Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
core_cmInstr.h
00001 /**************************************************************************//** 00002 * @file core_cmInstr.h 00003 * @brief CMSIS Cortex-M Core Instruction Access Header File 00004 * @version V3.00 00005 * @date 09. December 2011 00006 * 00007 * @note 00008 * Copyright (C) 2009-2011 ARM Limited. All rights reserved. 00009 * 00010 * @par 00011 * ARM Limited (ARM) is supplying this software for use with Cortex-M 00012 * processor based microcontrollers. This file can be freely distributed 00013 * within development tools that are supporting such ARM based processors. 00014 * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 00024 #ifndef __CORE_CMINSTR_H 00025 #define __CORE_CMINSTR_H 00026 00027 00028 /* ########################## Core Instruction Access ######################### */ 00029 /** \defgroup CMSIS_Core_InstructionInterface CMSIS Core Instruction Interface 00030 Access to dedicated instructions 00031 @{ 00032 */ 00033 00034 #if defined ( __CC_ARM ) /*------------------RealView Compiler -----------------*/ 00035 /* ARM armcc specific functions */ 00036 00037 #if (__ARMCC_VERSION < 400677) 00038 #error "Please use ARM Compiler Toolchain V4.0.677 or later!" 00039 #endif 00040 00041 00042 /** \brief No Operation 00043 00044 No Operation does nothing. This instruction can be used for code alignment purposes. 00045 */ 00046 #define __NOP __nop 00047 00048 00049 /** \brief Wait For Interrupt 00050 00051 Wait For Interrupt is a hint instruction that suspends execution 00052 until one of a number of events occurs. 00053 */ 00054 #define __WFI __wfi 00055 00056 00057 /** \brief Wait For Event 00058 00059 Wait For Event is a hint instruction that permits the processor to enter 00060 a low-power state until one of a number of events occurs. 00061 */ 00062 #define __WFE __wfe 00063 00064 00065 /** \brief Send Event 00066 00067 Send Event is a hint instruction. It causes an event to be signaled to the CPU. 00068 */ 00069 #define __SEV __sev 00070 00071 00072 /** \brief Instruction Synchronization Barrier 00073 00074 Instruction Synchronization Barrier flushes the pipeline in the processor, 00075 so that all instructions following the ISB are fetched from cache or 00076 memory, after the instruction has been completed. 00077 */ 00078 #define __ISB() __isb(0xF) 00079 00080 00081 /** \brief Data Synchronization Barrier 00082 00083 This function acts as a special kind of Data Memory Barrier. 00084 It completes when all explicit memory accesses before this instruction complete. 00085 */ 00086 #define __DSB() __dsb(0xF) 00087 00088 00089 /** \brief Data Memory Barrier 00090 00091 This function ensures the apparent order of the explicit memory operations before 00092 and after the instruction, without ensuring their completion. 00093 */ 00094 #define __DMB() __dmb(0xF) 00095 00096 00097 /** \brief Reverse byte order (32 bit) 00098 00099 This function reverses the byte order in integer value. 00100 00101 \param [in] value Value to reverse 00102 \return Reversed value 00103 */ 00104 #define __REV __rev 00105 00106 00107 /** \brief Reverse byte order (16 bit) 00108 00109 This function reverses the byte order in two unsigned short values. 00110 00111 \param [in] value Value to reverse 00112 \return Reversed value 00113 */ 00114 static __attribute__((section(".rev16_text"))) __INLINE __ASM uint32_t __REV16(uint32_t value) 00115 { 00116 rev16 r0, r0 00117 bx lr 00118 } 00119 00120 00121 /** \brief Reverse byte order in signed short value 00122 00123 This function reverses the byte order in a signed short value with sign extension to integer. 00124 00125 \param [in] value Value to reverse 00126 \return Reversed value 00127 */ 00128 static __attribute__((section(".revsh_text"))) __INLINE __ASM int32_t __REVSH(int32_t value) 00129 { 00130 revsh r0, r0 00131 bx lr 00132 } 00133 00134 00135 #if (__CORTEX_M >= 0x03) 00136 00137 /** \brief Reverse bit order of value 00138 00139 This function reverses the bit order of the given value. 