Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
core_cm0.h
00001 /**************************************************************************//** 00002 * @file core_cm0.h 00003 * @brief CMSIS Cortex-M0 Core Peripheral Access Layer Header File 00004 * @version V3.01 00005 * @date 06. March 2012 00006 * 00007 * @note 00008 * Copyright (C) 2009-2012 ARM Limited. All rights reserved. 00009 * 00010 * @par 00011 * ARM Limited (ARM) is supplying this software for use with Cortex-M 00012 * processor based microcontrollers. This file can be freely distributed 00013 * within development tools that are supporting such ARM based processors. 00014 * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 #if defined ( __ICCARM__ ) 00024 #pragma system_include /* treat file as system include file for MISRA check */ 00025 #endif 00026 00027 #ifdef __cplusplus 00028 extern "C" { 00029 #endif 00030 00031 #ifndef __CORE_CM0_H_GENERIC 00032 #define __CORE_CM0_H_GENERIC 00033 00034 /** \page CMSIS_MISRA_Exceptions MISRA-C:2004 Compliance Exceptions 00035 CMSIS violates the following MISRA-C:2004 rules: 00036 00037 \li Required Rule 8.5, object/function definition in header file.<br> 00038 Function definitions in header files are used to allow 'inlining'. 00039 00040 \li Required Rule 18.4, declaration of union type or object of union type: '{...}'.<br> 00041 Unions are used for effective representation of core registers. 00042 00043 \li Advisory Rule 19.7, Function-like macro defined.<br> 00044 Function-like macros are used to allow more efficient code. 00045 */ 00046 00047 00048 /******************************************************************************* 00049 * CMSIS definitions 00050 ******************************************************************************/ 00051 /** \ingroup Cortex_M0 00052 @{ 00053 */ 00054 00055 /* CMSIS CM0 definitions */ 00056 #define __CM0_CMSIS_VERSION_MAIN (0x03) /*!< [31:16] CMSIS HAL main version */ 00057 #define __CM0_CMSIS_VERSION_SUB (0x01) /*!< [15:0] CMSIS HAL sub version */ 00058 #define __CM0_CMSIS_VERSION ((__CM0_CMSIS_VERSION_MAIN << 16) | \ 00059 __CM0_CMSIS_VERSION_SUB ) /*!< CMSIS HAL version number */ 00060 00061 #define __CORTEX_M (0x00) /*!< Cortex-M Core */ 00062 00063 00064 #if defined ( __CC_ARM ) 00065 #define __ASM __asm /*!< asm keyword for ARM Compiler */ 00066 #define __INLINE __inline /*!< inline keyword for ARM Compiler */ 00067 #define __STATIC_INLINE static __inline 00068 00069 #elif defined ( __ICCARM__ ) 00070 #define __ASM __asm /*!< asm keyword for IAR Compiler */ 00071 #define __INLINE inline /*!< inline keyword for IAR Compiler. Only available in High optimization mode! */ 00072 #define __STATIC_INLINE static inline 00073 00074 #elif defined ( __GNUC__ ) 00075 #define __ASM __asm /*!< asm keyword for GNU Compiler */ 00076 #define __INLINE inline /*!< inline keyword for GNU Compiler */ 00077 #define __STATIC_INLINE static inline 00078 00079 #elif defined ( __TASKING__ ) 00080 #define __ASM __asm /*!< asm keyword for TASKING Compiler */ 00081 #define __INLINE inline /*!