 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * ============================================================================= 00026 */ 00027 00028 #include "mbed.h" 00029 #include "TextLCD.h" 00030 #include "EthernetNetIf.h" 00031 #include "SuperTweetV1XML.h" 00032 #include "ConfigFile.h" 00033 #include "MyHomeLight.h" 00034 #include "StreamFilter.h" 00035 #include "TransmitterIR.h" 00036 #include "appconf.h" 00037 #include <string.h> 00038 00039 extern "C" void mbed_reset(); 00040 00041 /* 00042 * Definitions for a configuration file. 00043 */ 00044 #define CONFIG_FILENAME "/local/SBOE1.CFG" 00045 00046 /* 00047 * Variables. 00048 */ 00049 typedef struct { 00050 bool toggled; 00051 int fetch_count; 00052 int error_count; 00053 int toggle_count; 00054 int text_count; 00055 char txtcurr[1024]; 00056 char txtprev[1024]; 00057 } work_t; 00058 work_t work = { 00059 .toggled = false, 00060 .fetch_count = 0, 00061 .error_count = 0, 00062 .toggle_count = 0, 00063 .text_count = 0, 00064 .txtcurr = {0}, 00065 .txtprev = {0} 00066 }; 00067 static appconf_t appconf; 00068 static const int CHANNEL = 2; 00069 00070 /* 00071 * Classes. 00072 */ 00073 LocalFileSystem localfs("local"); 00074 TextLCD lcd(p24, p26, p27, p28, p29, p30); 00075 EthernetNetIf netif; 00076 MyHomeLight room_light(p21); 00077 StreamFilter sf("<text>", "</text>"); 00078 00079 void splash(void); 00080 int main(void); 00081 00082 /** 00083 * Display a splash screen. 00084 */ 00085 void splash(void) { 00086 lcd.cls(); 00087 lcd.locate(0, 0); 00088 lcd.printf("StarBoard Orange"); 00089 lcd.locate(0, 1); 00090 lcd.printf("Expansion Board "); 00091 wait(2); 00092 00093 lcd.cls(); 00094 lcd.locate(0, 0); 00095 lcd.printf("Example app No.1"); 00096 lcd.locate(0, 1); 00097 lcd.printf(" "); 00098 wait(2); 00099 } 00100 00101 /** 00102 * A callback function with SuperTweet. 00103 * 00104 * @param buf A pointer to a buffer. 00105 * @param siz Size of the buffer. 00106 */ 00107 void cbfunc(char *buf, size_t siz) { 00108 /* 00109 * Push the stream data to the stream filter. 00110 */ 00111 for (int i = 0; i < siz; i++) { 00112 sf.push(buf[i]); 00113 } 00114 00115 /* 00116 * Check the state of the stream filter. 00117 */ 00118 if (sf.done()) { 00119 if (sf.getContent(&work.txtcurr[0], sizeof(work.txtcurr))) { 00120 /* 00121 * Toggle my room light if there is a 'Light' text in twitter stream. 00122 */ 00123 if (strstr(work.txtcurr, "Light") != NULL) { 00124 work.text_count++; 00125 if (work.text_count == 1) { 00126 if (strcmp(work.txtcurr, work.txtprev) != 0) { 00127 if (work.toggle_count > 0) { 00128 if (room_light.toggle(CHANNEL)) { 00129 printf("Controlled.\n"); 00130 work.toggled = true; 00131 } else { 00132 printf("Control failed.\n"); 00133 } 00134 } 00135 work.toggle_count++; 00136 } 00137 strcpy(work.txtprev, work.txtcurr); 00138 } 00139 } 00140 } 00141 sf.reset(); 00142 } 00143 } 00144 00145 /** 00146 * Entry point. 00147 */ 00148 int main(void) { 00149 00150 /* 00151 * Splash. 00152 */ 00153 splash(); 00154 00155 /* 00156 * Initialize ethernet interface. 00157 */ 00158 lcd.cls(); 00159 lcd.locate(0, 0); 00160 lcd.printf("Initializing. "); 00161 lcd.locate(0, 1); 00162 lcd.printf("Ethernet: "); 00163 EthernetErr ethErr = netif.setup(); 00164 if (ethErr) { 00165 lcd.locate(0, 1); 00166 lcd.printf("Ethernet:NG "); 00167 error("Network setup failed.\n"); 00168 } else { 00169 lcd.locate(0, 1); 00170 lcd.printf("Ethernet:OK "); 00171 } 00172 wait(2); 00173 00174 /* 00175 * Read configuration variables from a file. 00176 */ 00177 appconf_init(&appconf); 00178 if (appconf_read(CONFIG_FILENAME, &appconf) != 0) { 00179 lcd.cls(); 00180 lcd.locate(0, 0); 00181 lcd.printf("ConfigFile"); 00182 lcd.locate(0, 1); 00183 lcd.printf("Read error."); 00184 error("Failure to read a configuration file.\n"); 00185 } 00186 00187 /* 00188 * Setup http client object. 00189 * 00190 * Please replace this information by your account. 00191 */ 00192 SuperTweetV1XML twitter(appconf.account, appconf.password); 00193 00194 int count = 0; 00195 while (1) { 00196 /* 00197 * Fetching... 00198 */ 00199 lcd.cls(); 00200 lcd.locate(0, 0); 00201 lcd.printf("Fetching:%d", count++); 00202 lcd.locate(0, 1); 00203 lcd.printf("Toggled :%d", work.toggle_count); 00204 00205 work.fetch_count++; 00206 work.text_count = 0; 00207 work.toggled = false; 00208 sf.reset(); 00209 00210 /* 00211 * getStatusesUserTimeline: Only You can control a device. 00212 * getStatusesHomeTimeline: Everybody can control a device. 00213 */ 00214 // HTTPResult r = twitter.getStatusesUserTimeline(cbfunc); 00215 HTTPResult r = twitter.getStatusesHomeTimeline(cbfunc); 00216 00217 /* 00218 * Report the toggled status to the twitter account. 00219 */ 00220 if (work.toggled) { 00221 work.toggled = false; 00222 char buf[64]; 00223 snprintf(buf, sizeof(buf), "Toggled (%d/%d)", work.toggle_count, count); 00224 if (twitter.postStatusesUpdate(buf) == 0) { 00225 printf("Post done.\n"); 00226 } else { 00227 printf("Post failed.\n"); 00228 } 00229 } 00230 00231 /* 00232 * Note: 00233 * I don't know why sometime it get a protocol error number 5. 00234 * I think it a bug in a mbed library. 00235 */ 00236 printf("No.%d:[%d]\n", work.fetch_count, (int)r); 00237 if ((int)r != 0) { 00238 work.error_count++; 00239 if (work.error_count > 2) { 00240 printf("\tResetting...\n"); 00241 mbed_reset(); 00242 } 00243 } 00244 00245 /* 00246 * Waiting... 00247 */ 00248 lcd.cls(); 00249 lcd.locate(0, 0); 00250 lcd.printf("Waiting..."); 00251 wait(2); 00252 } 00253 }
Generated on Fri Jul 15 2022 13:37:25 by
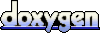