Just changed OUTPUT_SIZE and INPUT_SIZE in ros/node_handle.h
Dependents: WRS2020_mecanum_node
Config.h
00001 #ifndef _ROS_dynamic_reconfigure_Config_h 00002 #define _ROS_dynamic_reconfigure_Config_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 #include "dynamic_reconfigure/BoolParameter.h" 00009 #include "dynamic_reconfigure/IntParameter.h" 00010 #include "dynamic_reconfigure/StrParameter.h" 00011 #include "dynamic_reconfigure/DoubleParameter.h" 00012 #include "dynamic_reconfigure/GroupState.h" 00013 00014 namespace dynamic_reconfigure 00015 { 00016 00017 class Config : public ros::Msg 00018 { 00019 public: 00020 uint32_t bools_length; 00021 typedef dynamic_reconfigure::BoolParameter _bools_type; 00022 _bools_type st_bools; 00023 _bools_type * bools; 00024 uint32_t ints_length; 00025 typedef dynamic_reconfigure::IntParameter _ints_type; 00026 _ints_type st_ints; 00027 _ints_type * ints; 00028 uint32_t strs_length; 00029 typedef dynamic_reconfigure::StrParameter _strs_type; 00030 _strs_type st_strs; 00031 _strs_type * strs; 00032 uint32_t doubles_length; 00033 typedef dynamic_reconfigure::DoubleParameter _doubles_type; 00034 _doubles_type st_doubles; 00035 _doubles_type * doubles; 00036 uint32_t groups_length; 00037 typedef dynamic_reconfigure::GroupState _groups_type; 00038 _groups_type st_groups; 00039 _groups_type * groups; 00040 00041 Config(): 00042 bools_length(0), bools(NULL), 00043 ints_length(0), ints(NULL), 00044 strs_length(0), strs(NULL), 00045 doubles_length(0), doubles(NULL), 00046 groups_length(0), groups(NULL) 00047 { 00048 } 00049 00050 virtual int serialize(unsigned char *outbuffer) const 00051 { 00052 int offset = 0; 00053 *(outbuffer + offset + 0) = (this->bools_length >> (8 * 0)) & 0xFF; 00054 *(outbuffer + offset + 1) = (this->bools_length >> (8 * 1)) & 0xFF; 00055 *(outbuffer + offset + 2) = (this->bools_length >> (8 * 2)) & 0xFF; 00056 *(outbuffer + offset + 3) = (this->bools_length >> (8 * 3)) & 0xFF; 00057 offset += sizeof(this->bools_length); 00058 for( uint32_t i = 0; i < bools_length; i++){ 00059 offset += this->bools[i].serialize(outbuffer + offset); 00060 } 00061 *(outbuffer + offset + 0) = (this->ints_length >> (8 * 0)) & 0xFF; 00062 *(outbuffer + offset + 1) = (this->ints_length >> (8 * 1)) & 0xFF; 00063 *(outbuffer + offset + 2) = (this->ints_length >> (8 * 2)) & 0xFF; 00064 *(outbuffer + offset + 3) = (this->ints_length >> (8 * 3)) & 0xFF; 00065 offset += sizeof(this->ints_length); 00066 for( uint32_t i = 0; i < ints_length; i++){ 00067 offset += this->ints[i].serialize(outbuffer + offset); 00068 } 00069 *(outbuffer + offset + 0) = (this->strs_length >> (8 * 0)) & 0xFF; 00070 *(outbuffer + offset + 1) = (this->strs_length >> (8 * 1)) & 0xFF; 00071 *(outbuffer + offset + 2) = (this->strs_length >> (8 * 2)) & 0xFF; 00072 *(outbuffer + offset + 3) = (this->strs_length >> (8 * 3)) & 0xFF; 00073 offset += sizeof(this->strs_length); 00074 for( uint32_t i = 0; i < strs_length; i++){ 00075 offset += this->strs[i].serialize(outbuffer + offset); 00076 } 00077 *(outbuffer + offset + 0) = (this->doubles_length >> (8 * 0)) & 0xFF; 00078 *(outbuffer + offset + 1) = (this->doubles_length >> (8 * 1)) & 0xFF; 00079 *(outbuffer + offset + 2) = (this->doubles_length >> (8 * 2)) & 0xFF; 00080 *(outbuffer + offset + 3) = (this->doubles_length >> (8 * 3)) & 0xFF; 00081 offset += sizeof(this->doubles_length); 00082 for( uint32_t i = 0; i < doubles_length; i++){ 00083 offset += this->doubles[i].serialize(outbuffer + offset); 00084 } 00085 *(outbuffer + offset + 0) = (this->groups_length >> (8 * 0)) & 0xFF; 00086 *(outbuffer + offset + 1) = (this->groups_length >> (8 * 1)) & 0xFF; 00087 *(outbuffer + offset + 2) = (this->groups_length >> (8 * 2)) & 0xFF; 00088 *(outbuffer + offset + 3) = (this->groups_length >> (8 * 3)) & 0xFF; 00089 offset += sizeof(this->groups_length); 00090 for( uint32_t i = 0; i < groups_length; i++){ 00091 offset += this->groups[i].serialize(outbuffer + offset); 00092 } 00093 return offset; 00094 } 00095 00096 virtual int deserialize(unsigned