< inline keyword for TASKING Compiler */ 00082 #define __STATIC_INLINE static inline 00083 00084 #endif 00085 00086 /** __FPU_USED indicates whether an FPU is used or not. This core does not support an FPU at all 00087 */ 00088 #define __FPU_USED 0 00089 00090 #if defined ( __CC_ARM ) 00091 #if defined __TARGET_FPU_VFP 00092 #warning "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00093 #endif 00094 00095 #elif defined ( __ICCARM__ ) 00096 #if defined __ARMVFP__ 00097 #warning "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00098 #endif 00099 00100 #elif defined ( __GNUC__ ) 00101 #if defined (__VFP_FP__) && !defined(__SOFTFP__) 00102 #warning "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00103 #endif 00104 00105 #elif defined ( __TASKING__ ) 00106 /* add preprocessor checks */ 00107 #endif 00108 00109 #include <stdint.h> /* standard types definitions */ 00110 #include <core_cmInstr.h> /* Core Instruction Access */ 00111 #include <core_cmFunc.h> /* Core Function Access */ 00112 00113 #endif /* __CORE_CM0_H_GENERIC */ 00114 00115 #ifndef __CMSIS_GENERIC 00116 00117 #ifndef __CORE_CM0_H_DEPENDANT 00118 #define __CORE_CM0_H_DEPENDANT 00119 00120 /* check device defines and use defaults */ 00121 #if defined __CHECK_DEVICE_DEFINES 00122 #ifndef __CM0_REV 00123 #define __CM0_REV 0x0000 00124 #warning "__CM0_REV not defined in device header file; using default!" 00125 #endif 00126 00127 #ifndef __NVIC_PRIO_BITS 00128 #define __NVIC_PRIO_BITS 2 00129 #warning "__NVIC_PRIO_BITS not defined in device header file; using default!" 00130 #endif 00131 00132 #ifndef __Vendor_SysTickConfig 00133 #define __Vendor_SysTickConfig 0 00134 #warning "__Vendor_SysTickConfig not defined in device header file; using default!" 00135 #endif 00136 #endif 00137 00138 /* IO definitions (access restrictions to peripheral registers) */ 00139 /** 00140 \defgroup CMSIS_glob_defs CMSIS Global Defines 00141 00142 <strong>IO Type Qualifiers</strong> are used 00143 \li to specify the access to peripheral variables. 00144 \li for automatic generation of peripheral register debug information. 00145 */ 00146 #ifdef __cplusplus 00147 #define __I volatile /*!< Defines 'read only' permissions */ 00148 #else 00149 #define __I volatile const /*!< Defines 'read only' permissions */ 00150 #endif 00151 #define __O volatile /*!< Defines 'write only' permissions */ 00152 #define __IO volatile /*!< Defines 'read / write' permissions */ 00153 00154 /*@} end of group Cortex_M0 */ 00155 00156 00157 00158 /******************************************************************************* 00159 * Register Abstraction 00160 Core Register contain: 00161 - Core Register 00162 - Core NVIC Register 00163 - Core SCB Register 00164 - Core SysTick Register 00165 ******************************************************************************/ 00166 /** \defgroup CMSIS_core_register Defines and Type Definitions 00167 \brief Type definitions and defines for Cortex-M processor based devices. 00168 */ 00169 00170 /** \ingroup CMSIS_core_register 00171 \defgroup CMSIS_CORE Status and Control Registers 00172 \brief Core Register type definitions. 00173 @{ 00174 */ 00175 00176 /** \brief Union type to access the Application Program Status Register (APSR). 00177 */ 00178 typedef union 00179 { 00180 struct 00181 { 00182 #if (__CORTEX_M != 0x04) 00183 uint32_t _reserved0:27; /*!< bit: 0..26 Reserved */ 00184 #else 00185 uint32_t _reserved0:16; /*!< bit: 0..15 Reserved */ 00186 uint32_t GE:4; /*!< bit: 16..19 Greater than or Equal flags */ 00187 uint32_t _reserved1:7; /*!< bit: 20..26 Reserved */ 00188 #endif 00189 uint32_t Q:1; /*!