Just changed OUTPUT_SIZE and INPUT_SIZE in ros/node_handle.h
Dependents: WRS2020_mecanum_node
SwitchController.h
00001 #ifndef _ROS_SERVICE_SwitchController_h 00002 #define _ROS_SERVICE_SwitchController_h 00003 #include <stdint.h> 00004 #include <string.h> 00005 #include <stdlib.h> 00006 #include "ros/msg.h" 00007 00008 namespace controller_manager_msgs 00009 { 00010 00011 static const char SWITCHCONTROLLER[] = "controller_manager_msgs/SwitchController"; 00012 00013 class SwitchControllerRequest : public ros::Msg 00014 { 00015 public: 00016 uint32_t start_controllers_length; 00017 typedef char* _start_controllers_type; 00018 _start_controllers_type st_start_controllers; 00019 _start_controllers_type * start_controllers; 00020 uint32_t stop_controllers_length; 00021 typedef char* _stop_controllers_type; 00022 _stop_controllers_type st_stop_controllers; 00023 _stop_controllers_type * stop_controllers; 00024 typedef int32_t _strictness_type; 00025 _strictness_type strictness; 00026 enum { BEST_EFFORT = 1 }; 00027 enum { STRICT = 2 }; 00028 00029 SwitchControllerRequest(): 00030 start_controllers_length(0), start_controllers(NULL), 00031 stop_controllers_length(0), stop_controllers(NULL), 00032 strictness(0) 00033 { 00034 } 00035 00036 virtual int serialize(unsigned char *outbuffer) const 00037 { 00038 int offset = 0; 00039 *(outbuffer + offset + 0) = (this->start_controllers_length >> (8 * 0)) & 0xFF; 00040 *(outbuffer + offset + 1) = (this->start_controllers_length >> (8 * 1)) & 0xFF; 00041 *(outbuffer + offset + 2) = (this->start_controllers_length >> (8 * 2)) & 0xFF; 00042 *(outbuffer + offset + 3) = (this->start_controllers_length >> (8 * 3)) & 0xFF; 00043 offset += sizeof(this->start_controllers_length); 00044 for( uint32_t i = 0; i < start_controllers_length; i++){ 00045 uint32_t length_start_controllersi = strlen(this->start_controllers[i]); 00046 varToArr(outbuffer + offset, length_start_controllersi); 00047 offset += 4; 00048 memcpy(outbuffer + offset, this->start_controllers[i], length_start_controllersi); 00049 offset += length_start_controllersi; 00050 } 00051 *(outbuffer + offset + 0) = (this->stop_controllers_length >> (8 * 0)) & 0xFF; 00052 *(outbuffer + offset + 1) = (this->stop_controllers_length >> (8 * 1)) & 0xFF; 00053 *(outbuffer + offset + 2) = (this->stop_controllers_length >> (8 * 2)) & 0xFF; 00054 *(outbuffer + offset + 3) = (this->stop_controllers_length >> (8 * 3)) & 0xFF; 00055 offset += sizeof(this->stop_controllers_length); 00056 for( uint32_t i = 0; i < stop_controllers_length; i++){ 00057 uint32_t length_stop_controllersi = strlen(this->stop_controllers[i]); 00058 varToArr(outbuffer + offset, length_stop_controllersi); 00059 offset += 4; 00060 memcpy(outbuffer + offset, this->stop_controllers[i], length_stop_controllersi); 00061 offset += length_stop_controllersi; 00062 } 00063 union { 00064 int32_t real; 00065 uint32_t base; 00066 } u_strictness; 00067 u_strictness.real = this->strictness; 00068 *(outbuffer + offset + 0) = (u_strictness.base >> (8 * 0)) & 0xFF; 00069 *(outbuffer + offset + 1) = (u_strictness.base >> (8 * 1)) & 0xFF; 00070 *(outbuffer + offset + 2) = (u_strictness.base >> (8 * 2)) & 0xFF; 00071 *(outbuffer + offset + 3) = (u_strictness.base >> (8 * 3)) & 0xFF; 00072 offset += sizeof(this->strictness); 00073 return offset; 00074 } 00075 00076 virtual int deserialize(unsigned char *inbuffer) 00077 { 00078 int offset = 0; 00079 uint32_t start_controllers_lengthT = ((uint32_t) (*(inbuffer + offset))); 00080 start_controllers_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00081 start_controllers_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00082 start_controllers_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00083 offset += sizeof(this->start_controllers_length); 00084 if(start_controllers_lengthT > start_controllers_length) 00085 