My Version of CreaBotLib
Fork of CreaBotLib by
Creabot Class Reference
Synchronous Control of 2 wheels as part of a two wheel robot. More...
#include <CreaBot.h>
Public Member Functions | |
Creabot (CreaMot *left, CreaMot *right, float wheel_diam_cm, float bot_diam_cm) | |
Create a Creabot object with 2 wheels. | |
TWheelsState | getState () |
Property access to wheel state, indicating which of the wheels are moving. | |
void | setCallBack (void(*Acallback)(int status)) |
Setup a Callback method. | |
void | setSpeed (float AbotSpeed_cm_sec) |
High level: set bot-speed parameter for all future wheel commands. | |
void | qMove (BotCmdVerb Acommand, float Aparam) |
High level, queued: move bot according to command and parameter. | |
void | qMove (BotCmdVerb Acommand, float Aturn_angle_deg, float Adist_cm) |
High level, queued : move bot according to command and parameters. | |
void | qExecuteNext () |
Execute and remove the oldest element at the tail of the Queue. | |
int | qCount () |
Public access of Queue used Count. | |
void | iMoveAndWait (BotCmdVerb Acommand, float Aparam) |
High level, immediate: move bot and wait for movement end. | |
void | iMoveAndWait (BotCmdVerb Acommand, float Aturn_angle_deg, float Adist_cm) |
High level, immediate: move bot and wait for movement end. | |
void | iMove (BotCmdVerb Acommand, float Aparam) |
High level, immediate: move bot according to command and parameter Composes a BotCommand and passes it to executeCommand(). | |
void | iMove (BotCmdVerb Acommand, float Aturn_angle_deg, float Adist_cm) |
High level, immediate: move bot according to command and parameters Composes a BotCommand and passes it to executeCommand(). | |
void | iExeCommand (BotCommand *cmd) |
High level, immediate: move bot according to prefilled command structures. | |
void | iWaitEnd () |
High Level: spends all time with waits, returns only once wheelState = LRWHEELS_STOP, not recommended due its blocking behavior. | |
void | iWaitEnd (uint32_t max_wait_ms) |
High Level: spends all time with waits, returns only once wheelState = LRWHEELS_STOP, but waits no more than max_wait_ms milli seconds. | |
void | iStop () |
High level, immediate: Both wheels get stopped, and turned off updates the wheel state wheelsState, does not call the callback handler! It does not empty the queue. | |
void | moveForward (float dist_cm) |
Mid level, immediate: advance bot straight forward for a given distance, Preset the speed using setSpeed(). | |
void | moveBackward (float dist_cm) |
Mid level, immediate: reverse bot straight backwards for a given distance, Preset the speed using setSpeed(). | |
void | moveRight (float turn_angle_deg, float center_cm) |
Mid level, immediate: turn bot forward right, around a radius that is center_cm away from the right wheel, Preset the speed using setSpeed(). | |
void | moveLeft (float turn_angle_deg) |
Mid level, immediate: turn bot forward left, around a radius twice the bot size, Same as moveLeft(turn_angle_deg, 0); Preset the speed using setSpeed(). | |
void | moveLeft (float turn_angle_deg, float center_cm) |
Mid level, immediate: turn bot forward left, around a radius that is center_cm away from the left wheel, Preset the speed using setSpeed(). | |
void | moveBackRight (float turn_angle_deg, float center_cm) |
Mid level, immediate: turn bot backwards right, around a radius that is center_cm away from the right wheel, Preset the speed using setSpeed(). | |
void | moveBackLeft (float turn_angle_deg) |
Mid level, immediate: turn bot backwards left, around a radius twice the bot size, Same as moveBackLeft(turn_angle_deg, 0); Preset the speed using setSpeed(). | |
void | moveBackLeft (float turn_angle_deg, float center_cm) |
Mid level, immediate: turn bot backwards left, around a radius that is center_cm away from the left wheel, Preset the speed using setSpeed(). | |
void | moveRotate (float turn_angle_deg) |
Mid level, immediate: turn bot on its place for a given angle. |
Detailed Description
Synchronous Control of 2 wheels as part of a two wheel robot.
