Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
LPC17xx.h
00001 /**************************************************************************//** 00002 * @file LPC17xx.h 00003 * @brief CMSIS Cortex-M3 Core Peripheral Access Layer Header File for 00004 * NXP LPC17xx Device Series 00005 * @version: V1.09 00006 * @date: 17. March 2010 00007 00008 * 00009 * @note 00010 * Copyright (C) 2009 ARM Limited. All rights reserved. 00011 * 00012 * @par 00013 * ARM Limited (ARM) is supplying this software for use with Cortex-M 00014 * processor based microcontrollers. This file can be freely distributed 00015 * within development tools that are supporting such ARM based processors. 00016 * 00017 * @par 00018 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00019 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00021 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00022 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00023 * 00024 ******************************************************************************/ 00025 00026 00027 #ifndef __LPC17xx_H__ 00028 #define __LPC17xx_H__ 00029 00030 /* 00031 * ========================================================================== 00032 * ---------- Interrupt Number Definition ----------------------------------- 00033 * ========================================================================== 00034 */ 00035 00036 typedef enum IRQn 00037 { 00038 /****** Cortex-M3 Processor Exceptions Numbers ***************************************************/ 00039 NonMaskableInt_IRQn = -14, /*!< 2 Non Maskable Interrupt */ 00040 MemoryManagement_IRQn = -12, /*!< 4 Cortex-M3 Memory Management Interrupt */ 00041 BusFault_IRQn = -11, /*!< 5 Cortex-M3 Bus Fault Interrupt */ 00042 UsageFault_IRQn = -10, /*!< 6 Cortex-M3 Usage Fault Interrupt */ 00043 SVCall_IRQn = -5, /*!< 11 Cortex-M3 SV Call Interrupt */ 00044 DebugMonitor_IRQn = -4, /*!< 12 Cortex-M3 Debug Monitor Interrupt */ 00045 PendSV_IRQn = -2, /*!< 14 Cortex-M3 Pend SV Interrupt */ 00046 SysTick_IRQn = -1, /*!< 15 Cortex-M3 System Tick Interrupt */ 00047 00048 /****** LPC17xx Specific Interrupt Numbers *******************************************************/ 00049 WDT_IRQn = 0, /*!< Watchdog Timer Interrupt */ 00050 TIMER0_IRQn = 1, /*!< Timer0 Interrupt */ 00051 TIMER1_IRQn = 2, /*!< Timer1 Interrupt */ 00052 TIMER2_IRQn = 3, /*!< Timer2 Interrupt */ 00053 TIMER3_IRQn = 4, /*!< Timer3 Interrupt */ 00054 UART0_IRQn = 5, /*!< UART0 Interrupt */ 00055 UART1_IRQn = 6, /*!< UART1 Interrupt */ 00056 UART2_IRQn = 7, /*!< UART2 Interrupt */ 00057 UART3_IRQn = 8, /*!< UART3 Interrupt */ 00058 PWM1_IRQn = 9, /*!< PWM1 Interrupt */ 00059 I2C0_IRQn = 10, /*!< I2C0 Interrupt */ 00060 I2C1_IRQn = 11, /*!< I2C1 Interrupt */ 00061 I2C2_IRQn = 12, /*!< I2C2 Interrupt */ 00062 SPI_IRQn = 13, /*!< SPI Interrupt */ 00063 SSP0_IRQn = 14, /*!< SSP0 Interrupt */ 00064 SSP1_IRQn = 15, /*!< SSP1 Interrupt */ 00065 PLL0_IRQn = 16, /*!< PLL0 Lock (Main PLL) Interrupt */ 00066 RTC_IRQn = 17, /*!< Real Time Clock Interrupt */ 00067 EINT0_IRQn = 18, /*!< External Interrupt 0 Interrupt */ 00068 EINT1_IRQn = 19, /*!< External Interrupt 1 Interrupt */ 00069 EINT2_IRQn = 20, /*!< External Interrupt 2 Interrupt */ 00070 EINT3_IRQn = 21, /*!< External Interrupt 3 Interrupt */ 00071 ADC_IRQn = 22, /*!< A/D Converter Interrupt */ 00072 BOD_IRQn = 23, /*!< Brown-Out Detect Interrupt */ 00073 USB_IRQn = 24, /*!< USB Interrupt */ 00074 CAN_IRQn = 25, /*!< CAN Interrupt */ 00075 DMA_IRQn = 26, /*!< General Purpose DMA Interrupt */ 00076 I2S_IRQn = 27, /*!< I2S Interrupt */ 00077 ENET_IRQn = 28, /*!< Ethernet Interrupt */ 00078 RIT_IRQn = 29, /*!< Repetitive Interrupt Timer Interrupt */ 00079 MCPWM_IRQn = 30, /*!< Motor Control PWM Interrupt */ 00080 QEI_IRQn = 31, /*!< Quadrature Encoder Interface Interrupt */ 00081 PLL1_IRQn = 32, /*!< PLL1 Lock (USB PLL) Interrupt */ 00082 USBActivity_IRQn = 33, /* USB Activity interrupt */ 00083 CANActivity_IRQn = 34, /* CAN Activity interrupt */ 00084 } IRQn_Type ; 00085 00086 00087 /* 00088 * ========================================================================== 00089 * ----------- Processor and Core Peripheral Section ------------------------ 00090 * ========================================================================== 00091 */ 00092 00093 /* Configuration of the Cortex-M3 Processor and Core Peripherals */ 00094 #define __MPU_PRESENT 1 /*!