Just changed OUTPUT_SIZE and INPUT_SIZE in ros/node_handle.h
Dependents: WRS2020_mecanum_node
JointControllerState.h
00001 #ifndef _ROS_control_msgs_JointControllerState_h 00002 #define _ROS_control_msgs_JointControllerState_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 #include "std_msgs/Header.h" 00009 00010 namespace control_msgs 00011 { 00012 00013 class JointControllerState : public ros::Msg 00014 { 00015 public: 00016 typedef std_msgs::Header _header_type; 00017 _header_type header; 00018 typedef double _set_point_type; 00019 _set_point_type set_point; 00020 typedef double _process_value_type; 00021 _process_value_type process_value; 00022 typedef double _process_value_dot_type; 00023 _process_value_dot_type process_value_dot; 00024 typedef double _error_type; 00025 _error_type error; 00026 typedef double _time_step_type; 00027 _time_step_type time_step; 00028 typedef double _command_type; 00029 _command_type command; 00030 typedef double _p_type; 00031 _p_type p; 00032 typedef double _i_type; 00033 _i_type i; 00034 typedef double _d_type; 00035 _d_type d; 00036 typedef double _i_clamp_type; 00037 _i_clamp_type i_clamp; 00038 typedef bool _antiwindup_type; 00039 _antiwindup_type antiwindup; 00040 00041 JointControllerState(): 00042 header(), 00043 set_point(0), 00044 process_value(0), 00045 process_value_dot(0), 00046 error(0), 00047 time_step(0), 00048 command(0), 00049 p(0), 00050 i(0), 00051 d(0), 00052 i_clamp(0), 00053 antiwindup(0) 00054 { 00055 } 00056 00057 virtual int serialize(unsigned char *outbuffer) const 00058 { 00059 int offset = 0; 00060 offset += this->header.serialize(outbuffer + offset); 00061 union { 00062 double real; 00063 uint64_t base; 00064 } u_set_point; 00065 u_set_point.real = this->set_point; 00066 *(outbuffer + offset + 0) = (u_set_point.base >> (8 * 0)) & 0xFF; 00067 *(outbuffer + offset + 1) = (u_set_point.base >> (8 * 1)) & 0xFF; 00068 *(outbuffer + offset + 2) = (u_set_point.base >> (8 * 2)) & 0xFF; 00069 *(outbuffer + offset + 3) = (u_set_point.base >> (8 * 3)) & 0xFF; 00070 *(outbuffer + offset + 4) = (u_set_point.base >> (8 * 4)) & 0xFF; 00071 *(outbuffer + offset + 5) = (u_set_point.base >> (8 * 5)) & 0xFF; 00072 *(outbuffer + offset + 6) = (u_set_point.base >> (8 * 6)) & 0xFF; 00073 *(outbuffer + offset + 7) = (u_set_point.base >> (8 * 7)) & 0xFF; 00074 offset += sizeof(this->set_point); 00075 union { 00076 double real; 00077 uint64_t base; 00078 } u_process_value; 00079 u_process_value.real = this->process_value; 00080 *(outbuffer + offset + 0) = (u_process_value.base >> (8 * 0)) & 0xFF; 00081 *(outbuffer + offset + 1) = (u_process_value.base >> (8 * 1)) & 0xFF; 00082 *(outbuffer + offset + 2) = (u_process_value.base >> (8 * 2)) & 0xFF; 00083 *(outbuffer + offset + 3) = (u_process_value.base >> (8 * 3)) & 0xFF; 00084 *(outbuffer + offset + 4) = (u_process_value.base >> (8 * 4)) & 0xFF; 00085 *(outbuffer + offset + 5) = (u_process_value.base >> (8 * 5)) & 0xFF; 00086 *(outbuffer + offset + 6) = (u_process_value.base >> (8 * 6)) & 0xFF; 00087 *(outbuffer + offset + 7) = (u_process_value.base >> (8 * 7)) & 0xFF; 00088 offset += sizeof(this->process_value); 00089 union { 00090 double real; 00091 uint64_t base; 00092 } u_process_value_dot; 00093 u_process_value_dot.real = this->process_value_dot; 00094 *(outbuffer + offset + 0) = (u_process_value_dot.base >> (8 * 0)) & 0xFF; 00095 *(outbuffer + offset + 1) = (u_process_value_dot.base >> (8 * 1)) & 0xFF; 00096 *(outbuffer + offset + 2) = (u_process_value_dot.base >> (8 * 2)) & 0xFF; 00097 *(outbuffer + offset + 3) = (u_process_value_dot.base >> (8 * 3)) & 0xFF; 00098 *(outbuffer + offset + 4) = (u_process_value_dot.base >> (8 * 4)) & 0xFF; 00099 *(outbuffer + offset + 5) = (u_process_value_dot.base >> (8 * 5)) & 0xFF; 00100 *(outbuffer + offset + 6) = (u_process_value_dot.base >> (8 * 6)) & 0xFF; 00101 *(outbuffer + offset + 7) = (u_process_value_dot.base >> (8 * 7)) & 0xFF; 00102 offset += sizeof(this->process_value_dot); 00103 union { 00104 double real; 00105 uint64_t base; 00106 } u_error; 00107 u_error.real = this->error; 00108 *(outbuffer + offset + 0) = (u_error.base >> (8 * 0)) & 0xFF; 00109 *(outbuffer + offset + 1) = (u_error.base >> (8 * 1)) & 0xFF; 