Handles two instances of CreaMot from motor.h library simultaneously. Using the set distance between the wheels, allows to run pecise curves.
A first set of movement functions starting with qXXX are using command verbs, these commands are queued up in a waiting queue, and are executed one by one in programmed order.
A second set of movement functions starting with iXXX are also using command verbs, however these commands are executed immediately, they override each other if issued while the previous movement still ongoing
A third set of movements functions starting with moveXXXX are provided, each function performs one specific movement, also immediately. So they also override each other, and collide with queued commands.
A callback is supplied to react to the end of all programmed movements.
Example:
// --- Define the Four PINs & Time of movement used for wheel drive ----- CreaMot wheelLeft(PA_12, PB_0, PB_1, PB_6); // Declare first the 2 wheels (to avoid to have an object with 8 pins to create) CreaMot wheelRight(PA_5,PA_4,PA_3,PA_1); CreaBot mybot(&wheelLeft, &WheelRight, 10.0f, 13.0f); // insert the wheels and indicate wheel diameter (10cm) and distance between wheels (13cm) int main() { mybot.setSpeed(12.5); // Preset speed to 12.5cm/s mybot.iMove(FORWARD,10); // Go forward of 10cm mybot.iWaitEnd(); // Wait end of Move mybot.iMove(ROTATE,90); // Start rotation of 90° around the center between wheels (two wheels running in same direction at same speed) mybot.iMove(BACKWARD,40); // Stop immediately the rotation and go backward of 40cm mybot.iWaitEnd(); // Wait end of Backward mybot.iMoveAndWait(LEFT,60); // Move Left of 60° in circle, center being the left wheel (off). Wait end of move mybot.iWaitEnd(); // Not needed, as already waited... mybot.iMoveAndWait(RIGHT,45, 33); // Move Right of 45°, center being at 33cm of the right wheel. Right wheel moving slower and on a shorter distance than left one. mybot.iMoveAndWait(ROTATE,90); mybot.iMove(ROTATE,-90); // Opposite direction. mybot.iWaitEnd(6); // with time-out => if after 6s the move is not ended, continue the execution mybot.iStop(); // Stop the movement in case it was not ended before ... // Same with a Queue of command, opposite to the move, receiving a new command will not stop the current execution, but the bot will store it. mybot.qMove(FORWARD,10); // Already starting... mybot.qMove(BACKWARD,10); // will wait end of previous command to go mybot.qMove(ROTATE,120.0); mybot.qMove(LEFT, 30, 120); mybot.qMove(RIGHT, 25); ... // insert other code here that executes while the motors continue to move mybot.iWaitEnd(100000); // wait until Queue end... can flush anytime with stopMove... mybot.qEmpty(); // before end... Flush the Queue and remove all instructions… while(1) { }; }
Definition at line 129 of file CreaBot.h.
Constructor & Destructor Documentation
Creabot | ( | CreaMot * | left, |
CreaMot * | right, | ||
float | wheel_diam_cm, | ||
float | bot_diam_cm | ||
) |
Create a Creabot object with 2 wheels.
- Parameters:
-
[in] <left> CreaMot object, corresponding to left wheel of the Creabot [in] <right> CreaMot object, corresponding to right wheel of the Creabot [in] <wheel_diam_cm> Diameter in cm of the wheels (both must be the same diameter) [in] <bot_diam_cm> Distance cm between center of left wheel and center of right wheel
Definition at line 26 of file CreaBot.cpp.
Member Function Documentation
TWheelsState getState | ( | ) |
void iExeCommand | ( | BotCommand * | cmd ) |
High level, immediate: move bot according to prefilled command structures.
Recommended to use iMove() methods to fill the command structure correctly. Branches to the moveXXXX() methods. For details see docs for those methods. Preset the speed using setSpeed(). Warning: Collides with queued commands if called individually.
- Parameters:
-
[in] <*cmd> Pointer to type BotCommand, the prefilled command structure,
Recommended to use iMove() methods to fill the command structure correctly. Branches to the moveXXXX() methods. For details see docs for those methods. Warning: Collides with queued commands if called individually.
