Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
CMSIS Core Register Access Functions
[Functions and Instructions Reference]
Functions | |
static __INLINE uint32_t | __get_CONTROL (void) |
Get Control Register. | |
static __INLINE void | __set_CONTROL (uint32_t control) |
Set Control Register. | |
static __INLINE uint32_t | __get_IPSR (void) |
Get IPSR Register. | |
static __INLINE uint32_t | __get_APSR (void) |
Get APSR Register. | |
static __INLINE uint32_t | __get_xPSR (void) |
Get xPSR Register. | |
static __INLINE uint32_t | __get_PSP (void) |
Get Process Stack Pointer. | |
static __INLINE void | __set_PSP (uint32_t topOfProcStack) |
Set Process Stack Pointer. | |
static __INLINE uint32_t | __get_MSP (void) |
Get Main Stack Pointer. | |
static __INLINE void | __set_MSP (uint32_t topOfMainStack) |
Set Main Stack Pointer. | |
static __INLINE uint32_t | __get_PRIMASK (void) |
Get Priority Mask. | |
static __INLINE void | __set_PRIMASK (uint32_t priMask) |
Set Priority Mask. | |
static __INLINE uint32_t | __get_BASEPRI (void) |
Get Base Priority. | |
static __INLINE void | __set_BASEPRI (uint32_t basePri) |
Set Base Priority. | |
static __INLINE uint32_t | __get_FAULTMASK (void) |
Get Fault Mask. | |
static __INLINE void | __set_FAULTMASK (uint32_t faultMask) |
Set Fault Mask. | |
static __INLINE uint32_t | __get_FPSCR (void) |
Get FPSCR. | |
static __INLINE void | __set_FPSCR (uint32_t fpscr) |
Set FPSCR. | |
__attribute__ ((always_inline)) static __INLINE void __enable_irq(void) | |
Enable IRQ Interrupts. |
Function Documentation
__attribute__ | ( | (always_inline) | ) |
Enable IRQ Interrupts.
No Operation.
STR Exclusive (8 bit)
LDR Exclusive (32 bit)
LDR Exclusive (16 bit)
LDR Exclusive (8 bit)
Reverse bit order of value.
Reverse byte order in signed short value.
Reverse byte order (16 bit)
Reverse byte order (32 bit)
Data Memory Barrier.
Data Synchronization Barrier.
Instruction Synchronization Barrier.
Send Event.
Wait For Event.
Wait For Interrupt.
Set FPSCR.
Get FPSCR.
Set Fault Mask.
Get Fault Mask.
Set Base Priority.
Get Base Priority.
Disable FIQ.
Enable FIQ.
Set Priority Mask.
Get Priority Mask.
Set Main Stack Pointer.
Get Main Stack Pointer.
Set Process Stack Pointer.
Get Process Stack Pointer.
Get xPSR Register.
Get APSR Register.
Get IPSR Register.
Set Control Register.
Get Control Register.
Disable IRQ Interrupts.
This function enables IRQ interrupts by clearing the I-bit in the CPSR. Can only be executed in Privileged modes.
This function disables IRQ interrupts by setting the I-bit in the CPSR. Can only be executed in Privileged modes.
This function returns the content of the Control Register.
- Returns:
- Control Register value
This function writes the given value to the Control Register.
- Parameters:
-
[in] control Control Register value to set
This function returns the content of the IPSR Register.
- Returns:
- IPSR Register value
This function returns the content of the APSR Register.
- Returns:
- APSR Register value
This function returns the content of the xPSR Register.
- Returns:
- xPSR Register value
This function returns the current value of the Process Stack Pointer (PSP).
- Returns:
- PSP Register value
This function assigns the given value to the Process Stack Pointer (PSP).
- Parameters:
-
[in] topOfProcStack Process Stack Pointer value to set
This function returns the current value of the Main Stack Pointer (MSP).
- Returns:
- MSP Register value
This function assigns the given value to the Main Stack Pointer (MSP).