< bit: 27 Saturation condition flag */ 00190 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00191 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00192 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00193 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00194 } b; /*!< Structure used for bit access */ 00195 uint32_t w ; /*!< Type used for word access */ 00196 } APSR_Type; 00197 00198 00199 /** \brief Union type to access the Interrupt Program Status Register (IPSR). 00200 */ 00201 typedef union 00202 { 00203 struct 00204 { 00205 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00206 uint32_t _reserved0:23; /*!< bit: 9..31 Reserved */ 00207 } b; /*!< Structure used for bit access */ 00208 uint32_t w ; /*!< Type used for word access */ 00209 } IPSR_Type; 00210 00211 00212 /** \brief Union type to access the Special-Purpose Program Status Registers (xPSR). 00213 */ 00214 typedef union 00215 { 00216 struct 00217 { 00218 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00219 #if (__CORTEX_M != 0x04) 00220 uint32_t _reserved0:15; /*!< bit: 9..23 Reserved */ 00221 #else 00222 uint32_t _reserved0:7; /*!< bit: 9..15 Reserved */ 00223 uint32_t GE:4; /*!< bit: 16..19 Greater than or Equal flags */ 00224 uint32_t _reserved1:4; /*!< bit: 20..23 Reserved */ 00225 #endif 00226 uint32_t T:1; /*!< bit: 24 Thumb bit (read 0) */ 00227 uint32_t IT:2; /*!< bit: 25..26 saved IT state (read 0) */ 00228 uint32_t Q:1; /*!< bit: 27 Saturation condition flag */ 00229 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00230 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00231 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00232 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00233 } b; /*!< Structure used for bit access */ 00234 uint32_t w ; /*!< Type used for word access */ 00235 } xPSR_Type; 00236 00237 00238 /** \brief Union type to access the Control Registers (CONTROL). 00239 */ 00240 typedef union 00241 { 00242 struct 00243 { 00244 uint32_t nPRIV:1; /*!< bit: 0 Execution privilege in Thread mode */ 00245 uint32_t SPSEL:1; /*!< bit: 1 Stack to be used */ 00246 uint32_t FPCA:1; /*!< bit: 2 FP extension active flag */ 00247 uint32_t _reserved0:29; /*!< bit: 3..31 Reserved */ 00248 } b; /*!< Structure used for bit access */ 00249 uint32_t w ; /*!< Type used for word access */ 00250 } CONTROL_Type; 00251 00252 /*@} end of group CMSIS_CORE */ 00253 00254 00255 /** \ingroup CMSIS_core_register 00256 \defgroup CMSIS_NVIC Nested Vectored Interrupt Controller (NVIC) 00257 \brief Type definitions for the NVIC Registers 00258 @{ 00259 */ 00260 00261 /** \brief Structure type to access the Nested Vectored Interrupt Controller (NVIC). 00262 */ 00263 typedef struct 00264 { 00265 __IO uint32_t ISER[1]; /*!< Offset: 0x000 (R/W) Interrupt Set Enable Register */ 00266 uint32_t RESERVED0[31]; 00267 __IO uint32_t ICER[1]; /*!< Offset: 0x080 (R/W) Interrupt Clear Enable Register */ 00268 uint32_t RSERVED1[31]; 00269 __IO uint32_t ISPR[1]; /*!< Offset: 0x100 (R/W) Interrupt Set Pending Register */ 00270 uint32_t RESERVED2[31]; 00271 __IO uint32_t ICPR[1]; /*!< Offset: 0x180 (R/W) Interrupt Clear Pending Register */ 00272 uint32_t RESERVED3[31]; 00273 uint32_t RESERVED4[64]; 00274 __IO uint32_t IP[8]; /*!< Offset: 0x300 (R/W) Interrupt Priority Register */ 00275 } NVIC_Type; 00276 00277 /*@} end of group CMSIS_NVIC */ 00278 00279 00280 /** \ingroup CMSIS_core_register 00281 \defgroup CMSIS_SCB System Control Block (SCB) 00282 \brief Type definitions for the System Control Block Registers 00283 @{ 00284 */ 00285 00286 /** \brief Structure type to access the System Control Block (SCB). 