char *inbuffer) 00097 { 00098 int offset = 0; 00099 uint32_t bools_lengthT = ((uint32_t) (*(inbuffer + offset))); 00100 bools_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00101 bools_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00102 bools_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00103 offset += sizeof(this->bools_length); 00104 if(bools_lengthT > bools_length) 00105 this->bools = (dynamic_reconfigure::BoolParameter*)realloc(this->bools, bools_lengthT * sizeof(dynamic_reconfigure::BoolParameter)); 00106 bools_length = bools_lengthT; 00107 for( uint32_t i = 0; i < bools_length; i++){ 00108 offset += this->st_bools.deserialize(inbuffer + offset); 00109 memcpy( &(this->bools[i]), &(this->st_bools), sizeof(dynamic_reconfigure::BoolParameter)); 00110 } 00111 uint32_t ints_lengthT = ((uint32_t) (*(inbuffer + offset))); 00112 ints_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00113 ints_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00114 ints_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00115 offset += sizeof(this->ints_length); 00116 if(ints_lengthT > ints_length) 00117 this->ints = (dynamic_reconfigure::IntParameter*)realloc(this->ints, ints_lengthT * sizeof(dynamic_reconfigure::IntParameter)); 00118 ints_length = ints_lengthT; 00119 for( uint32_t i = 0; i < ints_length; i++){ 00120 offset += this->st_ints.deserialize(inbuffer + offset); 00121 memcpy( &(this->ints[i]), &(this->st_ints), sizeof(dynamic_reconfigure::IntParameter)); 00122 } 00123 uint32_t strs_lengthT = ((uint32_t) (*(inbuffer + offset))); 00124 strs_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00125 strs_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00126 strs_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00127 offset += sizeof(this->strs_length); 00128 if(strs_lengthT > strs_length) 00129 this->strs = (dynamic_reconfigure::StrParameter*)realloc(this->strs, strs_lengthT * sizeof(dynamic_reconfigure::StrParameter)); 00130 strs_length = strs_lengthT; 00131 for( uint32_t i = 0; i < strs_length; i++){ 00132 offset += this->st_strs.deserialize(inbuffer + offset); 00133 memcpy( &(this->strs[i]), &(this->st_strs), sizeof(dynamic_reconfigure::StrParameter)); 00134 } 00135 uint32_t doubles_lengthT = ((uint32_t) (*(inbuffer + offset))); 00136 doubles_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00137 doubles_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00138 doubles_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00139 offset += sizeof(this->doubles_length); 00140 if(doubles_lengthT > doubles_length) 00141 this->doubles = (dynamic_reconfigure::DoubleParameter*)realloc(this->doubles, doubles_lengthT * sizeof(dynamic_reconfigure::DoubleParameter)); 00142 doubles_length = doubles_lengthT; 00143 for( uint32_t i = 0; i < doubles_length; i++){ 00144 offset += this->st_doubles.deserialize(inbuffer + offset); 00145 memcpy( &(this->doubles[i]), &(this->st_doubles), sizeof(dynamic_reconfigure::DoubleParameter)); 00146 } 00147 uint32_t groups_lengthT = ((uint32_t) (*(inbuffer + offset))); 00148 groups_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00149 groups_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00150 groups_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00151 offset += sizeof(this->groups_length); 00152 if(groups_lengthT > groups_length) 00153 this->groups = (dynamic_reconfigure::GroupState*)realloc(this->groups, groups_lengthT * sizeof(dynamic_reconfigure::GroupState)); 00154 groups_length = groups_lengthT; 00155 for( uint32_t i = 0; i < groups_length; i++){ 00156 offset += this->st_groups.deserialize(inbuffer + offset); 00157 memcpy( &(this->groups[i]), &(this->st_groups), sizeof(dynamic_reconfigure::GroupState)); 00158 } 00159 return offset; 00160 } 00161 00162 const char * getType(){ return "dynamic_reconfigure/Config"; }; 00163 const char * getMD5(){ return "958f16a05573709014982821e6822580"; }; 00164 00165 }; 00166 00167 } 00168 #endif
Generated on Tue Jul 12 2022 18:49:18 by
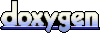