< MPU present or not */ 00095 #define __NVIC_PRIO_BITS 5 /*!< Number of Bits used for Priority Levels */ 00096 #define __Vendor_SysTickConfig 0 /*!< Set to 1 if different SysTick Config is used */ 00097 00098 00099 #include "core_cm3.h" /* Cortex-M3 processor and core peripherals */ 00100 #include "system_LPC17xx.h" /* System Header */ 00101 00102 00103 /******************************************************************************/ 00104 /* Device Specific Peripheral registers structures */ 00105 /******************************************************************************/ 00106 00107 #if defined ( __CC_ARM ) 00108 #pragma anon_unions 00109 #endif 00110 00111 /*------------- System Control (SC) ------------------------------------------*/ 00112 typedef struct 00113 { 00114 __IO uint32_t FLASHCFG; /* Flash Accelerator Module */ 00115 uint32_t RESERVED0[31]; 00116 __IO uint32_t PLL0CON; /* Clocking and Power Control */ 00117 __IO uint32_t PLL0CFG; 00118 __I uint32_t PLL0STAT; 00119 __O uint32_t PLL0FEED; 00120 uint32_t RESERVED1[4]; 00121 __IO uint32_t PLL1CON; 00122 __IO uint32_t PLL1CFG; 00123 __I uint32_t PLL1STAT; 00124 __O uint32_t PLL1FEED; 00125 uint32_t RESERVED2[4]; 00126 __IO uint32_t PCON; 00127 __IO uint32_t PCONP; 00128 uint32_t RESERVED3[15]; 00129 __IO uint32_t CCLKCFG; 00130 __IO uint32_t USBCLKCFG; 00131 __IO uint32_t CLKSRCSEL; 00132 __IO uint32_t CANSLEEPCLR; 00133 __IO uint32_t CANWAKEFLAGS; 00134 uint32_t RESERVED4[10]; 00135 __IO uint32_t EXTINT; /* External Interrupts */ 00136 uint32_t RESERVED5; 00137 __IO uint32_t EXTMODE; 00138 __IO uint32_t EXTPOLAR; 00139 uint32_t RESERVED6[12]; 00140 __IO uint32_t RSID; /* Reset */ 00141 uint32_t RESERVED7[7]; 00142 __IO uint32_t SCS; /* Syscon Miscellaneous Registers */ 00143 __IO uint32_t IRCTRIM; /* Clock Dividers */ 00144 __IO uint32_t PCLKSEL0; 00145 __IO uint32_t PCLKSEL1; 00146 uint32_t RESERVED8[4]; 00147 __IO uint32_t USBIntSt; /* USB Device/OTG Interrupt Register */ 00148 __IO uint32_t DMAREQSEL; 00149 __IO uint32_t CLKOUTCFG; /* Clock Output Configuration */ 00150 } LPC_SC_TypeDef; 00151 00152 /*------------- Pin Connect Block (PINCON) -----------------------------------*/ 00153 typedef struct 00154 { 00155 __IO uint32_t PINSEL0; 00156 __IO uint32_t PINSEL1; 00157 __IO uint32_t PINSEL2; 00158 __IO uint32_t PINSEL3; 00159 __IO uint32_t PINSEL4; 00160 __IO uint32_t PINSEL5; 00161 __IO uint32_t PINSEL6; 00162 __IO uint32_t PINSEL7; 00163 __IO uint32_t PINSEL8; 00164 __IO uint32_t PINSEL9; 00165 __IO uint32_t PINSEL10; 00166 uint32_t RESERVED0[5]; 00167 __IO uint32_t PINMODE0; 00168 __IO uint32_t PINMODE1; 00169 __IO uint32_t PINMODE2; 00170 __IO uint32_t PINMODE3; 00171 __IO uint32_t PINMODE4; 00172 __IO uint32_t PINMODE5; 00173 __IO uint32_t PINMODE6; 00174 __IO uint32_t PINMODE7; 00175 __IO uint32_t PINMODE8; 00176 __IO uint32_t PINMODE9; 00177 __IO uint32_t PINMODE_OD0; 00178 __IO uint32_t PINMODE_OD1; 00179 __IO uint32_t PINMODE_OD2; 00180 __IO uint32_t PINMODE_OD3; 00181 __IO uint32_t PINMODE_OD4; 00182 __IO uint32_t I2CPADCFG; 00183 } LPC_PINCON_TypeDef; 00184 00185 /*------------- General Purpose Input/Output (GPIO) --------------------------*/ 00186 typedef struct 00187 { 00188 union { 00189 __IO uint32_t FIODIR; 00190 struct { 00191 __IO uint16_t FIODIRL; 00192 __IO uint16_t FIODIRH; 00193 }; 00194 struct { 00195 __IO uint8_t FIODIR0; 00196 __IO uint8_t FIODIR1; 00197 __IO uint8_t FIODIR2; 00198 __IO uint8_t FIODIR3; 00199 }; 00200 }; 00201 uint32_t RESERVED0[3]; 00202 union { 00203 __IO uint32_t FIOMASK; 00204 struct { 00205 __IO uint16_t FIOMASKL; 00206 __IO uint16_t FIOMASKH; 00207 }; 00208 struct { 00209 __IO uint8_t FIOMASK0; 00210 __IO uint8_t FIOMASK1; 00211 __IO uint8_t FIOMASK2; 00212 __IO uint8_t FIOMASK3; 00213 }; 00214 }; 00215 union { 00216 __IO uint32_t FIOPIN; 00217 struct { 00218 __IO uint16_t FIOPINL; 00219 __IO uint16_t FIOPINH; 00220 }; 00221 struct { 00222 __IO uint8_t FIOPIN0; 00223 __IO uint8_t FIOPIN1; 00224 __IO uint8_t FIOPIN2; 00225 __IO uint8_t FIOPIN3; 00226 }; 00227 }; 00228 union { 00229 __IO uint32_t FIOSET; 00230 struct { 00231 __IO