00140 00141 \param [in] value Value to reverse 00142 \return Reversed value 00143 */ 00144 #define __RBIT __rbit 00145 00146 00147 /** \brief LDR Exclusive (8 bit) 00148 00149 This function performs a exclusive LDR command for 8 bit value. 00150 00151 \param [in] ptr Pointer to data 00152 \return value of type uint8_t at (*ptr) 00153 */ 00154 #define __LDREXB(ptr) ((uint8_t ) __ldrex(ptr)) 00155 00156 00157 /** \brief LDR Exclusive (16 bit) 00158 00159 This function performs a exclusive LDR command for 16 bit values. 00160 00161 \param [in] ptr Pointer to data 00162 \return value of type uint16_t at (*ptr) 00163 */ 00164 #define __LDREXH(ptr) ((uint16_t) __ldrex(ptr)) 00165 00166 00167 /** \brief LDR Exclusive (32 bit) 00168 00169 This function performs a exclusive LDR command for 32 bit values. 00170 00171 \param [in] ptr Pointer to data 00172 \return value of type uint32_t at (*ptr) 00173 */ 00174 #define __LDREXW(ptr) ((uint32_t ) __ldrex(ptr)) 00175 00176 00177 /** \brief STR Exclusive (8 bit) 00178 00179 This function performs a exclusive STR command for 8 bit values. 00180 00181 \param [in] value Value to store 00182 \param [in] ptr Pointer to location 00183 \return 0 Function succeeded 00184 \return 1 Function failed 00185 */ 00186 #define __STREXB(value, ptr) __strex(value, ptr) 00187 00188 00189 /** \brief STR Exclusive (16 bit) 00190 00191 This function performs a exclusive STR command for 16 bit values. 00192 00193 \param [in] value Value to store 00194 \param [in] ptr Pointer to location 00195 \return 0 Function succeeded 00196 \return 1 Function failed 00197 */ 00198 #define __STREXH(value, ptr) __strex(value, ptr) 00199 00200 00201 /** \brief STR Exclusive (32 bit) 00202 00203 This function performs a exclusive STR command for 32 bit values. 00204 00205 \param [in] value Value to store 00206 \param [in] ptr Pointer to location 00207 \return 0 Function succeeded 00208 \return 1 Function failed 00209 */ 00210 #define __STREXW(value, ptr) __strex(value, ptr) 00211 00212 00213 /** \brief Remove the exclusive lock 00214 00215 This function removes the exclusive lock which is created by LDREX. 00216 00217 */ 00218 #define __CLREX __clrex 00219 00220 00221 /** \brief Signed Saturate 00222 00223 This function saturates a signed value. 00224 00225 \param [in] value Value to be saturated 00226 \param [in] sat Bit position to saturate to (1..32) 00227 \return Saturated value 00228 */ 00229 #define __SSAT __ssat 00230 00231 00232 /** \brief Unsigned Saturate 00233 00234 This function saturates an unsigned value. 00235 00236 \param [in] value Value to be saturated 00237 \param [in] sat Bit position to saturate to (0..31) 00238 \return Saturated value 00239 */ 00240 #define __USAT __usat 00241 00242 00243 /** \brief Count leading zeros 00244 00245 This function counts the number of leading zeros of a data value. 00246 00247 \param [in] value Value to count the leading zeros 00248 \return number of leading zeros in value 00249 */ 00250 #define __CLZ __clz 00251 00252 #endif /* (__CORTEX_M >= 0x03) */ 00253 00254 00255 00256 #elif defined ( __ICCARM__ ) /*------------------ ICC Compiler -------------------*/ 00257 /* IAR iccarm specific functions */ 00258 00259 #include <cmsis_iar.h> 00260 00261 00262 #elif defined ( __GNUC__ ) /*------------------ GNU Compiler ---------------------*/ 00263 /* GNU gcc specific functions */ 00264 00265 /** \brief No Operation 00266 00267 No Operation does nothing. This instruction can be used for code alignment purposes. 00268 */ 00269 __attribute__( ( always_inline ) ) static __INLINE void __NOP(void) 00270 { 00271 __ASM volatile ("nop"); 00272 } 00273 00274 00275 /** \brief Wait For Interrupt 00276 00277 Wait For Interrupt is a hint instruction that suspends execution 00278 until one of a number of events occurs. 00279 */ 00280 __attribute__( ( always_inline ) ) static __INLINE void __WFI(void) 00281 { 00282 __ASM volatile ("wfi"); 00283 } 00284 00285 00286 /** \brief Wait For Event 00287 00288 Wait For Event is a hint instruction that permits the processor to enter 00289 a low-power state until one of a number of events occurs. 