- Parameters:
-
[in] <*cmd> Pointer to type BotCommand, the prefilled command structure,
Definition at line 149 of file CreaBot.cpp.
void iMove | ( | BotCmdVerb | Acommand, |
float | Aparam | ||
) |
High level, immediate: move bot according to command and parameter Composes a BotCommand and passes it to executeCommand().
Use for commands that need only one parameter: FORWARD, BACKWARD, ROTATE For details refer to docu of moveForward(), moveBackward(), moveRotate(). Preset the speed using setSpeed(). Warning: Collides with queued commands.
- Parameters:
-
[in] <Acommand> Requested movement, of type BotCmdVerb. [in] <Aparam> Requested amount, defines angle for ROTATE, or distance for FORWARD, BACKWARD.
Definition at line 135 of file CreaBot.cpp.
void iMove | ( | BotCmdVerb | Acommand, |
float | Aturn_angle_deg, | ||
float | Adist_cm | ||
) |
High level, immediate: move bot according to command and parameters Composes a BotCommand and passes it to executeCommand().
Use for commands that need two parameters: LEFT, RIGHT, BACKLEFT, BACKRIGHT For details refer to docu of moveLeft(), moveRight(), moveBackLeft(), moveBackRight(). Preset the speed using setSpeed(). Warning: Collides with queued commands.
- Parameters:
-
[in] <Acommand> Requested movement, of type BotCmdVerb. [in] <Aturn_angle_deg> Requested angle in degrees. [in] <Adist_cm> Requested distance in centimeters.
Definition at line 138 of file CreaBot.cpp.
void iMoveAndWait | ( | BotCmdVerb | Acommand, |
float | Aparam | ||
) |
High level, immediate: move bot and wait for movement end.
Simple wrapper for iMove() and iWaitEnd(). Refer to those methods for further docs.
Definition at line 125 of file CreaBot.cpp.
void iMoveAndWait | ( | BotCmdVerb | Acommand, |
float | Aturn_angle_deg, | ||
float | Adist_cm | ||
) |
High level, immediate: move bot and wait for movement end.
Simple wrapper for iMove() and iWaitEnd(). Refer to those methods for further docs.
Definition at line 130 of file CreaBot.cpp.
void iStop | ( | ) |
High level, immediate: Both wheels get stopped, and turned off updates the wheel state wheelsState, does not call the callback handler! It does not empty the queue.
Adding new elements into the queue after calling iStop() Would continue using all commands still in the queue.
Definition at line 189 of file CreaBot.cpp.
void iWaitEnd | ( | ) |
High Level: spends all time with waits, returns only once wheelState = LRWHEELS_STOP, not recommended due its blocking behavior.
Definition at line 178 of file CreaBot.cpp.
void iWaitEnd | ( | uint32_t | max_wait_ms ) |
High Level: spends all time with waits, returns only once wheelState = LRWHEELS_STOP, but waits no more than max_wait_ms milli seconds.
Not recommended due its blocking behavior
Definition at line 183 of file CreaBot.cpp.
void moveBackLeft | ( | float | turn_angle_deg ) |
Mid level, immediate: turn bot backwards left, around a radius twice the bot size, Same as moveBackLeft(turn_angle_deg, 0); Preset the speed using setSpeed().
Warning: Collides with queued commands.
Definition at line 298 of file CreaBot.cpp.
void moveBackLeft | ( | float | turn_angle_deg, |
float | center_cm | ||
) |
Mid level, immediate: turn bot backwards left, around a radius that is center_cm away from the left wheel, Preset the speed using setSpeed().
Warning: Collides with queued commands.
Definition at line 293 of file CreaBot.cpp.
void moveBackRight | ( | float | turn_angle_deg, |
float | center_cm | ||
) |
Mid level, immediate: turn bot backwards right, around a radius that is center_cm away from the right wheel, Preset the speed using setSpeed().
Warning: Collides with queued commands.