uint16_t FIOSETL; 00232 __IO uint16_t FIOSETH; 00233 }; 00234 struct { 00235 __IO uint8_t FIOSET0; 00236 __IO uint8_t FIOSET1; 00237 __IO uint8_t FIOSET2; 00238 __IO uint8_t FIOSET3; 00239 }; 00240 }; 00241 union { 00242 __O uint32_t FIOCLR; 00243 struct { 00244 __O uint16_t FIOCLRL; 00245 __O uint16_t FIOCLRH; 00246 }; 00247 struct { 00248 __O uint8_t FIOCLR0; 00249 __O uint8_t FIOCLR1; 00250 __O uint8_t FIOCLR2; 00251 __O uint8_t FIOCLR3; 00252 }; 00253 }; 00254 } LPC_GPIO_TypeDef; 00255 00256 typedef struct 00257 { 00258 __I uint32_t IntStatus; 00259 __I uint32_t IO0IntStatR; 00260 __I uint32_t IO0IntStatF; 00261 __O uint32_t IO0IntClr; 00262 __IO uint32_t IO0IntEnR; 00263 __IO uint32_t IO0IntEnF; 00264 uint32_t RESERVED0[3]; 00265 __I uint32_t IO2IntStatR; 00266 __I uint32_t IO2IntStatF; 00267 __O uint32_t IO2IntClr; 00268 __IO uint32_t IO2IntEnR; 00269 __IO uint32_t IO2IntEnF; 00270 } LPC_GPIOINT_TypeDef; 00271 00272 /*------------- Timer (TIM) --------------------------------------------------*/ 00273 typedef struct 00274 { 00275 __IO uint32_t IR; 00276 __IO uint32_t TCR; 00277 __IO uint32_t TC; 00278 __IO uint32_t PR; 00279 __IO uint32_t PC; 00280 __IO uint32_t MCR; 00281 __IO uint32_t MR0; 00282 __IO uint32_t MR1; 00283 __IO uint32_t MR2; 00284 __IO uint32_t MR3; 00285 __IO uint32_t CCR; 00286 __I uint32_t CR0; 00287 __I uint32_t CR1; 00288 uint32_t RESERVED0[2]; 00289 __IO uint32_t EMR; 00290 uint32_t RESERVED1[12]; 00291 __IO uint32_t CTCR; 00292 } LPC_TIM_TypeDef; 00293 00294 /*------------- Pulse-Width Modulation (PWM) ---------------------------------*/ 00295 typedef struct 00296 { 00297 __IO uint32_t IR; 00298 __IO uint32_t TCR; 00299 __IO uint32_t TC; 00300 __IO uint32_t PR; 00301 __IO uint32_t PC; 00302 __IO uint32_t MCR; 00303 __IO uint32_t MR0; 00304 __IO uint32_t MR1; 00305 __IO uint32_t MR2; 00306 __IO uint32_t MR3; 00307 __IO uint32_t CCR; 00308 __I uint32_t CR0; 00309 __I uint32_t CR1; 00310 __I uint32_t CR2; 00311 __I uint32_t CR3; 00312 uint32_t RESERVED0; 00313 __IO uint32_t MR4; 00314 __IO uint32_t MR5; 00315 __IO uint32_t MR6; 00316 __IO uint32_t PCR; 00317 __IO uint32_t LER; 00318 uint32_t RESERVED1[7]; 00319 __IO uint32_t CTCR; 00320 } LPC_PWM_TypeDef; 00321 00322 /*------------- Universal Asynchronous Receiver Transmitter (UART) -----------*/ 00323 typedef struct 00324 { 00325 union { 00326 __I uint8_t RBR; 00327 __O uint8_t THR; 00328 __IO uint8_t DLL; 00329 uint32_t RESERVED0; 00330 }; 00331 union { 00332 __IO uint8_t DLM; 00333 __IO uint32_t IER; 00334 }; 00335 union { 00336 __I uint32_t IIR; 00337 __O uint8_t FCR; 00338 }; 00339 __IO uint8_t LCR; 00340 uint8_t RESERVED1[7]; 00341 __I uint8_t LSR; 00342 uint8_t RESERVED2[7]; 00343 __IO uint8_t SCR; 00344 uint8_t RESERVED3[3]; 00345 __IO uint32_t ACR; 00346 __IO uint8_t ICR; 00347 uint8_t RESERVED4[3]; 00348 __IO uint8_t FDR; 00349 uint8_t RESERVED5[7]; 00350 __IO uint8_t TER; 00351 uint8_t RESERVED6[39]; 00352 __IO uint32_t FIFOLVL; 00353 } LPC_UART_TypeDef; 00354 00355 typedef struct 00356 { 00357 union { 00358 __I uint8_t RBR; 00359 __O uint8_t THR; 00360 __IO uint8_t DLL; 00361 uint32_t RESERVED0; 00362 }; 00363 union { 00364 __IO uint8_t DLM; 00365 __IO uint32_t IER; 00366 }; 00367 union { 00368 __I uint32_t IIR; 00369 __O uint8_t FCR; 00370 }; 00371 __IO uint8_t LCR; 00372 uint8_t RESERVED1[7]; 00373 __I uint8_t LSR; 00374 uint8_t RESERVED2[7]; 00375 __IO uint8_t SCR; 00376 uint8_t RESERVED3[3]; 00377 __IO uint32_t ACR; 00378 __IO uint8_t ICR; 00379 uint8_t RESERVED4[3]; 00380 __IO uint8_t FDR; 00381 uint8_t RESERVED5[7]; 00382 __IO uint8_t TER; 00383 uint8_t RESERVED6[39]; 00384 __IO uint32_t FIFOLVL; 00385 } LPC_UART0_TypeDef; 00386 00387 typedef struct 00388 { 00389 union { 00390 __I uint8_t RBR; 00391 __O uint8_t THR; 00392 __IO uint8_t DLL; 00393 uint32_t RESERVED0; 00394 }; 00395 union { 00396 __IO uint8_t DLM; 00397 __IO uint32_t IER; 00398 }; 00399 union { 00400 __I uint32_t IIR; 00401 __O uint8_t FCR; 00402 }; 00403 __IO uint8_t LCR; 00404 uint8_t RESERVED1[3]; 00405 __IO uint8_t MCR; 00406 uint8_t RESERVED2[3]; 00407 __I uint8_t LSR; 00408 uint8_t RESERVED3[3]; 00409 __I uint8_t MSR; 00410 uint8_t RESERVED4[3]; 00411 __IO uint8_t SCR; 00412 uint8_t RESERVED5[3]; 00413 __IO uint32_t ACR; 00414 