
Code for autonomous rover for Sparkfun AVC. DataBus won 3rd in 2012 and the same code was used on Troubled Child, a 1986 Jeep Grand Wagoneer to win 1st in 2014.
Dependencies: mbed Watchdog SDFileSystem DigoleSerialDisp
print.h
00001 /* 00002 * print.h 00003 * 00004 * Created on: Jan 6, 2014 00005 * Author: mes 00006 */ 00007 00008 #ifndef MYPRINT_H_ 00009 #define MYPRINT_H_ 00010 00011 #include <stdio.h> 00012 #include <stdint.h> 00013 00014 #ifdef __CPLUSPLUS 00015 extern "C" { 00016 #endif 00017 00018 /** print an unsigned long number to FILE 00019 * 00020 * @param f is the FILE * to which the data will be sent 00021 * @param n is the number to print 00022 * @return the number of bytes printed 00023 */ 00024 extern "C" size_t printNumber(FILE *f, unsigned long n); 00025 00026 /** print a signed long number to FILE 00027 * 00028 * @param f is the FILE * to which the data will be sent 00029 * @param n is the number to print 00030 * @return the number of bytes printed 00031 */ 00032 extern "C" size_t printInt(FILE *f, long n); 00033 00034 /** print a double to FILE 00035 * 00036 * @param f is the FILE * to which the data will be sent 00037 * @param number is the number to print 00038 * @param digits is the number of digits to print after the decimal point 00039 * @return the number of bytes printed 00040 */ 00041 extern "C" size_t printFloat(FILE *f, double number, uint8_t digits); 00042 00043 /** print an int as hex to FILE 00044 * 00045 * @param f is the FILE * to which the data will be sent 00046 * @param n is the number to print 00047 * @param digits is the number of hex digits to print 00048 * @return the number of bytes printed 00049 */ 00050 extern "C" size_t printHex(FILE *f, long n, uint8_t digits); 00051 00052 #ifdef __CPLUSPLUS 00053 } 00054 #endif 00055 00056 #endif /* MYPRINT_H_ */
Generated on Tue Jul 12 2022 21:36:19 by
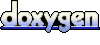