00287 */ 00288 typedef struct 00289 { 00290 __I uint32_t CPUID ; /*!< Offset: 0x000 (R/ ) CPUID Base Register */ 00291 __IO uint32_t ICSR ; /*!< Offset: 0x004 (R/W) Interrupt Control and State Register */ 00292 uint32_t RESERVED0; 00293 __IO uint32_t AIRCR ; /*!< Offset: 0x00C (R/W) Application Interrupt and Reset Control Register */ 00294 __IO uint32_t SCR ; /*!< Offset: 0x010 (R/W) System Control Register */ 00295 __IO uint32_t CCR ; /*!< Offset: 0x014 (R/W) Configuration Control Register */ 00296 uint32_t RESERVED1; 00297 __IO uint32_t SHP[2]; /*!< Offset: 0x01C (R/W) System Handlers Priority Registers. [0] is RESERVED */ 00298 __IO uint32_t SHCSR ; /*!< Offset: 0x024 (R/W) System Handler Control and State Register */ 00299 } SCB_Type; 00300 00301 /* SCB CPUID Register Definitions */ 00302 #define SCB_CPUID_IMPLEMENTER_Pos 24 /*!< SCB CPUID: IMPLEMENTER Position */ 00303 #define SCB_CPUID_IMPLEMENTER_Msk (0xFFUL << SCB_CPUID_IMPLEMENTER_Pos) /*!< SCB CPUID: IMPLEMENTER Mask */ 00304 00305 #define SCB_CPUID_VARIANT_Pos 20 /*!< SCB CPUID: VARIANT Position */ 00306 #define SCB_CPUID_VARIANT_Msk (0xFUL << SCB_CPUID_VARIANT_Pos) /*!< SCB CPUID: VARIANT Mask */ 00307 00308 #define SCB_CPUID_ARCHITECTURE_Pos 16 /*!< SCB CPUID: ARCHITECTURE Position */ 00309 #define SCB_CPUID_ARCHITECTURE_Msk (0xFUL << SCB_CPUID_ARCHITECTURE_Pos) /*!< SCB CPUID: ARCHITECTURE Mask */ 00310 00311 #define SCB_CPUID_PARTNO_Pos 4 /*!< SCB CPUID: PARTNO Position */ 00312 #define SCB_CPUID_PARTNO_Msk (0xFFFUL << SCB_CPUID_PARTNO_Pos) /*!< SCB CPUID: PARTNO Mask */ 00313 00314 #define SCB_CPUID_REVISION_Pos 0 /*!< SCB CPUID: REVISION Position */ 00315 #define SCB_CPUID_REVISION_Msk (0xFUL << SCB_CPUID_REVISION_Pos) /*!< SCB CPUID: REVISION Mask */ 00316 00317 /* SCB Interrupt Control State Register Definitions */ 00318 #define SCB_ICSR_NMIPENDSET_Pos 31 /*!< SCB ICSR: NMIPENDSET Position */ 00319 #define SCB_ICSR_NMIPENDSET_Msk (1UL << SCB_ICSR_NMIPENDSET_Pos) /*!< SCB ICSR: NMIPENDSET Mask */ 00320 00321 #define SCB_ICSR_PENDSVSET_Pos 28 /*!< SCB ICSR: PENDSVSET Position */ 00322 #define SCB_ICSR_PENDSVSET_Msk (1UL << SCB_ICSR_PENDSVSET_Pos) /*!< SCB ICSR: PENDSVSET Mask */ 00323 00324 #define SCB_ICSR_PENDSVCLR_Pos 27 /*!< SCB ICSR: PENDSVCLR Position */ 00325 #define SCB_ICSR_PENDSVCLR_Msk (1UL << SCB_ICSR_PENDSVCLR_Pos) /*!< SCB ICSR: PENDSVCLR Mask */ 00326 00327 #define SCB_ICSR_PENDSTSET_Pos 26 /*!< SCB ICSR: PENDSTSET Position */ 00328 #define SCB_ICSR_PENDSTSET_Msk (1UL << SCB_ICSR_PENDSTSET_Pos) /*!< SCB ICSR: PENDSTSET Mask */ 00329 00330 #define SCB_ICSR_PENDSTCLR_Pos 25 /*!< SCB ICSR: PENDSTCLR Position */ 00331 #define SCB_ICSR_PENDSTCLR_Msk (1UL << SCB_ICSR_PENDSTCLR_Pos) /*!< SCB ICSR: PENDSTCLR Mask */ 00332 00333 #define SCB_ICSR_ISRPREEMPT_Pos 23 /*!< SCB ICSR: ISRPREEMPT Position */ 00334 #define SCB_ICSR_ISRPREEMPT_Msk (1UL << SCB_ICSR_ISRPREEMPT_Pos) /*!< SCB ICSR: ISRPREEMPT Mask */ 00335 00336 #define SCB_ICSR_ISRPENDING_Pos 22 /*!< SCB ICSR: ISRPENDING Position */ 00337 #define SCB_ICSR_ISRPENDING_Msk (1UL << SCB_ICSR_ISRPENDING_Pos) /*!< SCB ICSR: ISRPENDING Mask */ 00338 00339 #define SCB_ICSR_VECTPENDING_Pos 12 /*!< SCB ICSR: VECTPENDING Position */ 00340 #define SCB_ICSR_VECTPENDING_Msk (0x1FFUL << SCB_ICSR_VECTPENDING_Pos) /*!< SCB ICSR: VECTPENDING Mask */ 00341 00342 #define SCB_ICSR_VECTACTIVE_Pos 0 /*!