Driver for National Semiconductor ADC128Sxxx family of analog to digital converters
Embed:
(wiki syntax)
Show/hide line numbers
ADC128S.cpp
00001 // ADC128S a library for the National Semiconductor ADC128S family of ADCs 00002 // 00003 // by Michael Shimniok - http://www.bot-thoughts.com/ 00004 // 00005 #include "mbed.h" 00006 #include "ADC128S.h" 00007 00008 ADC128S::ADC128S(PinName mosi, PinName miso, PinName sck, PinName cs) : _adc(mosi, miso, sck), _cs(cs), _channel(0) { 00009 _adc.format(16,3); 00010 _adc.frequency(8000000); 00011 } 00012 00013 int ADC128S::getChannel() { 00014 return _channel; 00015 } 00016 00017 void ADC128S::setChannel(int channel) { 00018 _cs = 0; 00019 _adc.write(channel<<11); // send channel for next acquisition; XXXAAAXX XXXXXXXX 00020 _adc.write(channel<<11); // send channel for next acquisition; XXXAAAXX XXXXXXXX 00021 _cs = 1; 00022 _channel = channel; 00023 } 00024 00025 unsigned int ADC128S::read() { 00026 unsigned int result = 0; 00027 _cs = 0; 00028 // get next acquisition, send next channel: XXXAAAXX XXXXXXXX 00029 _channel++; 00030 _channel %= 8; 00031 result = _adc.write(_channel<<11); 00032 _cs = 1; 00033 00034 return result; 00035 } 00036 00037 unsigned int ADC128S::read(int channel) { 00038 unsigned int result = 0; 00039 _cs = 0; 00040 _adc.write(channel<<11); // send channel for next acquisition; XXXAAAXX XXXXXXXX 00041 _cs = 1; 00042 wait_us(50); 00043 _cs = 0; 00044 result = _adc.write(channel<<11); // get next acquisition 00045 _cs = 1; 00046 00047 return result; 00048 }
Generated on Sat Jul 23 2022 19:31:45 by
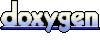