00110 *(outbuffer + offset + 2) = (u_error.base >> (8 * 2)) & 0xFF; 00111 *(outbuffer + offset + 3) = (u_error.base >> (8 * 3)) & 0xFF; 00112 *(outbuffer + offset + 4) = (u_error.base >> (8 * 4)) & 0xFF; 00113 *(outbuffer + offset + 5) = (u_error.base >> (8 * 5)) & 0xFF; 00114 *(outbuffer + offset + 6) = (u_error.base >> (8 * 6)) & 0xFF; 00115 *(outbuffer + offset + 7) = (u_error.base >> (8 * 7)) & 0xFF; 00116 offset += sizeof(this->error); 00117 union { 00118 double real; 00119 uint64_t base; 00120 } u_time_step; 00121 u_time_step.real = this->time_step; 00122 *(outbuffer + offset + 0) = (u_time_step.base >> (8 * 0)) & 0xFF; 00123 *(outbuffer + offset + 1) = (u_time_step.base >> (8 * 1)) & 0xFF; 00124 *(outbuffer + offset + 2) = (u_time_step.base >> (8 * 2)) & 0xFF; 00125 *(outbuffer + offset + 3) = (u_time_step.base >> (8 * 3)) & 0xFF; 00126 *(outbuffer + offset + 4) = (u_time_step.base >> (8 * 4)) & 0xFF; 00127 *(outbuffer + offset + 5) = (u_time_step.base >> (8 * 5)) & 0xFF; 00128 *(outbuffer + offset + 6) = (u_time_step.base >> (8 * 6)) & 0xFF; 00129 *(outbuffer + offset + 7) = (u_time_step.base >> (8 * 7)) & 0xFF; 00130 offset += sizeof(this->time_step); 00131 union { 00132 double real; 00133 uint64_t base; 00134 } u_command; 00135 u_command.real = this->command; 00136 *(outbuffer + offset + 0) = (u_command.base >> (8 * 0)) & 0xFF; 00137 *(outbuffer + offset + 1) = (u_command.base >> (8 * 1)) & 0xFF; 00138 *(outbuffer + offset + 2) = (u_command.base >> (8 * 2)) & 0xFF; 00139 *(outbuffer + offset + 3) = (u_command.base >> (8 * 3)) & 0xFF; 00140 *(outbuffer + offset + 4) = (u_command.base >> (8 * 4)) & 0xFF; 00141 *(outbuffer + offset + 5) = (u_command.base >> (8 * 5)) & 0xFF; 00142 *(outbuffer + offset + 6) = (u_command.base >> (8 * 6)) & 0xFF; 00143 *(outbuffer + offset + 7) = (u_command.base >> (8 * 7)) & 0xFF; 00144 offset += sizeof(this->command); 00145 union { 00146 double real; 00147 uint64_t base; 00148 } u_p; 00149 u_p.real = this->p; 00150 *(outbuffer + offset + 0) = (u_p.base >> (8 * 0)) & 0xFF; 00151 *(outbuffer + offset + 1) = (u_p.base >> (8 * 1)) & 0xFF; 00152 *(outbuffer + offset + 2) = (u_p.base >> (8 * 2)) & 0xFF; 00153 *(outbuffer + offset + 3) = (u_p.base >> (8 * 3)) & 0xFF; 00154 *(outbuffer + offset + 4) = (u_p.base >> (8 * 4)) & 0xFF; 00155 *(outbuffer + offset + 5) = (u_p.base >> (8 * 5)) & 0xFF; 00156 *(outbuffer + offset + 6) = (u_p.base >> (8 * 6)) & 0xFF; 00157 *(outbuffer + offset + 7) = (u_p.base >> (8 * 7)) & 0xFF; 00158 offset += sizeof(this->p); 00159 union { 00160 double real; 00161 uint64_t base; 00162 } u_i; 00163 u_i.real = this->i; 00164 *(outbuffer + offset + 0) = (u_i.base >> (8 * 0)) & 0xFF; 00165 *(outbuffer + offset + 1) = (u_i.base >> (8 * 1)) & 0xFF; 00166 *(outbuffer + offset + 2) = (u_i.base >> (8 * 2)) & 0xFF; 00167 *(outbuffer + offset + 3) = (u_i.base >> (8 * 3)) & 0xFF; 00168 *(outbuffer + offset + 4) = (u_i.base >> (8 * 4)) & 0xFF; 00169 *(outbuffer + offset + 5) = (u_i.base >> (8 * 5)) & 0xFF; 00170 *(outbuffer + offset + 6) = (u_i.base >> (8 * 6)) & 0xFF; 00171 *(outbuffer + offset + 7) = (u_i.base >> (8 * 7)) & 0xFF; 00172 offset += sizeof(this->i); 00173 union { 00174 double real; 00175 uint64_t base; 00176 } u_d; 00177 u_d.real = this->d; 00178 *(outbuffer + offset + 0) = (u_d.base >> (8 * 0)) & 0xFF; 00179 *(outbuffer + offset + 1) = (u_d.base >> (8 * 1)) & 0xFF; 00180 *(outbuffer + offset + 2) = (u_d.base >> (8 * 2)) & 0xFF; 00181 *(outbuffer + offset + 3) = (u_d.base >> (8 * 3)) & 0xFF; 00182 *(outbuffer + offset + 4) = (u_d.base >> (8 * 4)) & 0xFF; 00183 *(outbuffer + offset + 5) = (u_d.base >> (8 * 5)) & 0xFF; 00184 *(outbuffer + offset + 6) = (u_d.base >> (8 * 6)) & 0xFF; 00185 *(outbuffer + offset + 7) = (u_d.base >> (8 * 7)) & 0xFF; 00186 offset += sizeof(this->d); 00187 union { 00188 double real; 00189 uint64_t base; 00190 } u_i_clamp; 00191 u_i_clamp.real = this->i_clamp; 00192 *(outbuffer + offset + 0) = (u_i_clamp.base >> (8 * 0)) & 0xFF; 00193 *(outbuffer + offset + 1) = (u_i_clamp.base >> (8 * 1)) & 0xFF; 00194 *(outbuffer + offset + 2) = (u_i_clamp.base >> (8 * 2)) & 0xFF; 00195 *(outbuffer + offset + 3) = (u_i_clamp.base >> (8 * 3)) & 0xFF; 00196 *(outbuffer + offset + 