< SCB ICSR: VECTACTIVE Position */ 00343 #define SCB_ICSR_VECTACTIVE_Msk (0x1FFUL << SCB_ICSR_VECTACTIVE_Pos) /*!< SCB ICSR: VECTACTIVE Mask */ 00344 00345 /* SCB Application Interrupt and Reset Control Register Definitions */ 00346 #define SCB_AIRCR_VECTKEY_Pos 16 /*!< SCB AIRCR: VECTKEY Position */ 00347 #define SCB_AIRCR_VECTKEY_Msk (0xFFFFUL << SCB_AIRCR_VECTKEY_Pos) /*!< SCB AIRCR: VECTKEY Mask */ 00348 00349 #define SCB_AIRCR_VECTKEYSTAT_Pos 16 /*!< SCB AIRCR: VECTKEYSTAT Position */ 00350 #define SCB_AIRCR_VECTKEYSTAT_Msk (0xFFFFUL << SCB_AIRCR_VECTKEYSTAT_Pos) /*!< SCB AIRCR: VECTKEYSTAT Mask */ 00351 00352 #define SCB_AIRCR_ENDIANESS_Pos 15 /*!< SCB AIRCR: ENDIANESS Position */ 00353 #define SCB_AIRCR_ENDIANESS_Msk (1UL << SCB_AIRCR_ENDIANESS_Pos) /*!< SCB AIRCR: ENDIANESS Mask */ 00354 00355 #define SCB_AIRCR_SYSRESETREQ_Pos 2 /*!< SCB AIRCR: SYSRESETREQ Position */ 00356 #define SCB_AIRCR_SYSRESETREQ_Msk (1UL << SCB_AIRCR_SYSRESETREQ_Pos) /*!< SCB AIRCR: SYSRESETREQ Mask */ 00357 00358 #define SCB_AIRCR_VECTCLRACTIVE_Pos 1 /*!< SCB AIRCR: VECTCLRACTIVE Position */ 00359 #define SCB_AIRCR_VECTCLRACTIVE_Msk (1UL << SCB_AIRCR_VECTCLRACTIVE_Pos) /*!< SCB AIRCR: VECTCLRACTIVE Mask */ 00360 00361 /* SCB System Control Register Definitions */ 00362 #define SCB_SCR_SEVONPEND_Pos 4 /*!< SCB SCR: SEVONPEND Position */ 00363 #define SCB_SCR_SEVONPEND_Msk (1UL << SCB_SCR_SEVONPEND_Pos) /*!< SCB SCR: SEVONPEND Mask */ 00364 00365 #define SCB_SCR_SLEEPDEEP_Pos 2 /*!< SCB SCR: SLEEPDEEP Position */ 00366 #define SCB_SCR_SLEEPDEEP_Msk (1UL << SCB_SCR_SLEEPDEEP_Pos) /*!< SCB SCR: SLEEPDEEP Mask */ 00367 00368 #define SCB_SCR_SLEEPONEXIT_Pos 1 /*!< SCB SCR: SLEEPONEXIT Position */ 00369 #define SCB_SCR_SLEEPONEXIT_Msk (1UL << SCB_SCR_SLEEPONEXIT_Pos) /*!< SCB SCR: SLEEPONEXIT Mask */ 00370 00371 /* SCB Configuration Control Register Definitions */ 00372 #define SCB_CCR_STKALIGN_Pos 9 /*!< SCB CCR: STKALIGN Position */ 00373 #define SCB_CCR_STKALIGN_Msk (1UL << SCB_CCR_STKALIGN_Pos) /*!< SCB CCR: STKALIGN Mask */ 00374 00375 #define SCB_CCR_UNALIGN_TRP_Pos 3 /*!< SCB CCR: UNALIGN_TRP Position */ 00376 #define SCB_CCR_UNALIGN_TRP_Msk (1UL << SCB_CCR_UNALIGN_TRP_Pos) /*!< SCB CCR: UNALIGN_TRP Mask */ 00377 00378 /* SCB System Handler Control and State Register Definitions */ 00379 #define SCB_SHCSR_SVCALLPENDED_Pos 15 /*!< SCB SHCSR: SVCALLPENDED Position */ 00380 #define SCB_SHCSR_SVCALLPENDED_Msk (1UL << SCB_SHCSR_SVCALLPENDED_Pos) /*!< SCB SHCSR: SVCALLPENDED Mask */ 00381 00382 /*@} end of group CMSIS_SCB */ 00383 00384 00385 /** \ingroup CMSIS_core_register 00386 \defgroup CMSIS_SysTick System Tick Timer (SysTick) 00387 \brief Type definitions for the System Timer Registers. 00388 @{ 00389 */ 00390 00391 /** \brief Structure type to access the System Timer (SysTick). 00392 */ 00393 typedef struct 00394 { 00395 __IO uint32_t CTRL ; /*!< Offset: 0x000 (R/W) SysTick Control and Status Register */ 00396 __IO uint32_t LOAD ; /*!< Offset: 0x004 (R/W) SysTick Reload Value Register */ 00397 __IO uint32_t VAL ; /*!< Offset: 0x008 (R/W) SysTick Current Value Register */ 00398 __I uint32_t CALIB ; /*!< Offset: 0x00C (R/ ) SysTick Calibration Register */ 00399 } SysTick_Type; 00400 00401 /* SysTick Control / Status Register Definitions */ 00402 #define SysTick_CTRL_COUNTFLAG_Pos 16 /*!