Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
SerialHalfDuplex.h
00001 /* mbed Microcontroller Library - SerialHalfDuplex 00002 * Copyright (c) 2010-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_SERIALHALFDUPLEX_H 00006 #define MBED_SERIALHALFDUPLEX_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_SERIAL 00011 00012 #include "Serial.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 00016 namespace mbed { 00017 00018 /** A serial port (UART) for communication with other devices using 00019 * Half-Duplex, allowing transmit and receive on a single 00020 * shared transmit and receive line. Only one end should be transmitting 00021 * at a time. 00022 * 00023 * Both the tx and rx pin should be defined, and wired together. 00024 * This is in addition to them being wired to the other serial 00025 * device to allow both read and write functions to operate. 00026 * 00027 * For Simplex and Full-Duplex Serial communication, see Serial() 00028 * 00029 * Example: 00030 * @code 00031 * // Send a byte to a second HalfDuplex device, and read the response 00032 * 00033 * #include "mbed.h" 00034 * 00035 * // p9 and p10 should be wired together to form "a" 00036 * // p28 and p27 should be wired together to form "b" 00037 * // p9/p10 should be wired to p28/p27 as the Half Duplex connection 00038 * 00039 * SerialHalfDuplex a(p9, p10); 00040 * SerialHalfDuplex b(p28, p27); 00041 * 00042 * void b_rx() { // second device response 00043 * b.putc(b.getc() + 4); 00044 * } 00045 * 00046 * int main() { 00047 * b.attach(&b_rx); 00048 * for (int c = 'A'; c < 'Z'; c++) { 00049 * a.putc(c); 00050 * printf("sent [%c]\n", c); 00051 * wait(0.5); // b should respond 00052 * if (a.readable()) { 00053 * printf("received [%c]\n", a.getc()); 00054 * } 00055 * } 00056 * } 00057 * @endcode 00058 */ 00059 class SerialHalfDuplex : public Serial { 00060 00061 public: 00062 /** Create a half-duplex serial port, connected to the specified transmit 00063 * and receive pins. 00064 * 00065 * These pins should be wired together, as well as to the target device 00066 * 00067 * @param tx Transmit pin 00068 * @param rx Receive pin 00069 */ 00070 SerialHalfDuplex(PinName tx, PinName rx, const char *name = NULL); 00071 00072 #if 0 // Inherited from Serial class, for documentation 00073 /** Set the baud rate of the serial port 00074 * 00075 * @param baudrate The baudrate of the serial port (default = 9600). 00076 */ 00077 void baud(int baudrate); 00078 00079 enum Parity { 00080 None = 0 00081 , Odd 00082 , Even 00083 , Forced1 00084 , Forced0 00085 }; 00086 00087 /** Set the transmission format used by the Serial port 00088 * 00089 * @param bits The number of bits in a word (5-8; default = 8) 00090 * @param parity The parity used (Serial::None, Serial::Odd, 00091 * Serial::Even, Serial::Forced1, Serial::Forced0; default = Serial::None) 00092 * @param stop The number of stop bits (1 or 2; default = 1) 00093 */ 00094 void format(int bits = 8, Parity parity = Serial::None, int stop_bits 00095 = 1); 00096 00097 /** Write a character 00098 * 00099 * @param c The character to write to the serial port 00100 */ 00101 int putc(int c); 00102 00103 /** Read a character 00104 * 00105 * Read a character from the serial port. This call will block 00106 * until a character is available. For testing if a character is 00107 * available for reading, see <readable>. 00108 * 00109 * @returns 00110 * The character read from the serial port 00111 */ 00112 int getc(); 00113 00114 /** Write a formated string 00115 * 00116 * @param format A printf-style format string, followed by the 00117 * variables to use in formating the string. 00118 */ 00119 int printf(const char* format, ...); 00120 00121 /** Read a formated string 00122 * 00123 * @param format A scanf-style format string, 00124 * followed by the pointers to variables to store the results. 00125 */ 00126 int scanf(const char* format, ...); 00127 00128 /** Determine if there is a character available to read 00129 * 00130 * @returns 00131 * 1 if there is a character available to read, 00132 * 0 otherwise 00133 */ 00134 int readable(); 00135 00136 /** Determine if there is space available to write a character 00137 * 00138 * @returns 00139 * 1 if there is space to write a character, 00140 * 0 otherwise 00141 */ 00142 int writeable(); 00143 00144 /** Attach a function to call whenever a serial interrupt is generated 00145 * 00146 * @param fptr A pointer to a void function, or 0 to set as none 00147 */ 00148 void attach(void (*fptr)(void)); 00149 00150 /** Attach a member function to call whenever a serial interrupt is generated 00151 * 00152 * @param tptr pointer to the object to call the member function on 00153 * @param mptr pointer to the member function to be called 00154 */ 00155 template<typename T> 00156 void attach(T* tptr, void (T::*mptr)(void)); 00157 00158 #endif 00159 00160 protected: 00161 PinName _txpin; 00162 00163 virtual int _putc(int c); 00164 virtual int _getc(void); 00165 00166 }; // End class SerialHalfDuplex 00167 00168 } // End namespace 00169 00170 #endif 00171 00172 #endif
Generated on Tue Jul 12 2022 11:27:27 by
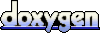