00290 */ 00291 __attribute__( ( always_inline ) ) static __INLINE void __WFE(void) 00292 { 00293 __ASM volatile ("wfe"); 00294 } 00295 00296 00297 /** \brief Send Event 00298 00299 Send Event is a hint instruction. It causes an event to be signaled to the CPU. 00300 */ 00301 __attribute__( ( always_inline ) ) static __INLINE void __SEV(void) 00302 { 00303 __ASM volatile ("sev"); 00304 } 00305 00306 00307 /** \brief Instruction Synchronization Barrier 00308 00309 Instruction Synchronization Barrier flushes the pipeline in the processor, 00310 so that all instructions following the ISB are fetched from cache or 00311 memory, after the instruction has been completed. 00312 */ 00313 __attribute__( ( always_inline ) ) static __INLINE void __ISB(void) 00314 { 00315 __ASM volatile ("isb"); 00316 } 00317 00318 00319 /** \brief Data Synchronization Barrier 00320 00321 This function acts as a special kind of Data Memory Barrier. 00322 It completes when all explicit memory accesses before this instruction complete. 00323 */ 00324 __attribute__( ( always_inline ) ) static __INLINE void __DSB(void) 00325 { 00326 __ASM volatile ("dsb"); 00327 } 00328 00329 00330 /** \brief Data Memory Barrier 00331 00332 This function ensures the apparent order of the explicit memory operations before 00333 and after the instruction, without ensuring their completion. 00334 */ 00335 __attribute__( ( always_inline ) ) static __INLINE void __DMB(void) 00336 { 00337 __ASM volatile ("dmb"); 00338 } 00339 00340 00341 /** \brief Reverse byte order (32 bit) 00342 00343 This function reverses the byte order in integer value. 00344 00345 \param [in] value Value to reverse 00346 \return Reversed value 00347 */ 00348 __attribute__( ( always_inline ) ) static __INLINE uint32_t __REV(uint32_t value) 00349 { 00350 uint32_t result; 00351 00352 __ASM volatile ("rev %0, %1" : "=r" (result) : "r" (value) ); 00353 return(result); 00354 } 00355 00356 00357 /** \brief Reverse byte order (16 bit) 00358 00359 This function reverses the byte order in two unsigned short values. 00360 00361 \param [in] value Value to reverse 00362 \return Reversed value 00363 */ 00364 __attribute__( ( always_inline ) ) static __INLINE uint32_t __REV16(uint32_t value) 00365 { 00366 uint32_t result; 00367 00368 __ASM volatile ("rev16 %0, %1" : "=r" (result) : "r" (value) ); 00369 return(result); 00370 } 00371 00372 00373 /** \brief Reverse byte order in signed short value 00374 00375 This function reverses the byte order in a signed short value with sign extension to integer. 00376 00377 \param [in] value Value to reverse 00378 \return Reversed value 00379 */ 00380 __attribute__( ( always_inline ) ) static __INLINE int32_t __REVSH(int32_t value) 00381 { 00382 uint32_t result; 00383 00384 __ASM volatile ("revsh %0, %1" : "=r" (result) : "r" (value) ); 00385 return(result); 00386 } 00387 00388 00389 #if (__CORTEX_M >= 0x03) 00390 00391 /** \brief Reverse bit order of value 00392 00393 This function reverses the bit order of the given value. 00394 00395 \param [in] value Value to reverse 00396 \return Reversed value 00397 */ 00398 __attribute__( ( always_inline ) ) static __INLINE uint32_t __RBIT(uint32_t value) 00399 { 00400 uint32_t result; 00401 00402 __ASM volatile ("rbit %0, %1" : "=r" (result) : "r" (value) ); 00403 return(result); 00404 } 00405 00406 00407 /** \brief LDR Exclusive (8 bit) 00408 00409 This function performs a exclusive LDR command for 8 bit value. 00410 00411 \param [in] ptr Pointer to data 00412 \return value of type uint8_t at (*ptr) 00413 */ 00414 __attribute__( ( always_inline ) ) static __INLINE uint8_t __LDREXB(volatile uint8_t *addr) 00415 { 00416 uint8_t result; 00417 00418 __ASM volatile ("ldrexb %0, [%1]" : "=r" (result) : "r" (addr) ); 00419 return(result); 00420 } 00421 00422 00423 /** \brief LDR Exclusive (16 bit) 00424 00425 This function performs a exclusive LDR command for 16 bit values. 00426 00427 \param [in] ptr Pointer to data 00428 \return value of type uint16_t at (*ptr) 00429 */ 00430 __attribute__( ( always_inline ) ) static __INLINE uint16_t __LDREXH(volatile uint16_t *addr) 00431 { 00432 uint16_t result; 00433 00434 __ASM volatile ("ldrexh %0, [%1]" : "=r" (result) : "r" (addr) ); 00435 return(result); 00436 } 00437 00438 00439 /** \brief LDR Exclusive (32 bit) 00440 00441 This function performs a exclusive LDR command for 32 bit values. 