< SysTick CTRL: COUNTFLAG Position */ 00403 #define SysTick_CTRL_COUNTFLAG_Msk (1UL << SysTick_CTRL_COUNTFLAG_Pos) /*!< SysTick CTRL: COUNTFLAG Mask */ 00404 00405 #define SysTick_CTRL_CLKSOURCE_Pos 2 /*!< SysTick CTRL: CLKSOURCE Position */ 00406 #define SysTick_CTRL_CLKSOURCE_Msk (1UL << SysTick_CTRL_CLKSOURCE_Pos) /*!< SysTick CTRL: CLKSOURCE Mask */ 00407 00408 #define SysTick_CTRL_TICKINT_Pos 1 /*!< SysTick CTRL: TICKINT Position */ 00409 #define SysTick_CTRL_TICKINT_Msk (1UL << SysTick_CTRL_TICKINT_Pos) /*!< SysTick CTRL: TICKINT Mask */ 00410 00411 #define SysTick_CTRL_ENABLE_Pos 0 /*!< SysTick CTRL: ENABLE Position */ 00412 #define SysTick_CTRL_ENABLE_Msk (1UL << SysTick_CTRL_ENABLE_Pos) /*!< SysTick CTRL: ENABLE Mask */ 00413 00414 /* SysTick Reload Register Definitions */ 00415 #define SysTick_LOAD_RELOAD_Pos 0 /*!< SysTick LOAD: RELOAD Position */ 00416 #define SysTick_LOAD_RELOAD_Msk (0xFFFFFFUL << SysTick_LOAD_RELOAD_Pos) /*!< SysTick LOAD: RELOAD Mask */ 00417 00418 /* SysTick Current Register Definitions */ 00419 #define SysTick_VAL_CURRENT_Pos 0 /*!< SysTick VAL: CURRENT Position */ 00420 #define SysTick_VAL_CURRENT_Msk (0xFFFFFFUL << SysTick_VAL_CURRENT_Pos) /*!< SysTick VAL: CURRENT Mask */ 00421 00422 /* SysTick Calibration Register Definitions */ 00423 #define SysTick_CALIB_NOREF_Pos 31 /*!< SysTick CALIB: NOREF Position */ 00424 #define SysTick_CALIB_NOREF_Msk (1UL << SysTick_CALIB_NOREF_Pos) /*!< SysTick CALIB: NOREF Mask */ 00425 00426 #define SysTick_CALIB_SKEW_Pos 30 /*!< SysTick CALIB: SKEW Position */ 00427 #define SysTick_CALIB_SKEW_Msk (1UL << SysTick_CALIB_SKEW_Pos) /*!< SysTick CALIB: SKEW Mask */ 00428 00429 #define SysTick_CALIB_TENMS_Pos 0 /*!< SysTick CALIB: TENMS Position */ 00430 #define SysTick_CALIB_TENMS_Msk (0xFFFFFFUL << SysTick_VAL_CURRENT_Pos) /*!< SysTick CALIB: TENMS Mask */ 00431 00432 /*@} end of group CMSIS_SysTick */ 00433 00434 00435 /** \ingroup CMSIS_core_register 00436 \defgroup CMSIS_CoreDebug Core Debug Registers (CoreDebug) 00437 \brief Cortex-M0 Core Debug Registers (DCB registers, SHCSR, and DFSR) 00438 are only accessible over DAP and not via processor. Therefore 00439 they are not covered by the Cortex-M0 header file. 00440 @{ 00441 */ 00442 /*@} end of group CMSIS_CoreDebug */ 00443 00444 00445 /** \ingroup CMSIS_core_register 00446 \defgroup CMSIS_core_base Core Definitions 00447 \brief Definitions for base addresses, unions, and structures. 00448 @{ 00449 */ 00450 00451 /* Memory mapping of Cortex-M0 Hardware */ 00452 #define SCS_BASE (0xE000E000UL) /*!< System Control Space Base Address */ 00453 #define SysTick_BASE (SCS_BASE + 0x0010UL) /*!< SysTick Base Address */ 00454 #define NVIC_BASE (SCS_BASE + 0x0100UL) /*!< NVIC Base Address */ 00455 #define SCB_BASE (SCS_BASE + 0x0D00UL) /*!< System Control Block Base Address */ 00456 00457 #define SCB ((SCB_Type *) SCB_BASE ) /*!< SCB configuration struct */ 00458 #define SysTick ((SysTick_Type *) SysTick_BASE ) /*!< SysTick configuration struct */ 00459 #define NVIC ((NVIC_Type *) NVIC_BASE ) /*!