Just changed OUTPUT_SIZE and INPUT_SIZE in ros/node_handle.h
Dependents: WRS2020_mecanum_node
QueryTrajectoryState.h
00001 #ifndef _ROS_SERVICE_QueryTrajectoryState_h 00002 #define _ROS_SERVICE_QueryTrajectoryState_h 00003 #include <stdint.h> 00004 #include <string.h> 00005 #include <stdlib.h> 00006 #include "ros/msg.h" 00007 #include "ros/time.h" 00008 00009 namespace control_msgs 00010 { 00011 00012 static const char QUERYTRAJECTORYSTATE[] = "control_msgs/QueryTrajectoryState"; 00013 00014 class QueryTrajectoryStateRequest : public ros::Msg 00015 { 00016 public: 00017 typedef ros::Time _time_type; 00018 _time_type time; 00019 00020 QueryTrajectoryStateRequest(): 00021 time() 00022 { 00023 } 00024 00025 virtual int serialize(unsigned char *outbuffer) const 00026 { 00027 int offset = 0; 00028 *(outbuffer + offset + 0) = (this->time.sec >> (8 * 0)) & 0xFF; 00029 *(outbuffer + offset + 1) = (this->time.sec >> (8 * 1)) & 0xFF; 00030 *(outbuffer + offset + 2) = (this->time.sec >> (8 * 2)) & 0xFF; 00031 *(outbuffer + offset + 3) = (this->time.sec >> (8 * 3)) & 0xFF; 00032 offset += sizeof(this->time.sec); 00033 *(outbuffer + offset + 0) = (this->time.nsec >> (8 * 0)) & 0xFF; 00034 *(outbuffer + offset + 1) = (this->time.nsec >> (8 * 1)) & 0xFF; 00035 *(outbuffer + offset + 2) = (this->time.nsec >> (8 * 2)) & 0xFF; 00036 *(outbuffer + offset + 3) = (this->time.nsec >> (8 * 3)) & 0xFF; 00037 offset += sizeof(this->time.nsec); 00038 return offset; 00039 } 00040 00041 virtual int deserialize(unsigned char *inbuffer) 00042 { 00043 int offset = 0; 00044 this->time.sec = ((uint32_t) (*(inbuffer + offset))); 00045 this->time.sec |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00046 this->time.sec |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00047 this->time.sec |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00048 offset += sizeof(this->time.sec); 00049 this->time.nsec = ((uint32_t) (*(inbuffer + offset))); 00050 this->time.nsec |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00051 this->time.nsec |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00052 this->time.nsec |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00053 offset += sizeof(this->time.nsec); 00054 return offset; 00055 } 00056 00057 const char * getType(){ return QUERYTRAJECTORYSTATE; }; 00058 const char * getMD5(){ return "556a4fb76023a469987922359d08a844"; }; 00059 00060 }; 00061 00062 class QueryTrajectoryStateResponse : public ros::Msg 00063 { 00064 public: 00065 uint32_t name_length; 00066 typedef char* _name_type; 00067 _name_type st_name; 00068 _name_type * name; 00069 uint32_t position_length; 00070 typedef double _position_type; 00071 _position_type st_position; 00072 _position_type * position; 00073 uint32_t velocity_length; 00074 typedef double _velocity_type; 00075 _velocity_type st_velocity; 00076 _velocity_type * velocity; 00077 uint32_t acceleration_length; 00078 typedef double _acceleration_type; 00079 _acceleration_type st_acceleration; 00080 _acceleration_type * acceleration; 00081 00082 QueryTrajectoryStateResponse(): 00083 name_length(0), name(NULL), 00084 position_length(0), position(NULL), 00085 velocity_length(0), velocity(NULL), 00086 acceleration_length(0), acceleration(NULL) 00087 { 00088 } 00089 00090 virtual int serialize(unsigned char *outbuffer) const 00091 { 00092 int offset = 0; 00093 *(outbuffer + offset + 0) = (this->name_length >> (8 * 0)) & 0xFF; 00094 *(outbuffer + offset + 1) = (this->name_length >> (8 * 1)) & 0xFF; 00095 *(outbuffer + offset + 2) = (this->name_length >> (8 * 2)) & 0xFF; 00096 *(outbuffer + offset + 3) = (this->name_length >> (8 * 3)) & 0xFF; 00097 offset += sizeof(this->name_length); 00098 for( uint32_t i = 0; i < name_length; i++){ 00099 uint32_t length_namei = strlen(this->name[i]); 00100 varToArr(outbuffer + offset, length_namei); 00101 offset += 4; 00102 memcpy(outbuffer + offset, this->name[i], length_namei); 00103 offset += length_namei; 00104 } 00105 *(outbuffer + offset + 0) = (this->position_length >> (8 * 0)) & 0xFF; 00106 *(outbuffer + offset + 1) = (this->position_length >> (8 * 1)) & 0xFF; 00107 *(outbuffer + offset + 2) = (this->position_length >> (8 * 2)) & 0xFF; 00108 *(outbuffer + offset + 3) = (this->position_length >> (8 * 3)) & 0xFF; 00109 offset += sizeof(this->position_length); 00110 for( uint32_t i = 0; i < position_length; i++){ 00111 union { 00112 double real; 00113 uint64_t