uint32_t RESERVED6; 00415 __IO uint32_t FDR; 00416 uint32_t RESERVED7; 00417 __IO uint8_t TER; 00418 uint8_t RESERVED8[27]; 00419 __IO uint8_t RS485CTRL; 00420 uint8_t RESERVED9[3]; 00421 __IO uint8_t ADRMATCH; 00422 uint8_t RESERVED10[3]; 00423 __IO uint8_t RS485DLY; 00424 uint8_t RESERVED11[3]; 00425 __IO uint32_t FIFOLVL; 00426 } LPC_UART1_TypeDef; 00427 00428 /*------------- Serial Peripheral Interface (SPI) ----------------------------*/ 00429 typedef struct 00430 { 00431 __IO uint32_t SPCR; 00432 __I uint32_t SPSR; 00433 __IO uint32_t SPDR; 00434 __IO uint32_t SPCCR; 00435 uint32_t RESERVED0[3]; 00436 __IO uint32_t SPINT; 00437 } LPC_SPI_TypeDef; 00438 00439 /*------------- Synchronous Serial Communication (SSP) -----------------------*/ 00440 typedef struct 00441 { 00442 __IO uint32_t CR0; 00443 __IO uint32_t CR1; 00444 __IO uint32_t DR; 00445 __I uint32_t SR; 00446 __IO uint32_t CPSR; 00447 __IO uint32_t IMSC; 00448 __IO uint32_t RIS; 00449 __IO uint32_t MIS; 00450 __IO uint32_t ICR; 00451 __IO uint32_t DMACR; 00452 } LPC_SSP_TypeDef; 00453 00454 /*------------- Inter-Integrated Circuit (I2C) -------------------------------*/ 00455 typedef struct 00456 { 00457 __IO uint32_t I2CONSET; 00458 __I uint32_t I2STAT; 00459 __IO uint32_t I2DAT; 00460 __IO uint32_t I2ADR0; 00461 __IO uint32_t I2SCLH; 00462 __IO uint32_t I2SCLL; 00463 __O uint32_t I2CONCLR; 00464 __IO uint32_t MMCTRL; 00465 __IO uint32_t I2ADR1; 00466 __IO uint32_t I2ADR2; 00467 __IO uint32_t I2ADR3; 00468 __I uint32_t I2DATA_BUFFER; 00469 __IO uint32_t I2MASK0; 00470 __IO uint32_t I2MASK1; 00471 __IO uint32_t I2MASK2; 00472 __IO uint32_t I2MASK3; 00473 } LPC_I2C_TypeDef; 00474 00475 /*------------- Inter IC Sound (I2S) -----------------------------------------*/ 00476 typedef struct 00477 { 00478 __IO uint32_t I2SDAO; 00479 __IO uint32_t I2SDAI; 00480 __O uint32_t I2STXFIFO; 00481 __I uint32_t I2SRXFIFO; 00482 __I uint32_t I2SSTATE; 00483 __IO uint32_t I2SDMA1; 00484 __IO uint32_t I2SDMA2; 00485 __IO uint32_t I2SIRQ; 00486 __IO uint32_t I2STXRATE; 00487 __IO uint32_t I2SRXRATE; 00488 __IO uint32_t I2STXBITRATE; 00489 __IO uint32_t I2SRXBITRATE; 00490 __IO uint32_t I2STXMODE; 00491 __IO uint32_t I2SRXMODE; 00492 } LPC_I2S_TypeDef; 00493 00494 /*------------- Repetitive Interrupt Timer (RIT) -----------------------------*/ 00495 typedef struct 00496 { 00497 __IO uint32_t RICOMPVAL; 00498 __IO uint32_t RIMASK; 00499 __IO uint8_t RICTRL; 00500 uint8_t RESERVED0[3]; 00501 __IO uint32_t RICOUNTER; 00502 } LPC_RIT_TypeDef; 00503 00504 /*------------- Real-Time Clock (RTC) ----------------------------------------*/ 00505 typedef struct 00506 { 00507 __IO uint8_t ILR; 00508 uint8_t RESERVED0[7]; 00509 __IO uint8_t CCR; 00510 uint8_t RESERVED1[3]; 00511 __IO uint8_t CIIR; 00512 uint8_t RESERVED2[3]; 00513 __IO uint8_t AMR; 00514 uint8_t RESERVED3[3]; 00515 __I uint32_t CTIME0; 00516 __I uint32_t CTIME1; 00517 __I uint32_t CTIME2; 00518 __IO uint8_t SEC; 00519 uint8_t RESERVED4[3]; 00520 __IO uint8_t MIN; 00521 uint8_t RESERVED5[3]; 00522 __IO uint8_t HOUR; 00523 uint8_t RESERVED6[3]; 00524 __IO uint8_t DOM; 00525 uint8_t RESERVED7[3]; 00526 __IO uint8_t DOW; 00527 uint8_t RESERVED8[3]; 00528 __IO uint16_t DOY; 00529 uint16_t RESERVED9; 00530 __IO uint8_t MONTH; 00531 uint8_t RESERVED10[3]; 00532 __IO uint16_t YEAR; 00533 uint16_t RESERVED11; 00534 __IO uint32_t CALIBRATION; 00535 __IO uint32_t GPREG0; 00536 __IO uint32_t GPREG1; 00537 __IO uint32_t GPREG2; 00538 __IO uint32_t GPREG3; 00539 __IO uint32_t GPREG4; 00540 __IO uint8_t RTC_AUXEN; 00541 uint8_t RESERVED12[3]; 00542 __IO uint8_t RTC_AUX; 00543 uint8_t RESERVED13[3]; 00544 __IO uint8_t ALSEC; 00545 uint8_t RESERVED14[3]; 00546 __IO uint8_t ALMIN; 00547 uint8_t RESERVED15[3]; 00548 __IO uint8_t ALHOUR; 00549 uint8_t RESERVED16[3]; 00550 __IO uint8_t ALDOM; 00551 uint8_t RESERVED17[3]; 00552 __IO uint8_t ALDOW; 00553 uint8_t RESERVED18[3]; 00554 __IO uint16_t ALDOY; 00555 uint16_t RESERVED19; 00556 __IO uint8_t ALMON; 00557 uint8_t RESERVED20[3]; 00558 __IO uint16_t ALYEAR; 00559 uint16_t RESERVED21; 00560 } LPC_RTC_TypeDef; 00561 00562 /*------------- Watchdog Timer (WDT) -----------------------------------------*/ 00563 typedef struct 00564 { 00565 __IO uint8_t WDMOD; 00566 