Just changed OUTPUT_SIZE and INPUT_SIZE in ros/node_handle.h
Dependents: WRS2020_mecanum_node
TF2Error.h
00001 #ifndef _ROS_tf2_msgs_TF2Error_h 00002 #define _ROS_tf2_msgs_TF2Error_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 00009 namespace tf2_msgs 00010 { 00011 00012 class TF2Error : public ros::Msg 00013 { 00014 public: 00015 typedef uint8_t _error_type; 00016 _error_type error; 00017 typedef const char* _error_string_type; 00018 _error_string_type error_string; 00019 enum { NO_ERROR = 0 }; 00020 enum { LOOKUP_ERROR = 1 }; 00021 enum { CONNECTIVITY_ERROR = 2 }; 00022 enum { EXTRAPOLATION_ERROR = 3 }; 00023 enum { INVALID_ARGUMENT_ERROR = 4 }; 00024 enum { TIMEOUT_ERROR = 5 }; 00025 enum { TRANSFORM_ERROR = 6 }; 00026 00027 TF2Error(): 00028 error(0), 00029 error_string("") 00030 { 00031 } 00032 00033 virtual int serialize(unsigned char *outbuffer) const 00034 { 00035 int offset = 0; 00036 *(outbuffer + offset + 0) = (this->error >> (8 * 0)) & 0xFF; 00037 offset += sizeof(this->error); 00038 uint32_t length_error_string = strlen(this->error_string); 00039 varToArr(outbuffer + offset, length_error_string); 00040 offset += 4; 00041 memcpy(outbuffer + offset, this->error_string, length_error_string); 00042 offset += length_error_string; 00043 return offset; 00044 } 00045 00046 virtual int deserialize(unsigned char *inbuffer) 00047 { 00048 int offset = 0; 00049 this->error = ((uint8_t) (*(inbuffer + offset))); 00050 offset += sizeof(this->error); 00051 uint32_t length_error_string; 00052 arrToVar(length_error_string, (inbuffer + offset)); 00053 offset += 4; 00054 for(unsigned int k= offset; k< offset+length_error_string; ++k){ 00055 inbuffer[k-1]=inbuffer[k]; 00056 } 00057 inbuffer[offset+length_error_string-1]=0; 00058 this->error_string = (char *)(inbuffer + offset-1); 00059 offset += length_error_string; 00060 return offset; 00061 } 00062 00063 const char * getType(){ return "tf2_msgs/TF2Error"; }; 00064 const char * getMD5(){ return "bc6848fd6fd750c92e38575618a4917d"; }; 00065 00066 }; 00067 00068 } 00069 #endif
Generated on Tue Jul 12 2022 18:49:20 by
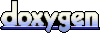