base; 00114 } u_positioni; 00115 u_positioni.real = this->position[i]; 00116 *(outbuffer + offset + 0) = (u_positioni.base >> (8 * 0)) & 0xFF; 00117 *(outbuffer + offset + 1) = (u_positioni.base >> (8 * 1)) & 0xFF; 00118 *(outbuffer + offset + 2) = (u_positioni.base >> (8 * 2)) & 0xFF; 00119 *(outbuffer + offset + 3) = (u_positioni.base >> (8 * 3)) & 0xFF; 00120 *(outbuffer + offset + 4) = (u_positioni.base >> (8 * 4)) & 0xFF; 00121 *(outbuffer + offset + 5) = (u_positioni.base >> (8 * 5)) & 0xFF; 00122 *(outbuffer + offset + 6) = (u_positioni.base >> (8 * 6)) & 0xFF; 00123 *(outbuffer + offset + 7) = (u_positioni.base >> (8 * 7)) & 0xFF; 00124 offset += sizeof(this->position[i]); 00125 } 00126 *(outbuffer + offset + 0) = (this->velocity_length >> (8 * 0)) & 0xFF; 00127 *(outbuffer + offset + 1) = (this->velocity_length >> (8 * 1)) & 0xFF; 00128 *(outbuffer + offset + 2) = (this->velocity_length >> (8 * 2)) & 0xFF; 00129 *(outbuffer + offset + 3) = (this->velocity_length >> (8 * 3)) & 0xFF; 00130 offset += sizeof(this->velocity_length); 00131 for( uint32_t i = 0; i < velocity_length; i++){ 00132 union { 00133 double real; 00134 uint64_t base; 00135 } u_velocityi; 00136 u_velocityi.real = this->velocity[i]; 00137 *(outbuffer + offset + 0) = (u_velocityi.base >> (8 * 0)) & 0xFF; 00138 *(outbuffer + offset + 1) = (u_velocityi.base >> (8 * 1)) & 0xFF; 00139 *(outbuffer + offset + 2) = (u_velocityi.base >> (8 * 2)) & 0xFF; 00140 *(outbuffer + offset + 3) = (u_velocityi.base >> (8 * 3)) & 0xFF; 00141 *(outbuffer + offset + 4) = (u_velocityi.base >> (8 * 4)) & 0xFF; 00142 *(outbuffer + offset + 5) = (u_velocityi.base >> (8 * 5)) & 0xFF; 00143 *(outbuffer + offset + 6) = (u_velocityi.base >> (8 * 6)) & 0xFF; 00144 *(outbuffer + offset + 7) = (u_velocityi.base >> (8 * 7)) & 0xFF; 00145 offset += sizeof(this->velocity[i]); 00146 } 00147 *(outbuffer + offset + 0) = (this->acceleration_length >> (8 * 0)) & 0xFF; 00148 *(outbuffer + offset + 1) = (this->acceleration_length >> (8 * 1)) & 0xFF; 00149 *(outbuffer + offset + 2) = (this->acceleration_length >> (8 * 2)) & 0xFF; 00150 *(outbuffer + offset + 3) = (this->acceleration_length >> (8 * 3)) & 0xFF; 00151 offset += sizeof(this->acceleration_length); 00152 for( uint32_t i = 0; i < acceleration_length; i++){ 00153 union { 00154 double real; 00155 uint64_t base; 00156 } u_accelerationi; 00157 u_accelerationi.real = this->acceleration[i]; 00158 *(outbuffer + offset + 0) = (u_accelerationi.base >> (8 * 0)) & 0xFF; 00159 *(outbuffer + offset + 1) = (u_accelerationi.base >> (8 * 1)) & 0xFF; 00160 *(outbuffer + offset + 2) = (u_accelerationi.base >> (8 * 2)) & 0xFF; 00161 *(outbuffer + offset + 3) = (u_accelerationi.base >> (8 * 3)) & 0xFF; 00162 *(outbuffer + offset + 4) = (u_accelerationi.base >> (8 * 4)) & 0xFF; 00163 *(outbuffer + offset + 5) = (u_accelerationi.base >> (8 * 5)) & 0xFF; 00164 *(outbuffer + offset + 6) = (u_accelerationi.base >> (8 * 6)) & 0xFF; 00165 *(outbuffer + offset + 7) = (u_accelerationi.base >> (8 * 7)) & 0xFF; 00166 offset += sizeof(this->acceleration[i]); 00167 } 00168 return offset; 00169 } 00170 00171 virtual int deserialize(unsigned char *inbuffer) 00172 { 00173 int offset = 0; 00174 uint32_t name_lengthT = ((uint32_t) (*(inbuffer + offset))); 00175 name_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00176 name_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00177 name_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00178 offset += sizeof(this->name_length); 00179 if(name_lengthT > name_length) 00180 this->name = (char**)realloc(this->name, name_lengthT * sizeof(char*)); 00181 name_length = name_lengthT; 00182 for( uint32_t i = 0; i < name_length; i++){ 00183 uint32_t length_st_name; 00184 arrToVar(length_st_name, (inbuffer + offset)); 00185 offset += 4; 00186 for(unsigned int k= offset; k< offset+length_st_name; ++k){ 00187 inbuffer[k-1]=inbuffer[k]; 00188 } 00189 inbuffer[offset+length_st_name-1]=0; 00190 this->st_name = (char *)(inbuffer + offset-1); 00191 offset += length_st_name; 00192 memcpy( &(this->name[i]), &(this->st_name), sizeof(char*)); 00193 } 00194 uint32_t position_lengthT = ((uint32_t) (*(inbuffer + offset))); 00195 position_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00196 position_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00197 position_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00198 offset += sizeof(this->position_length); 