uint8_t RESERVED0[3]; 00567 __IO uint32_t WDTC; 00568 __O uint8_t WDFEED; 00569 uint8_t RESERVED1[3]; 00570 __I uint32_t WDTV; 00571 __IO uint32_t WDCLKSEL; 00572 } LPC_WDT_TypeDef; 00573 00574 /*------------- Analog-to-Digital Converter (ADC) ----------------------------*/ 00575 typedef struct 00576 { 00577 __IO uint32_t ADCR; 00578 __IO uint32_t ADGDR; 00579 uint32_t RESERVED0; 00580 __IO uint32_t ADINTEN; 00581 __I uint32_t ADDR0; 00582 __I uint32_t ADDR1; 00583 __I uint32_t ADDR2; 00584 __I uint32_t ADDR3; 00585 __I uint32_t ADDR4; 00586 __I uint32_t ADDR5; 00587 __I uint32_t ADDR6; 00588 __I uint32_t ADDR7; 00589 __I uint32_t ADSTAT; 00590 __IO uint32_t ADTRM; 00591 } LPC_ADC_TypeDef; 00592 00593 /*------------- Digital-to-Analog Converter (DAC) ----------------------------*/ 00594 typedef struct 00595 { 00596 __IO uint32_t DACR; 00597 __IO uint32_t DACCTRL; 00598 __IO uint16_t DACCNTVAL; 00599 } LPC_DAC_TypeDef; 00600 00601 /*------------- Motor Control Pulse-Width Modulation (MCPWM) -----------------*/ 00602 typedef struct 00603 { 00604 __I uint32_t MCCON; 00605 __O uint32_t MCCON_SET; 00606 __O uint32_t MCCON_CLR; 00607 __I uint32_t MCCAPCON; 00608 __O uint32_t MCCAPCON_SET; 00609 __O uint32_t MCCAPCON_CLR; 00610 __IO uint32_t MCTIM0; 00611 __IO uint32_t MCTIM1; 00612 __IO uint32_t MCTIM2; 00613 __IO uint32_t MCPER0; 00614 __IO uint32_t MCPER1; 00615 __IO uint32_t MCPER2; 00616 __IO uint32_t MCPW0; 00617 __IO uint32_t MCPW1; 00618 __IO uint32_t MCPW2; 00619 __IO uint32_t MCDEADTIME; 00620 __IO uint32_t MCCCP; 00621 __IO uint32_t MCCR0; 00622 __IO uint32_t MCCR1; 00623 __IO uint32_t MCCR2; 00624 __I uint32_t MCINTEN; 00625 __O uint32_t MCINTEN_SET; 00626 __O uint32_t MCINTEN_CLR; 00627 __I uint32_t MCCNTCON; 00628 __O uint32_t MCCNTCON_SET; 00629 __O uint32_t MCCNTCON_CLR; 00630 __I uint32_t MCINTFLAG; 00631 __O uint32_t MCINTFLAG_SET; 00632 __O uint32_t MCINTFLAG_CLR; 00633 __O uint32_t MCCAP_CLR; 00634 } LPC_MCPWM_TypeDef; 00635 00636 /*------------- Quadrature Encoder Interface (QEI) ---------------------------*/ 00637 typedef struct 00638 { 00639 __O uint32_t QEICON; 00640 __I uint32_t QEISTAT; 00641 __IO uint32_t QEICONF; 00642 __I uint32_t QEIPOS; 00643 __IO uint32_t QEIMAXPOS; 00644 __IO uint32_t CMPOS0; 00645 __IO uint32_t CMPOS1; 00646 __IO uint32_t CMPOS2; 00647 __I uint32_t INXCNT; 00648 __IO uint32_t INXCMP; 00649 __IO uint32_t QEILOAD; 00650 __I uint32_t QEITIME; 00651 __I uint32_t QEIVEL; 00652 __I uint32_t QEICAP; 00653 __IO uint32_t VELCOMP; 00654 __IO uint32_t FILTER; 00655 uint32_t RESERVED0[998]; 00656 __O uint32_t QEIIEC; 00657 __O uint32_t QEIIES; 00658 __I uint32_t QEIINTSTAT; 00659 __I uint32_t QEIIE; 00660 __O uint32_t QEICLR; 00661 __O uint32_t QEISET; 00662 } LPC_QEI_TypeDef; 00663 00664 /*------------- Controller Area Network (CAN) --------------------------------*/ 00665 typedef struct 00666 { 00667 __IO uint32_t mask[512]; /* ID Masks */ 00668 } LPC_CANAF_RAM_TypeDef; 00669 00670 typedef struct /* Acceptance Filter Registers */ 00671 { 00672 __IO uint32_t AFMR; 00673 __IO uint32_t SFF_sa; 00674 __IO uint32_t SFF_GRP_sa; 00675 __IO uint32_t EFF_sa; 00676 __IO uint32_t EFF_GRP_sa; 00677 __IO uint32_t ENDofTable; 00678 __I uint32_t LUTerrAd; 00679 __I uint32_t LUTerr; 00680 __IO uint32_t FCANIE; 00681 __IO uint32_t FCANIC0; 00682 __IO uint32_t FCANIC1; 00683 } LPC_CANAF_TypeDef; 00684 00685 typedef struct /* Central Registers */ 00686 { 00687 __I uint32_t CANTxSR; 00688 __I uint32_t CANRxSR; 00689 __I uint32_t CANMSR; 00690 } LPC_CANCR_TypeDef; 00691 00692 typedef struct /* Controller Registers */ 00693 { 00694 __IO uint32_t MOD; 00695 __O uint32_t CMR; 00696 __IO uint32_t GSR; 00697 __I uint32_t ICR; 00698 __IO uint32_t IER; 00699 __IO uint32_t BTR; 00700 __IO uint32_t EWL; 00701 __I uint32_t SR; 00702 __IO uint32_t RFS; 00703 __IO uint32_t RID; 00704 __IO uint32_t RDA; 00705 __IO uint32_t RDB; 00706 __IO uint32_t TFI1; 00707 __IO uint32_t TID1; 00708 __IO uint32_t TDA1; 00709 __IO uint32_t TDB1; 00710 __IO uint32_t TFI2; 00711 __IO uint32_t TID2; 00712 __IO uint32_t TDA2; 00713 __IO uint32_t TDB2; 00714 __IO uint32_t TFI3; 00715 __IO uint32_t TID3; 00716 __IO uint32_t TDA3; 00717 __IO uint32_t TDB3; 00718 } LPC_CAN_TypeDef; 00719 00720 /*------------- General