- Parameters:
-
[in] topOfMainStack Main Stack Pointer value to set
This function returns the current state of the priority mask bit from the Priority Mask Register.
- Returns:
- Priority Mask value
This function assigns the given value to the Priority Mask Register.
- Parameters:
-
[in] priMask Priority Mask
This function enables FIQ interrupts by clearing the F-bit in the CPSR. Can only be executed in Privileged modes.
This function disables FIQ interrupts by setting the F-bit in the CPSR. Can only be executed in Privileged modes.
This function returns the current value of the Base Priority register.
- Returns:
- Base Priority register value
This function assigns the given value to the Base Priority register.
- Parameters:
-
[in] basePri Base Priority value to set
This function returns the current value of the Fault Mask register.
- Returns:
- Fault Mask register value
This function assigns the given value to the Fault Mask register.
- Parameters:
-
[in] faultMask Fault Mask value to set
This function returns the current value of the Floating Point Status/Control register.
- Returns:
- Floating Point Status/Control register value
This function assigns the given value to the Floating Point Status/Control register.
- Parameters:
-
[in] fpscr Floating Point Status/Control value to set
Wait For Interrupt is a hint instruction that suspends execution until one of a number of events occurs.
Wait For Event is a hint instruction that permits the processor to enter a low-power state until one of a number of events occurs.
Send Event is a hint instruction. It causes an event to be signaled to the CPU.
Instruction Synchronization Barrier flushes the pipeline in the processor, so that all instructions following the ISB are fetched from cache or memory, after the instruction has been completed.
This function acts as a special kind of Data Memory Barrier. It completes when all explicit memory accesses before this instruction complete.
This function ensures the apparent order of the explicit memory operations before and after the instruction, without ensuring their completion.
This function reverses the byte order in integer value.
- Parameters:
-
[in] value Value to reverse
- Returns:
- Reversed value
This function reverses the byte order in two unsigned short values.
- Parameters:
-
[in] value Value to reverse
- Returns:
- Reversed value
This function reverses the byte order in a signed short value with sign extension to integer.
- Parameters:
-
[in] value Value to reverse
- Returns:
- Reversed value
This function reverses the bit order of the given value.
- Parameters:
-
[in] value Value to reverse
- Returns:
- Reversed value
This function performs a exclusive LDR command for 8 bit value.
- Parameters:
-
[in] ptr Pointer to data
- Returns:
- value of type uint8_t at (*ptr)
This function performs a exclusive LDR command for 16 bit values.
- Parameters:
-
[in] ptr Pointer to data
- Returns:
- value of type uint16_t at (*ptr)
This function performs a exclusive LDR command for 32 bit values.
- Parameters:
-
[in] ptr Pointer to data
- Returns:
- value of type uint32_t at (*ptr)
This function performs a exclusive STR command for 8 bit values.
- Parameters:
-
[in] value Value to store [in] ptr Pointer to location
- Returns:
- 0 Function succeeded
- 1 Function failed
No Operation does nothing. This instruction can be used for code alignment purposes.
Definition at line 308 of file LPC11U24/core_cmFunc.h.
static __INLINE uint32_t __get_APSR | ( | void | ) | [static] |
Get APSR Register.
This function returns the content of the APSR Register.
- Returns:
- APSR Register value
Definition at line 89 of file LPC11U24/core_cmFunc.h.
static __INLINE uint32_t __get_BASEPRI | ( | void | ) | [static] |
Get Base Priority.
This function returns the current value of the Base Priority register.
- Returns:
- Base Priority register value
Definition at line 211 of file LPC11U24/core_cmFunc.h.
static __INLINE uint32_t __get_CONTROL | ( | void | ) | [static] |
Get Control Register.
This function returns the content of the Control Register.
- Returns:
- Control Register value
Definition at line 50 of file LPC11U24/core_cmFunc.h.
static __INLINE uint32_t __get_FAULTMASK | ( | void | ) | [static] |
Get Fault Mask.
This function returns the current value of the Fault Mask register.