Definition at line 259 of file CreaBot.cpp.
void moveBackward | ( | float | dist_cm ) |
Mid level, immediate: reverse bot straight backwards for a given distance, Preset the speed using setSpeed().
Warning: Collides with queued commands.
Definition at line 224 of file CreaBot.cpp.
void moveForward | ( | float | dist_cm ) |
Mid level, immediate: advance bot straight forward for a given distance, Preset the speed using setSpeed().
Warning: Collides with queued commands.
Definition at line 214 of file CreaBot.cpp.
void moveLeft | ( | float | turn_angle_deg ) |
Mid level, immediate: turn bot forward left, around a radius twice the bot size, Same as moveLeft(turn_angle_deg, 0); Preset the speed using setSpeed().
Warning: Collides with queued commands.
Definition at line 269 of file CreaBot.cpp.
void moveLeft | ( | float | turn_angle_deg, |
float | center_cm | ||
) |
Mid level, immediate: turn bot forward left, around a radius that is center_cm away from the left wheel, Preset the speed using setSpeed().
Warning: Collides with queued commands.
Definition at line 278 of file CreaBot.cpp.
void moveRight | ( | float | turn_angle_deg, |
float | center_cm | ||
) |
Mid level, immediate: turn bot forward right, around a radius that is center_cm away from the right wheel, Preset the speed using setSpeed().
Warning: Collides with queued commands.
Definition at line 244 of file CreaBot.cpp.
void moveRotate | ( | float | turn_angle_deg ) |
Mid level, immediate: turn bot on its place for a given angle.
positive angles turn right, negative angles turn left, Preset the speed using setSpeed(). Warning: Collides with queued commands.
Definition at line 303 of file CreaBot.cpp.
int qCount | ( | ) |
void qExecuteNext | ( | ) |
Execute and remove the oldest element at the tail of the Queue.
If Queue is empty, nothing happens Otherwise executes oldest commands from the tail of the ring buffer at position readIdx, Then advances the read index once.
Definition at line 104 of file CreaBot.cpp.
void qMove | ( | BotCmdVerb | Acommand, |
float | Aparam | ||
) |
High level, queued: move bot according to command and parameter.
Composes a BotCommand and appends it to the queue for queued execution. Use for commands that need only one parameter: FORWARD, BACKWARD, ROTATE For details refer to docu of moveForward(), moveBackward(), moveRotate(). Preset the speed using setSpeed().
- Parameters:
-
[in] <Acommand> Requested movement, of type BotCmdVerb. [in] <Aparam> Requested amount, defines angle for ROTATE, or distance for FORWARD, BACKWARD.
Definition at line 62 of file CreaBot.cpp.
void qMove | ( | BotCmdVerb | Acommand, |
float | Aturn_angle_deg, | ||
float | Adist_cm | ||
) |
High level, queued : move bot according to command and parameters.
Composes a BotCommand and appends it to the queue for queued execution. Use for commands that need two parameters: LEFT, RIGHT, BACKLEFT, BACKRIGHT For details refer to docu of moveLeft(), moveRight(), moveBackLeft(), moveBackRight().
Reserves a new command element at the head of the Queue
Adds incoming commands at the head of the ring buffer at position writeIdx, If Queue is full, it passes back NULL Otherwise Advances the write index once and returns a pointer the next free command struct. The caller then must fill the command structure at that position. Do not free memory associated to the pointer.
- Parameters:
-
[in] <Acommand> Requested movement, of type BotCmdVerb. [in] <Aturn_angle_deg> Requested angle in degrees. [in] <Adist_cm> Requested distance in centimeters.
Definition at line 80 of file CreaBot.cpp.
void setCallBack | ( | void(*)(int status) | Acallback ) |
void setSpeed | ( | float | AbotSpeed_cm_sec ) |
High level: set bot-speed parameter for all future wheel commands.
The set speed is used for immediate as well as the queued movements. In a curve movement it determines the speed of the outer wheel.
- Parameters:
-
[in] <AbotSpeed_cm_sec> requested movement speed
Definition at line 206 of file CreaBot.cpp.
Generated on Wed Jul 13 2022 17:45:26 by
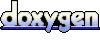