00199 if(position_lengthT > position_length) 00200 this->position = (double*)realloc(this->position, position_lengthT * sizeof(double)); 00201 position_length = position_lengthT; 00202 for( uint32_t i = 0; i < position_length; i++){ 00203 union { 00204 double real; 00205 uint64_t base; 00206 } u_st_position; 00207 u_st_position.base = 0; 00208 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00209 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00210 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00211 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00212 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00213 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00214 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00215 u_st_position.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00216 this->st_position = u_st_position.real; 00217 offset += sizeof(this->st_position); 00218 memcpy( &(this->position[i]), &(this->st_position), sizeof(double)); 00219 } 00220 uint32_t velocity_lengthT = ((uint32_t) (*(inbuffer + offset))); 00221 velocity_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00222 velocity_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00223 velocity_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00224 offset += sizeof(this->velocity_length); 00225 if(velocity_lengthT > velocity_length) 00226 this->velocity = (double*)realloc(this->velocity, velocity_lengthT * sizeof(double)); 00227 velocity_length = velocity_lengthT; 00228 for( uint32_t i = 0; i < velocity_length; i++){ 00229 union { 00230 double real; 00231 uint64_t base; 00232 } u_st_velocity; 00233 u_st_velocity.base = 0; 00234 u_st_velocity.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00235 u_st_velocity.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00236 u_st_velocity.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00237 u_st_velocity.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00238 u_st_velocity.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00239 u_st_velocity.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00240 u_st_velocity.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00241 u_st_velocity.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00242 this->st_velocity = u_st_velocity.real; 00243 offset += sizeof(this->st_velocity); 00244 memcpy( &(this->velocity[i]), &(this->st_velocity), sizeof(double)); 00245 } 00246 uint32_t acceleration_lengthT = ((uint32_t) (*(inbuffer + offset))); 00247 acceleration_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00248 acceleration_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00249 acceleration_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00250 offset += sizeof(this->acceleration_length); 00251 if(acceleration_lengthT > acceleration_length) 00252 this->acceleration = (double*)realloc(this->acceleration, acceleration_lengthT * sizeof(double)); 00253 acceleration_length = acceleration_lengthT; 00254 for( uint32_t i = 0; i < acceleration_length; i++){ 00255 union { 00256 double real; 00257 uint64_t base; 00258 } u_st_acceleration; 00259 u_st_acceleration.base = 0; 00260 u_st_acceleration.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00261 u_st_acceleration.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00262 u_st_acceleration.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00263 u_st_acceleration.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00264 u_st_acceleration.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00265 u_st_acceleration.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00266 u_st_acceleration.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00267 u_st_acceleration.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00268 this->st_acceleration = u_st_acceleration.real; 00269 offset += sizeof(this->st_acceleration); 00270 memcpy( &(this->acceleration[i]), &(this->st_acceleration), sizeof(double)); 00271 } 00272 return offset; 00273 } 00274 00275 const char * getType(){ return QUERYTRAJECTORYSTATE; }; 00276 const char * getMD5(){ return "1f1a6554ad060f44d013e71868403c1a"; }; 00277 00278 }; 00279 00280 class QueryTrajectoryState { 00281 public: 00282 typedef QueryTrajectoryStateRequest Request; 00283 typedef QueryTrajectoryStateResponse Response; 00284 }; 00285 00286 } 00287 #endif
Generated on Tue Jul 12 2022 18:49:19 by
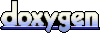