Purpose Direct Memory Access (GPDMA) -----------------*/ 00721 typedef struct /* Common Registers */ 00722 { 00723 __I uint32_t DMACIntStat; 00724 __I uint32_t DMACIntTCStat; 00725 __O uint32_t DMACIntTCClear; 00726 __I uint32_t DMACIntErrStat; 00727 __O uint32_t DMACIntErrClr; 00728 __I uint32_t DMACRawIntTCStat; 00729 __I uint32_t DMACRawIntErrStat; 00730 __I uint32_t DMACEnbldChns; 00731 __IO uint32_t DMACSoftBReq; 00732 __IO uint32_t DMACSoftSReq; 00733 __IO uint32_t DMACSoftLBReq; 00734 __IO uint32_t DMACSoftLSReq; 00735 __IO uint32_t DMACConfig; 00736 __IO uint32_t DMACSync; 00737 } LPC_GPDMA_TypeDef; 00738 00739 typedef struct /* Channel Registers */ 00740 { 00741 __IO uint32_t DMACCSrcAddr; 00742 __IO uint32_t DMACCDestAddr; 00743 __IO uint32_t DMACCLLI; 00744 __IO uint32_t DMACCControl; 00745 __IO uint32_t DMACCConfig; 00746 } LPC_GPDMACH_TypeDef; 00747 00748 /*------------- Universal Serial Bus (USB) -----------------------------------*/ 00749 typedef struct 00750 { 00751 __I uint32_t HcRevision; /* USB Host Registers */ 00752 __IO uint32_t HcControl; 00753 __IO uint32_t HcCommandStatus; 00754 __IO uint32_t HcInterruptStatus; 00755 __IO uint32_t HcInterruptEnable; 00756 __IO uint32_t HcInterruptDisable; 00757 __IO uint32_t HcHCCA; 00758 __I uint32_t HcPeriodCurrentED; 00759 __IO uint32_t HcControlHeadED; 00760 __IO uint32_t HcControlCurrentED; 00761 __IO uint32_t HcBulkHeadED; 00762 __IO uint32_t HcBulkCurrentED; 00763 __I uint32_t HcDoneHead; 00764 __IO uint32_t HcFmInterval; 00765 __I uint32_t HcFmRemaining; 00766 __I uint32_t HcFmNumber; 00767 __IO uint32_t HcPeriodicStart; 00768 __IO uint32_t HcLSTreshold; 00769 __IO uint32_t HcRhDescriptorA; 00770 __IO uint32_t HcRhDescriptorB; 00771 __IO uint32_t HcRhStatus; 00772 __IO uint32_t HcRhPortStatus1; 00773 __IO uint32_t HcRhPortStatus2; 00774 uint32_t RESERVED0[40]; 00775 __I uint32_t Module_ID; 00776 00777 __I uint32_t OTGIntSt; /* USB On-The-Go Registers */ 00778 __IO uint32_t OTGIntEn; 00779 __O uint32_t OTGIntSet; 00780 __O uint32_t OTGIntClr; 00781 __IO uint32_t OTGStCtrl; 00782 __IO uint32_t OTGTmr; 00783 uint32_t RESERVED1[58]; 00784 00785 __I uint32_t USBDevIntSt; /* USB Device Interrupt Registers */ 00786 __IO uint32_t USBDevIntEn; 00787 __O uint32_t USBDevIntClr; 00788 __O uint32_t USBDevIntSet; 00789 00790 __O uint32_t USBCmdCode; /* USB Device SIE Command Registers */ 00791 __I uint32_t USBCmdData; 00792 00793 __I uint32_t USBRxData; /* USB Device Transfer Registers */ 00794 __O uint32_t USBTxData; 00795 __I uint32_t USBRxPLen; 00796 __O uint32_t USBTxPLen; 00797 __IO uint32_t USBCtrl; 00798 __O uint32_t USBDevIntPri; 00799 00800 __I uint32_t USBEpIntSt; /* USB Device Endpoint Interrupt Regs */ 00801 __IO uint32_t USBEpIntEn; 00802 __O uint32_t USBEpIntClr; 00803 __O uint32_t USBEpIntSet; 00804 __O uint32_t USBEpIntPri; 00805 00806 __IO uint32_t USBReEp; /* USB Device Endpoint Realization Reg*/ 00807 __O uint32_t USBEpInd; 00808 __IO uint32_t USBMaxPSize; 00809 00810 __I uint32_t USBDMARSt; /* USB Device DMA Registers */ 00811 __O uint32_t USBDMARClr; 00812 __O uint32_t USBDMARSet; 00813 uint32_t RESERVED2[9]; 00814 __IO uint32_t USBUDCAH; 00815 __I uint32_t USBEpDMASt; 00816 __O uint32_t USBEpDMAEn; 00817 __O uint32_t USBEpDMADis; 00818 __I uint32_t USBDMAIntSt; 00819 __IO uint32_t USBDMAIntEn; 00820 uint32_t RESERVED3[2]; 00821 __I uint32_t USBEoTIntSt; 00822 __O uint32_t USBEoTIntClr; 00823 __O uint32_t USBEoTIntSet; 00824 __I uint32_t USBNDDRIntSt; 00825 __O uint32_t USBNDDRIntClr; 00826 __O uint32_t USBNDDRIntSet; 00827 __I uint32_t USBSysErrIntSt; 00828 __O uint32_t USBSysErrIntClr; 00829 __O uint32_t USBSysErrIntSet; 00830 uint32_t RESERVED4[15]; 00831 00832 union { 00833 __I uint32_t I2C_RX; /* USB OTG I2C Registers */ 00834 __O uint32_t I2C_TX; 00835 }; 00836 __I uint32_t I2C_STS; 00837 __IO uint32_t I2C_CTL; 00838 __IO uint32_t I2C_CLKHI; 00839 __O uint32_t I2C_CLKLO; 00840 uint32_t RESERVED5[824]; 00841 00842 union { 00843 __IO uint32_t USBClkCtrl; /* USB Clock Control Registers */ 00844 __IO uint32_t OTGClkCtrl; 00845 }; 00846 union { 00847 __I uint32_t USBClkSt; 00848 __I uint32_t OTGClkSt; 00849 }; 00850 } LPC_USB_TypeDef; 00851 00852 /*------------- Ethernet Media Access Controller (EMAC) ----------------------*/ 