< NVIC configuration struct */ 00460 00461 00462 /*@} */ 00463 00464 00465 00466 /******************************************************************************* 00467 * Hardware Abstraction Layer 00468 Core Function Interface contains: 00469 - Core NVIC Functions 00470 - Core SysTick Functions 00471 - Core Register Access Functions 00472 ******************************************************************************/ 00473 /** \defgroup CMSIS_Core_FunctionInterface Functions and Instructions Reference 00474 */ 00475 00476 00477 00478 /* ########################## NVIC functions #################################### */ 00479 /** \ingroup CMSIS_Core_FunctionInterface 00480 \defgroup CMSIS_Core_NVICFunctions NVIC Functions 00481 \brief Functions that manage interrupts and exceptions via the NVIC. 00482 @{ 00483 */ 00484 00485 /* Interrupt Priorities are WORD accessible only under ARMv6M */ 00486 /* The following MACROS handle generation of the register offset and byte masks */ 00487 #define _BIT_SHIFT(IRQn) ( (((uint32_t)(IRQn) ) & 0x03) * 8 ) 00488 #define _SHP_IDX(IRQn) ( ((((uint32_t)(IRQn) & 0x0F)-8) >> 2) ) 00489 #define _IP_IDX(IRQn) ( ((uint32_t)(IRQn) >> 2) ) 00490 00491 00492 /** \brief Enable External Interrupt 00493 00494 The function enables a device-specific interrupt in the NVIC interrupt controller. 00495 00496 \param [in] IRQn External interrupt number. Value cannot be negative. 00497 */ 00498 __STATIC_INLINE void NVIC_EnableIRQ(IRQn_Type IRQn ) 00499 { 00500 NVIC->ISER[0] = (1 << ((uint32_t)(IRQn) & 0x1F)); 00501 } 00502 00503 00504 /** \brief Disable External Interrupt 00505 00506 The function disables a device-specific interrupt in the NVIC interrupt controller. 00507 00508 \param [in] IRQn External interrupt number. Value cannot be negative. 00509 */ 00510 __STATIC_INLINE void NVIC_DisableIRQ(IRQn_Type IRQn ) 00511 { 00512 NVIC->ICER[0] = (1 << ((uint32_t)(IRQn) & 0x1F)); 00513 } 00514 00515 00516 /** \brief Get Pending Interrupt 00517 00518 The function reads the pending register in the NVIC and returns the pending bit 00519 for the specified interrupt. 00520 00521 \param [in] IRQn Interrupt number. 00522 00523 \return 0 Interrupt status is not pending. 00524 \return 1 Interrupt status is pending. 00525 */ 00526 __STATIC_INLINE uint32_t NVIC_GetPendingIRQ(IRQn_Type IRQn ) 00527 { 00528 return((uint32_t) ((NVIC->ISPR[0] & (1 << ((uint32_t)(IRQn) & 0x1F)))?1:0)); 00529 } 00530 00531 00532 /** \brief Set Pending Interrupt 00533 00534 The function sets the pending bit of an external interrupt. 00535 00536 \param [in] IRQn Interrupt number. Value cannot be negative. 00537 */ 00538 __STATIC_INLINE void NVIC_SetPendingIRQ(IRQn_Type IRQn ) 00539 { 00540 NVIC->ISPR[0] = (1 << ((uint32_t)(IRQn) & 0x1F)); 00541 } 00542 00543 00544 /** \brief Clear Pending Interrupt 00545 00546 The function clears the pending bit of an external interrupt. 00547 00548 \param [in] IRQn External interrupt number. Value cannot be negative. 00549 */ 00550 __STATIC_INLINE void NVIC_ClearPendingIRQ(IRQn_Type IRQn ) 00551 { 00552 NVIC->ICPR[0] = (1 << ((uint32_t)(IRQn) & 0x1F)); /* Clear pending interrupt */ 00553 } 00554 00555 00556 /** \brief Set Interrupt Priority 00557 00558 The function sets the priority of an interrupt. 00559 00560 \note The priority cannot be set for every core interrupt. 00561 00562 \param [in] IRQn Interrupt number. 00563 \param [in] priority Priority to set. 00564 */ 00565 __STATIC_INLINE void NVIC_SetPriority(IRQn_Type IRQn , uint32_t priority) 00566 { 00567 if(IRQn < 0) { 00568 SCB->SHP[_SHP_IDX(IRQn)] = (SCB->SHP[_SHP_IDX(IRQn)] & ~(0xFF << _BIT_SHIFT(IRQn))) | 00569 (((priority << (8 - __NVIC_PRIO_BITS)) & 0xFF) << _BIT_SHIFT(IRQn)); } 00570 else { 00571 NVIC->IP[_IP_IDX(IRQn)] = (NVIC->IP[_IP_IDX(IRQn)] & ~(0xFF << _BIT_SHIFT(IRQn))) | 00572 (((priority << (8 - __NVIC_PRIO_BITS)) & 0xFF) << _BIT_SHIFT(IRQn)); } 00573 } 00574 00575 00576 /** \brief Get Interrupt Priority 00577 00578 The function reads the priority of an interrupt. The interrupt 00579 number can be positive to specify an external (device specific) 00580 interrupt, or negative to specify an internal (core) interrupt. 