
http://mbed.org/users/shintamainjp/notebook/starboard_expbrd-one_ex1_en/
Dependencies: mbed RemoteIR SuperTweet ConfigFile EthernetNetIf
appconf.cpp
00001 /** 00002 * ============================================================================= 00003 * Application configuration for 'Expansion Board One' example no.1 00004 * http://mbed.org/users/shintamainjp/notebook/starboard_expbrd-one_ex1_en/ 00005 * ============================================================================= 00006 * Copyright (c) 2010 Shinichiro Nakamura (CuBeatSystems) 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * ============================================================================= 00026 */ 00027 00028 #include "appconf.h" 00029 #include "ConfigFile.h" 00030 00031 #define KEY_SUPERTWEET_ACCOUNT "SUPERTWEET_ACCOUNT" 00032 #define KEY_SUPERTWEET_PASSWORD "SUPERTWEET_PASSWORD" 00033 00034 /** 00035 * Initialize configuration. 00036 * 00037 * @param p A pointer to a application config. 00038 */ 00039 void appconf_init(appconf_t *p) { 00040 memset(p->account, 0, sizeof(p->account)); 00041 memset(p->password, 0, sizeof(p->password)); 00042 } 00043 00044 /** 00045 * Read configuration. 00046 * 00047 * @param filename Filename. 00048 * @param p A pointer to a application config. 00049 * @return Return zero if it succeed. 00050 */ 00051 int appconf_read(char *filename, appconf_t *p) { 00052 ConfigFile cf; 00053 if (!cf.read(filename)) { 00054 return -1; 00055 } 00056 if (!cf.getValue(KEY_SUPERTWEET_ACCOUNT, p->account, sizeof(p->account))) { 00057 return -2; 00058 } 00059 if (!cf.getValue(KEY_SUPERTWEET_PASSWORD, p->password, sizeof(p->password))) { 00060 return -3; 00061 } 00062 return 0; 00063 } 00064 00065 /** 00066 * Write configuration. 00067 * 00068 * @param filename Filename. 00069 * @param p A pointer to a application config. 00070 * @return Return zero if it succeed. 00071 */ 00072 int appconf_write(char *filename, appconf_t *p) { 00073 ConfigFile cf; 00074 if (!cf.setValue(KEY_SUPERTWEET_ACCOUNT, p->account)) { 00075 return -1; 00076 } 00077 if (!cf.setValue(KEY_SUPERTWEET_PASSWORD, p->password)) { 00078 return -2; 00079 } 00080 if (!cf.write(filename)) { 00081 return -3; 00082 } 00083 return 0; 00084 }
Generated on Fri Jul 15 2022 13:37:25 by
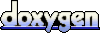