4) = (u_i_clamp.base >> (8 * 4)) & 0xFF; 00197 *(outbuffer + offset + 5) = (u_i_clamp.base >> (8 * 5)) & 0xFF; 00198 *(outbuffer + offset + 6) = (u_i_clamp.base >> (8 * 6)) & 0xFF; 00199 *(outbuffer + offset + 7) = (u_i_clamp.base >> (8 * 7)) & 0xFF; 00200 offset += sizeof(this->i_clamp); 00201 union { 00202 bool real; 00203 uint8_t base; 00204 } u_antiwindup; 00205 u_antiwindup.real = this->antiwindup; 00206 *(outbuffer + offset + 0) = (u_antiwindup.base >> (8 * 0)) & 0xFF; 00207 offset += sizeof(this->antiwindup); 00208 return offset; 00209 } 00210 00211 virtual int deserialize(unsigned char *inbuffer) 00212 { 00213 int offset = 0; 00214 offset += this->header.deserialize(inbuffer + offset); 00215 union { 00216 double real; 00217 uint64_t base; 00218 } u_set_point; 00219 u_set_point.base = 0; 00220 u_set_point.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00221 u_set_point.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00222 u_set_point.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00223 u_set_point.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00224 u_set_point.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00225 u_set_point.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00226 u_set_point.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00227 u_set_point.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00228 this->set_point = u_set_point.real; 00229 offset += sizeof(this->set_point); 00230 union { 00231 double real; 00232 uint64_t base; 00233 } u_process_value; 00234 u_process_value.base = 0; 00235 u_process_value.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00236 u_process_value.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00237 u_process_value.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00238 u_process_value.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00239 u_process_value.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00240 u_process_value.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00241 u_process_value.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00242 u_process_value.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00243 this->process_value = u_process_value.real; 00244 offset += sizeof(this->process_value); 00245 union { 00246 double real; 00247 uint64_t base; 00248 } u_process_value_dot; 00249 u_process_value_dot.base = 0; 00250 u_process_value_dot.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00251 u_process_value_dot.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00252 u_process_value_dot.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00253 u_process_value_dot.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00254 u_process_value_dot.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00255 u_process_value_dot.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00256 u_process_value_dot.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00257 u_process_value_dot.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00258 this->process_value_dot = u_process_value_dot.real; 00259 offset += sizeof(this->process_value_dot); 00260 union { 00261 double real; 00262 uint64_t base; 00263 } u_error; 00264 u_error.base = 0; 00265 u_error.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00266 u_error.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00267 u_error.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00268 u_error.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00269 u_error.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00270 u_error.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00271 u_error.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00272 u_error.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00273 this->error = u_error.real; 00274 offset += sizeof(this->error); 00275 union { 00276 double real; 00277 uint64_t base; 00278 } u_time_step; 00279 u_time_step.base = 0; 00280 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00281 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00282 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00283 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00284 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00285 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00286 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00287 u_time_step.