this->start_controllers = (char**)realloc(this->start_controllers, start_controllers_lengthT * sizeof(char*)); 00086 start_controllers_length = start_controllers_lengthT; 00087 for( uint32_t i = 0; i < start_controllers_length; i++){ 00088 uint32_t length_st_start_controllers; 00089 arrToVar(length_st_start_controllers, (inbuffer + offset)); 00090 offset += 4; 00091 for(unsigned int k= offset; k< offset+length_st_start_controllers; ++k){ 00092 inbuffer[k-1]=inbuffer[k]; 00093 } 00094 inbuffer[offset+length_st_start_controllers-1]=0; 00095 this->st_start_controllers = (char *)(inbuffer + offset-1); 00096 offset += length_st_start_controllers; 00097 memcpy( &(this->start_controllers[i]), &(this->st_start_controllers), sizeof(char*)); 00098 } 00099 uint32_t stop_controllers_lengthT = ((uint32_t) (*(inbuffer + offset))); 00100 stop_controllers_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00101 stop_controllers_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00102 stop_controllers_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00103 offset += sizeof(this->stop_controllers_length); 00104 if(stop_controllers_lengthT > stop_controllers_length) 00105 this->stop_controllers = (char**)realloc(this->stop_controllers, stop_controllers_lengthT * sizeof(char*)); 00106 stop_controllers_length = stop_controllers_lengthT; 00107 for( uint32_t i = 0; i < stop_controllers_length; i++){ 00108 uint32_t length_st_stop_controllers; 00109 arrToVar(length_st_stop_controllers, (inbuffer + offset)); 00110 offset += 4; 00111 for(unsigned int k= offset; k< offset+length_st_stop_controllers; ++k){ 00112 inbuffer[k-1]=inbuffer[k]; 00113 } 00114 inbuffer[offset+length_st_stop_controllers-1]=0; 00115 this->st_stop_controllers = (char *)(inbuffer + offset-1); 00116 offset += length_st_stop_controllers; 00117 memcpy( &(this->stop_controllers[i]), &(this->st_stop_controllers), sizeof(char*)); 00118 } 00119 union { 00120 int32_t real; 00121 uint32_t base; 00122 } u_strictness; 00123 u_strictness.base = 0; 00124 u_strictness.base |= ((uint32_t) (*(inbuffer + offset + 0))) << (8 * 0); 00125 u_strictness.base |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00126 u_strictness.base |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00127 u_strictness.base |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00128 this->strictness = u_strictness.real; 00129 offset += sizeof(this->strictness); 00130 return offset; 00131 } 00132 00133 const char * getType(){ return SWITCHCONTROLLER; }; 00134 const char * getMD5(){ return "434da54adc434a5af5743ed711fd6ba1"; }; 00135 00136 }; 00137 00138 class SwitchControllerResponse : public ros::Msg 00139 { 00140 public: 00141 typedef bool _ok_type; 00142 _ok_type ok; 00143 00144 SwitchControllerResponse(): 00145 ok(0) 00146 { 00147 } 00148 00149 virtual int serialize(unsigned char *outbuffer) const 00150 { 00151 int offset = 0; 00152 union { 00153 bool real; 00154 uint8_t base; 00155 } u_ok; 00156 u_ok.real = this->ok; 00157 *(outbuffer + offset + 0) = (u_ok.base >> (8 * 0)) & 0xFF; 00158 offset += sizeof(this->ok); 00159 return offset; 00160 } 00161 00162 virtual int deserialize(unsigned char *inbuffer) 00163 { 00164 int offset = 0; 00165 union { 00166 bool real; 00167 uint8_t base; 00168 } u_ok; 00169 u_ok.base = 0; 00170 u_ok.base |= ((uint8_t) (*(inbuffer + offset + 0))) << (8 * 0); 00171 this->ok = u_ok.real; 00172 offset += sizeof(this->ok); 00173 return offset; 00174 } 00175 00176 const char * getType(){ return SWITCHCONTROLLER; }; 00177 const char * getMD5(){ return "6f6da3883749771fac40d6deb24a8c02"; }; 00178 00179 }; 00180 00181 class SwitchController { 00182 public: 00183 typedef SwitchControllerRequest Request; 00184 typedef SwitchControllerResponse Response; 00185 }; 00186 00187 } 00188 #endif
Generated on Tue Jul 12 2022 18:49:20 by
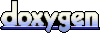