00581 00582 00583 \param [in] IRQn Interrupt number. 00584 \return Interrupt Priority. Value is aligned automatically to the implemented 00585 priority bits of the microcontroller. 00586 */ 00587 __STATIC_INLINE uint32_t NVIC_GetPriority(IRQn_Type IRQn ) 00588 { 00589 00590 if(IRQn < 0) { 00591 return((uint32_t)((SCB->SHP[_SHP_IDX(IRQn)] >> _BIT_SHIFT(IRQn) ) >> (8 - __NVIC_PRIO_BITS))); } /* get priority for Cortex-M0 system interrupts */ 00592 else { 00593 return((uint32_t)((NVIC->IP[ _IP_IDX(IRQn)] >> _BIT_SHIFT(IRQn) ) >> (8 - __NVIC_PRIO_BITS))); } /* get priority for device specific interrupts */ 00594 } 00595 00596 00597 /** \brief System Reset 00598 00599 The function initiates a system reset request to reset the MCU. 00600 */ 00601 __STATIC_INLINE void NVIC_SystemReset(void) 00602 { 00603 __DSB(); /* Ensure all outstanding memory accesses included 00604 buffered write are completed before reset */ 00605 SCB->AIRCR = ((0x5FA << SCB_AIRCR_VECTKEY_Pos) | 00606 SCB_AIRCR_SYSRESETREQ_Msk); 00607 __DSB(); /* Ensure completion of memory access */ 00608 while(1); /* wait until reset */ 00609 } 00610 00611 /*@} end of CMSIS_Core_NVICFunctions */ 00612 00613 00614 00615 /* ################################## SysTick function ############################################ */ 00616 /** \ingroup CMSIS_Core_FunctionInterface 00617 \defgroup CMSIS_Core_SysTickFunctions SysTick Functions 00618 \brief Functions that configure the System. 00619 @{ 00620 */ 00621 00622 #if (__Vendor_SysTickConfig == 0) 00623 00624 /** \brief System Tick Configuration 00625 00626 The function initializes the System Timer and its interrupt, and starts the System Tick Timer. 00627 Counter is in free running mode to generate periodic interrupts. 00628 00629 \param [in] ticks Number of ticks between two interrupts. 00630 00631 \return 0 Function succeeded. 00632 \return 1 Function failed. 00633 00634 \note When the variable <b>__Vendor_SysTickConfig</b> is set to 1, then the 00635 function <b>SysTick_Config</b> is not included. In this case, the file <b><i>device</i>.h</b> 00636 must contain a vendor-specific implementation of this function. 00637 00638 */ 00639 __STATIC_INLINE uint32_t SysTick_Config(uint32_t ticks) 00640 { 00641 if (ticks > SysTick_LOAD_RELOAD_Msk) return (1); /* Reload value impossible */ 00642 00643 SysTick->LOAD = (ticks & SysTick_LOAD_RELOAD_Msk) - 1; /* set reload register */ 00644 NVIC_SetPriority (SysTick_IRQn , (1<<__NVIC_PRIO_BITS) - 1); /* set Priority for Systick Interrupt */ 00645 SysTick->VAL = 0; /* Load the SysTick Counter Value */ 00646 SysTick->CTRL = SysTick_CTRL_CLKSOURCE_Msk | 00647 SysTick_CTRL_TICKINT_Msk | 00648 SysTick_CTRL_ENABLE_Msk; /* Enable SysTick IRQ and SysTick Timer */ 00649 return (0); /* Function successful */ 00650 } 00651 00652 #endif 00653 00654 /*@} end of CMSIS_Core_SysTickFunctions */ 00655 00656 00657 00658 00659 #endif /* __CORE_CM0_H_DEPENDANT */ 00660 00661 #endif /* __CMSIS_GENERIC */ 00662 00663 #ifdef __cplusplus 00664 } 00665 #endif
Generated on Tue Jul 12 2022 11:27:26 by
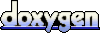