00853 typedef struct 00854 { 00855 __IO uint32_t MAC1; /* MAC Registers */ 00856 __IO uint32_t MAC2; 00857 __IO uint32_t IPGT; 00858 __IO uint32_t IPGR; 00859 __IO uint32_t CLRT; 00860 __IO uint32_t MAXF; 00861 __IO uint32_t SUPP; 00862 __IO uint32_t TEST; 00863 __IO uint32_t MCFG; 00864 __IO uint32_t MCMD; 00865 __IO uint32_t MADR; 00866 __O uint32_t MWTD; 00867 __I uint32_t MRDD; 00868 __I uint32_t MIND; 00869 uint32_t RESERVED0[2]; 00870 __IO uint32_t SA0; 00871 __IO uint32_t SA1; 00872 __IO uint32_t SA2; 00873 uint32_t RESERVED1[45]; 00874 __IO uint32_t Command; /* Control Registers */ 00875 __I uint32_t Status; 00876 __IO uint32_t RxDescriptor; 00877 __IO uint32_t RxStatus; 00878 __IO uint32_t RxDescriptorNumber; 00879 __I uint32_t RxProduceIndex; 00880 __IO uint32_t RxConsumeIndex; 00881 __IO uint32_t TxDescriptor; 00882 __IO uint32_t TxStatus; 00883 __IO uint32_t TxDescriptorNumber; 00884 __IO uint32_t TxProduceIndex; 00885 __I uint32_t TxConsumeIndex; 00886 uint32_t RESERVED2[10]; 00887 __I uint32_t TSV0; 00888 __I uint32_t TSV1; 00889 __I uint32_t RSV; 00890 uint32_t RESERVED3[3]; 00891 __IO uint32_t FlowControlCounter; 00892 __I uint32_t FlowControlStatus; 00893 uint32_t RESERVED4[34]; 00894 __IO uint32_t RxFilterCtrl; /* Rx Filter Registers */ 00895 __IO uint32_t RxFilterWoLStatus; 00896 __IO uint32_t RxFilterWoLClear; 00897 uint32_t RESERVED5; 00898 __IO uint32_t HashFilterL; 00899 __IO uint32_t HashFilterH; 00900 uint32_t RESERVED6[882]; 00901 __I uint32_t IntStatus; /* Module Control Registers */ 00902 __IO uint32_t IntEnable; 00903 __O uint32_t IntClear; 00904 __O uint32_t IntSet; 00905 uint32_t RESERVED7; 00906 __IO uint32_t PowerDown; 00907 uint32_t RESERVED8; 00908 __IO uint32_t Module_ID; 00909 } LPC_EMAC_TypeDef; 00910 00911 #if defined ( __CC_ARM ) 00912 #pragma no_anon_unions 00913 #endif 00914 00915 00916 /******************************************************************************/ 00917 /* Peripheral memory map */ 00918 /******************************************************************************/ 00919 /* Base addresses */ 00920 #define LPC_FLASH_BASE (0x00000000UL) 00921 #define LPC_RAM_BASE (0x10000000UL) 00922 #define LPC_GPIO_BASE (0x2009C000UL) 00923 #define LPC_APB0_BASE (0x40000000UL) 00924 #define LPC_APB1_BASE (0x40080000UL) 00925 #define LPC_AHB_BASE (0x50000000UL) 00926 #define LPC_CM3_BASE (0xE0000000UL) 00927 00928 /* APB0 peripherals */ 00929 #define LPC_WDT_BASE (LPC_APB0_BASE + 0x00000) 00930 #define LPC_TIM0_BASE (LPC_APB0_BASE + 0x04000) 00931 #define LPC_TIM1_BASE (LPC_APB0_BASE + 0x08000) 00932 #define LPC_UART0_BASE (LPC_APB0_BASE + 0x0C000) 00933 #define LPC_UART1_BASE (LPC_APB0_BASE + 0x10000) 00934 #define LPC_PWM1_BASE (LPC_APB0_BASE + 0x18000) 00935 #define LPC_I2C0_BASE (LPC_APB0_BASE + 0x1C000) 00936 #define LPC_SPI_BASE (LPC_APB0_BASE + 0x20000) 00937 #define LPC_RTC_BASE (LPC_APB0_BASE + 0x24000) 00938 #define LPC_GPIOINT_BASE (LPC_APB0_BASE + 0x28080) 00939 #define LPC_PINCON_BASE (LPC_APB0_BASE + 0x2C000) 00940 #define LPC_SSP1_BASE (LPC_APB0_BASE + 0x30000) 00941 #define LPC_ADC_BASE (LPC_APB0_BASE + 0x34000) 00942 #define LPC_CANAF_RAM_BASE (LPC_APB0_BASE + 0x38000) 00943 #define LPC_CANAF_BASE (LPC_APB0_BASE + 0x3C000) 00944 #define LPC_CANCR_BASE (LPC_APB0_BASE + 0x40000) 00945 #define LPC_CAN1_BASE (LPC_APB0_BASE + 0x44000) 00946 #define LPC_CAN2_BASE (LPC_APB0_BASE + 0x48000) 00947 #define LPC_I2C1_BASE (LPC_APB0_BASE + 0x5C000) 00948 00949 /* APB1 peripherals */ 00950 #define LPC_SSP0_BASE (LPC_APB1_BASE + 0x08000) 00951 #define LPC_DAC_BASE (LPC_APB1_BASE + 0x0C000) 00952 #define LPC_TIM2_BASE (LPC_APB1_BASE + 0x10000) 00953 #define LPC_TIM3_BASE (LPC_APB1_BASE + 0x14000) 00954 #define LPC_UART2_BASE (LPC_APB1_BASE + 0x18000) 00955 #define LPC_UART3_BASE (LPC_APB1_BASE + 0x1C000) 00956 #define LPC_I2C2_BASE (LPC_APB1_BASE + 0x20000) 00957 #define LPC_I2S_BASE (LPC_APB1_BASE + 0x28000) 00958 #define LPC_RIT_BASE (LPC_APB1_BASE + 0x30000) 00959 #define LPC_MCPWM_BASE (LPC_APB1_BASE + 0x38000) 00960 #define LPC_QEI_BASE (LPC_APB1_BASE + 0x3C000) 00961 #define LPC_SC_BASE (LPC_APB1_BASE + 0x7C000) 00962 00963 /* AHB peripherals */ 00964 #define LPC_EMAC_BASE (LPC_AHB_BASE + 0x00000) 00965 #define LPC_GPDMA_BASE (LPC_AHB_BASE + 0x04000) 00966 #define LPC_GPDMACH0_BASE (LPC_AHB_BASE + 0x04100) 00967 #define LPC_GPDMACH1_BASE (LPC_AHB_BASE + 0x04120) 00968 #define LPC_GPDMACH2_BASE (LPC_AHB_BASE + 0x04140) 00969 #define LPC_GPDMACH3_BASE (LPC_AHB_BASE + 0x04160) 00970 #define LPC_GPDMACH4_BASE (LPC_AHB_BASE + 0x04180) 00971 #define LPC_GPDMACH5_BASE (LPC_AHB_BASE + 0x041A0) 00972 #define LPC_GPDMACH6_BASE (LPC_AHB_BASE + 0x041C0) 00973 #define LPC_GPDMACH7_BASE (LPC_AHB_BASE + 0x041E0) 00974 #define LPC_USB_BASE (LPC_AHB_BASE + 0x0C000) 00975 00976 /* GPIOs */ 00977 #define LPC_GPIO0_BASE (LPC_GPIO_BASE + 0x00000) 00978 #define LPC_GPIO1_BASE (LPC_GPIO_BASE + 0x00020) 00979 #define LPC_GPIO2_BASE (LPC_GPIO_BASE + 0x00040) 00980 #define LPC_GPIO3_BASE (LPC_GPIO_BASE + 0x00060) 00981 #define LPC_GPIO4_BASE (LPC_GPIO_BASE + 0x00080) 00982 00983 00984 /******************************************************************************/ 00985 /* Peripheral declaration */ 00986 /******************************************************************************/ 00987 #define LPC_SC ((LPC_SC_TypeDef *) LPC_SC_BASE ) 00988 #define LPC_GPIO0 ((LPC_GPIO_TypeDef *) LPC_GPIO0_BASE ) 00989 #define LPC_GPIO1 ((LPC_GPIO_TypeDef *) LPC_GPIO1_BASE ) 00990 #define LPC_GPIO2 ((LPC_GPIO_TypeDef *) LPC_GPIO2_BASE ) 00991 #define LPC_GPIO3 ((LPC_GPIO_TypeDef *) LPC_GPIO3_BASE ) 00992 #define LPC_GPIO4 ((LPC_GPIO_TypeDef *) LPC_GPIO4_BASE ) 00993 #define LPC_WDT ((LPC_WDT_TypeDef *) LPC_WDT_BASE ) 00994 #define LPC_TIM0 ((LPC_TIM_TypeDef *) LPC_TIM0_BASE ) 00995 #define LPC_TIM1 ((LPC_TIM_TypeDef *) LPC_TIM1_BASE ) 00996 #define LPC_TIM2 ((LPC_TIM_TypeDef *) LPC_TIM2_BASE ) 00997 #define LPC_TIM3 ((LPC_TIM_TypeDef *) LPC_TIM3_BASE ) 00998 #define LPC_RIT ((LPC_RIT_TypeDef *) LPC_RIT_BASE ) 00999 #define LPC_UART0 ((LPC_UART0_TypeDef *) LPC_UART0_BASE ) 01000 #define LPC_UART1 ((LPC_UART1_TypeDef *) LPC_UART1_BASE ) 01001 #define LPC_UART2 ((LPC_UART_TypeDef *) LPC_UART2_BASE ) 01002 #define LPC_UART3 ((LPC_UART_TypeDef *) LPC_UART3_BASE ) 01003 #define LPC_PWM1 ((LPC_PWM_TypeDef *) LPC_PWM1_BASE ) 01004 #define LPC_I2C0 ((LPC_I2C_TypeDef *) LPC_I2C0_BASE ) 01005 #define LPC_I2C1 ((LPC_I2C_TypeDef *) LPC_I2C1_BASE ) 01006 #define LPC_I2C2 ((LPC_I2C_TypeDef *) LPC_I2C2_BASE ) 01007 #define LPC_I2S ((LPC_I2S_TypeDef *) LPC_I2S_BASE ) 01008 #define LPC_SPI ((LPC_SPI_TypeDef *) LPC_SPI_BASE ) 01009 #define LPC_RTC ((LPC_RTC_TypeDef *) LPC_RTC_BASE ) 01010 #define LPC_GPIOINT ((LPC_GPIOINT_TypeDef *) LPC_GPIOINT_BASE ) 01011 #define LPC_PINCON ((LPC_PINCON_TypeDef *) LPC_PINCON_BASE ) 01012 #define LPC_SSP0 ((LPC_SSP_TypeDef *) LPC_SSP0_BASE ) 01013 #define LPC_SSP1 ((LPC_SSP_TypeDef *) LPC_SSP1_BASE ) 01014 #define LPC_ADC ((LPC_ADC_TypeDef *) LPC_ADC_BASE ) 01015 #define LPC_DAC ((LPC_DAC_TypeDef *) LPC_DAC_BASE ) 01016 #define LPC_CANAF_RAM ((LPC_CANAF_RAM_TypeDef *) LPC_CANAF_RAM_BASE) 01017 #define LPC_CANAF ((LPC_CANAF_TypeDef *) LPC_CANAF_BASE ) 01018 #define LPC_CANCR ((LPC_CANCR_TypeDef *) LPC_CANCR_BASE ) 01019 #define LPC_CAN1 ((LPC_CAN_TypeDef *) LPC_CAN1_BASE ) 01020 #define LPC_CAN2 ((LPC_CAN_TypeDef *) LPC_CAN2_BASE ) 01021 #define LPC_MCPWM ((LPC_MCPWM_TypeDef *) LPC_MCPWM_BASE ) 01022 #define LPC_QEI ((LPC_QEI_TypeDef *) LPC_QEI_BASE ) 01023 #define LPC_EMAC ((LPC_EMAC_TypeDef *) LPC_EMAC_BASE ) 01024 #define LPC_GPDMA ((LPC_GPDMA_TypeDef *) LPC_GPDMA_BASE ) 01025 #define LPC_GPDMACH0 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH0_BASE ) 01026 #define LPC_GPDMACH1 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH1_BASE ) 01027 #define LPC_GPDMACH2 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH2_BASE ) 01028 #define LPC_GPDMACH3 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH3_BASE ) 01029 #define LPC_GPDMACH4 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH4_BASE ) 01030 #define LPC_GPDMACH5 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH5_BASE ) 01031 #define LPC_GPDMACH6 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH6_BASE ) 01032 #define LPC_GPDMACH7 ((LPC_GPDMACH_TypeDef *) LPC_GPDMACH7_BASE ) 01033 #define LPC_USB ((LPC_USB_TypeDef *) LPC_USB_BASE ) 01034 01035 #endif // __LPC17xx_H__
Generated on Tue Jul 12 2022 11:27:26 by
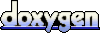