
Code for autonomous rover for Sparkfun AVC. DataBus won 3rd in 2012 and the same code was used on Troubled Child, a 1986 Jeep Grand Wagoneer to win 1st in 2014.
Dependencies: mbed Watchdog SDFileSystem DigoleSerialDisp
Bargraph.cpp
00001 #include "Bargraph.h" 00002 00003 #define CHAR_WIDTH 6 00004 #define WIDTH 8 00005 #define HEIGHT 9 00006 00007 SerialGraphicLCD *Bargraph::lcd = 0; 00008 00009 Bargraph::Bargraph(int x, int y, int size, char name): 00010 _x(x), _y(y), _x2(x+WIDTH), _y2(y+size-1), _s(size), _n(name), _last(0) 00011 { 00012 } 00013 00014 Bargraph::Bargraph(int x, int y, int size, int width, char name): 00015 _x(x), _y(y), _x2(x+width-1), _y2(y+size-1), _s(size), _w(width), _n(name), _last(0) 00016 { 00017 } 00018 00019 void Bargraph::init() 00020 { 00021 if (lcd) { 00022 if (_n != ' ') { 00023 lcd->posXY(_x + (_w/2 - CHAR_WIDTH/2), _y2+2); // horizontal center 00024 //wait_ms(5); 00025 lcd->printf("%c", _n); 00026 //wait_ms(5); 00027 } 00028 lcd->rect(_x, _y, _x2, _y2, true); 00029 //wait_ms(5); // doesn't seem to help 00030 int value = _last; 00031 _last = 0; 00032 update(value); 00033 } 00034 } 00035 00036 void Bargraph::calibrate(float min, float max) 00037 { 00038 _min = min; 00039 _max = max; 00040 } 00041 00042 void Bargraph::update(float value) 00043 { 00044 int ivalue; 00045 00046 ivalue = (int) ((value - _min) * (_s-1)/(_max - _min)); 00047 00048 update(ivalue); 00049 00050 return; 00051 } 00052 00053 void Bargraph::update(int value) 00054 { 00055 if (lcd) { 00056 if (value >= 0 && value < _s) { 00057 int newY = _y2-value; 00058 00059 for (int y=_y+1; y < _y2; y++) { 00060 lcd->line(_x+1, y, _x2-1, y, (y > newY)); 00061 wait_ms(8); 00062 } 00063 } 00064 _last = value; 00065 } 00066 } 00067
Generated on Tue Jul 12 2022 21:36:18 by
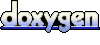