- Returns:
- Fault Mask register value
Definition at line 237 of file LPC11U24/core_cmFunc.h.
static __INLINE uint32_t __get_FPSCR | ( | void | ) | [static] |
Get FPSCR.
This function returns the current value of the Floating Point Status/Control register.
- Returns:
- Floating Point Status/Control register value
Definition at line 267 of file LPC11U24/core_cmFunc.h.
static __INLINE uint32_t __get_IPSR | ( | void | ) | [static] |
Get IPSR Register.
This function returns the content of the IPSR Register.
- Returns:
- IPSR Register value
Definition at line 76 of file LPC11U24/core_cmFunc.h.
static __INLINE uint32_t __get_MSP | ( | void | ) | [static] |
Get Main Stack Pointer.
This function returns the current value of the Main Stack Pointer (MSP).
- Returns:
- MSP Register value
Definition at line 141 of file LPC11U24/core_cmFunc.h.
static __INLINE uint32_t __get_PRIMASK | ( | void | ) | [static] |
Get Priority Mask.
This function returns the current state of the priority mask bit from the Priority Mask Register.
- Returns:
- Priority Mask value
Definition at line 167 of file LPC11U24/core_cmFunc.h.
static __INLINE uint32_t __get_PSP | ( | void | ) | [static] |
Get Process Stack Pointer.
This function returns the current value of the Process Stack Pointer (PSP).
- Returns:
- PSP Register value
Definition at line 115 of file LPC11U24/core_cmFunc.h.
static __INLINE uint32_t __get_xPSR | ( | void | ) | [static] |
Get xPSR Register.
This function returns the content of the xPSR Register.
- Returns:
- xPSR Register value
Definition at line 102 of file LPC11U24/core_cmFunc.h.
static __INLINE void __set_BASEPRI | ( | uint32_t | basePri ) | [static] |
Set Base Priority.
This function assigns the given value to the Base Priority register.
- Parameters:
-
[in] basePri Base Priority value to set
Definition at line 224 of file LPC11U24/core_cmFunc.h.
static __INLINE void __set_CONTROL | ( | uint32_t | control ) | [static] |
Set Control Register.
This function writes the given value to the Control Register.
- Parameters:
-
[in] control Control Register value to set
Definition at line 63 of file LPC11U24/core_cmFunc.h.
static __INLINE void __set_FAULTMASK | ( | uint32_t | faultMask ) | [static] |
Set Fault Mask.
This function assigns the given value to the Fault Mask register.
- Parameters:
-
[in] faultMask Fault Mask value to set
Definition at line 250 of file LPC11U24/core_cmFunc.h.
static __INLINE void __set_FPSCR | ( | uint32_t | fpscr ) | [static] |
Set FPSCR.
This function assigns the given value to the Floating Point Status/Control register.
- Parameters:
-
[in] fpscr Floating Point Status/Control value to set
Definition at line 284 of file LPC11U24/core_cmFunc.h.
static __INLINE void __set_MSP | ( | uint32_t | topOfMainStack ) | [static] |
Set Main Stack Pointer.
This function assigns the given value to the Main Stack Pointer (MSP).
- Parameters:
-
[in] topOfMainStack Main Stack Pointer value to set
Definition at line 154 of file LPC11U24/core_cmFunc.h.
static __INLINE void __set_PRIMASK | ( | uint32_t | priMask ) | [static] |
Set Priority Mask.
This function assigns the given value to the Priority Mask Register.
- Parameters:
-
[in] priMask Priority Mask
Definition at line 180 of file LPC11U24/core_cmFunc.h.
static __INLINE void __set_PSP | ( | uint32_t | topOfProcStack ) | [static] |
Set Process Stack Pointer.
This function assigns the given value to the Process Stack Pointer (PSP).
- Parameters:
-
[in] topOfProcStack Process Stack Pointer value to set
Definition at line 128 of file LPC11U24/core_cmFunc.h.
Generated on Tue Jul 12 2022 11:27:28 by
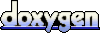