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00288 this->time_step = u_time_step.real; 00289 offset += sizeof(this->time_step); 00290 union { 00291 double real; 00292 uint64_t base; 00293 } u_command; 00294 u_command.base = 0; 00295 u_command.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00296 u_command.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00297 u_command.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00298 u_command.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00299 u_command.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00300 u_command.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00301 u_command.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00302 u_command.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00303 this->command = u_command.real; 00304 offset += sizeof(this->command); 00305 union { 00306 double real; 00307 uint64_t base; 00308 } u_p; 00309 u_p.base = 0; 00310 u_p.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00311 u_p.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00312 u_p.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00313 u_p.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00314 u_p.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00315 u_p.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00316 u_p.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00317 u_p.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00318 this->p = u_p.real; 00319 offset += sizeof(this->p); 00320 union { 00321 double real; 00322 uint64_t base; 00323 } u_i; 00324 u_i.base = 0; 00325 u_i.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00326 u_i.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00327 u_i.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00328 u_i.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00329 u_i.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00330 u_i.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00331 u_i.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00332 u_i.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00333 this->i = u_i.real; 00334 offset += sizeof(this->i); 00335 union { 00336 double real; 00337 uint64_t base; 00338 } u_d; 00339 u_d.base = 0; 00340 u_d.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00341 u_d.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00342 u_d.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00343 u_d.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00344 u_d.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00345 u_d.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00346 u_d.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00347 u_d.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00348 this->d = u_d.real; 00349 offset += sizeof(this->d); 00350 union { 00351 double real; 00352 uint64_t base; 00353 } u_i_clamp; 00354 u_i_clamp.base = 0; 00355 u_i_clamp.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00356 u_i_clamp.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00357 u_i_clamp.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00358 u_i_clamp.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00359 u_i_clamp.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00360 u_i_clamp.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00361 u_i_clamp.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00362 u_i_clamp.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00363 this->i_clamp = u_i_clamp.real; 00364 offset += sizeof(this->i_clamp); 00365 union { 00366 bool real; 00367 uint8_t base; 00368 } u_antiwindup; 00369 u_antiwindup.base = 0; 00370 u_antiwindup.base |= ((uint8_t) (*(inbuffer + offset + 0))) << (8 * 0); 00371 this->antiwindup = u_antiwindup.real; 00372 offset += sizeof(this->antiwindup); 00373 return offset; 00374 } 00375 00376 const char * getType(){ return "control_msgs/JointControllerState"; }; 00377 const char * getMD5(){ return "987ad85e4756f3aef7f1e5e7fe0595d1"; }; 00378 00379 }; 00380 00381 } 00382 #endif
Generated on Tue Jul 12 2022 18:49:19 by
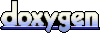