00442 00443 \param [in] ptr Pointer to data 00444 \return value of type uint32_t at (*ptr) 00445 */ 00446 __attribute__( ( always_inline ) ) static __INLINE uint32_t __LDREXW(volatile uint32_t *addr) 00447 { 00448 uint32_t result; 00449 00450 __ASM volatile ("ldrex %0, [%1]" : "=r" (result) : "r" (addr) ); 00451 return(result); 00452 } 00453 00454 00455 /** \brief STR Exclusive (8 bit) 00456 00457 This function performs a exclusive STR command for 8 bit values. 00458 00459 \param [in] value Value to store 00460 \param [in] ptr Pointer to location 00461 \return 0 Function succeeded 00462 \return 1 Function failed 00463 */ 00464 __attribute__( ( always_inline ) ) static __INLINE uint32_t __STREXB(uint8_t value, volatile uint8_t *addr) 00465 { 00466 uint32_t result; 00467 00468 __ASM volatile ("strexb %0, %2, [%1]" : "=&r" (result) : "r" (addr), "r" (value) ); 00469 return(result); 00470 } 00471 00472 00473 /** \brief STR Exclusive (16 bit) 00474 00475 This function performs a exclusive STR command for 16 bit values. 00476 00477 \param [in] value Value to store 00478 \param [in] ptr Pointer to location 00479 \return 0 Function succeeded 00480 \return 1 Function failed 00481 */ 00482 __attribute__( ( always_inline ) ) static __INLINE uint32_t __STREXH(uint16_t value, volatile uint16_t *addr) 00483 { 00484 uint32_t result; 00485 00486 __ASM volatile ("strexh %0, %2, [%1]" : "=&r" (result) : "r" (addr), "r" (value) ); 00487 return(result); 00488 } 00489 00490 00491 /** \brief STR Exclusive (32 bit) 00492 00493 This function performs a exclusive STR command for 32 bit values. 00494 00495 \param [in] value Value to store 00496 \param [in] ptr Pointer to location 00497 \return 0 Function succeeded 00498 \return 1 Function failed 00499 */ 00500 __attribute__( ( always_inline ) ) static __INLINE uint32_t __STREXW(uint32_t value, volatile uint32_t *addr) 00501 { 00502 uint32_t result; 00503 00504 __ASM volatile ("strex %0, %2, [%1]" : "=&r" (result) : "r" (addr), "r" (value) ); 00505 return(result); 00506 } 00507 00508 00509 /** \brief Remove the exclusive lock 00510 00511 This function removes the exclusive lock which is created by LDREX. 00512 00513 */ 00514 __attribute__( ( always_inline ) ) static __INLINE void __CLREX(void) 00515 { 00516 __ASM volatile ("clrex"); 00517 } 00518 00519 00520 /** \brief Signed Saturate 00521 00522 This function saturates a signed value. 00523 00524 \param [in] value Value to be saturated 00525 \param [in] sat Bit position to saturate to (1..32) 00526 \return Saturated value 00527 */ 00528 #define __SSAT(ARG1,ARG2) \ 00529 ({ \ 00530 uint32_t __RES, __ARG1 = (ARG1); \ 00531 __ASM ("ssat %0, %1, %2" : "=r" (__RES) : "I" (ARG2), "r" (__ARG1) ); \ 00532 __RES; \ 00533 }) 00534 00535 00536 /** \brief Unsigned Saturate 00537 00538 This function saturates an unsigned value. 00539 00540 \param [in] value Value to be saturated 00541 \param [in] sat Bit position to saturate to (0..31) 00542 \return Saturated value 00543 */ 00544 #define __USAT(ARG1,ARG2) \ 00545 ({ \ 00546 uint32_t __RES, __ARG1 = (ARG1); \ 00547 __ASM ("usat %0, %1, %2" : "=r" (__RES) : "I" (ARG2), "r" (__ARG1) ); \ 00548 __RES; \ 00549 }) 00550 00551 00552 /** \brief Count leading zeros 00553 00554 This function counts the number of leading zeros of a data value. 00555 00556 \param [in] value Value to count the leading zeros 00557 \return number of leading zeros in value 00558 */ 00559 __attribute__( ( always_inline ) ) static __INLINE uint8_t __CLZ(uint32_t value) 00560 { 00561 uint8_t result; 00562 00563 __ASM volatile ("clz %0, %1" : "=r" (result) : "r" (value) ); 00564 return(result); 00565 } 00566 00567 #endif /* (__CORTEX_M >= 0x03) */ 00568 00569 00570 00571 00572 #elif defined ( __TASKING__ ) /*------------------ TASKING Compiler --------------*/ 00573 /* TASKING carm specific functions */ 00574 00575 /* 00576 * The CMSIS functions have been implemented as intrinsics in the compiler. 00577 * Please use "carm -?i" to get an up to date list of all intrinsics, 00578 * Including the CMSIS ones. 00579 */ 00580 00581 #endif 00582 00583 /*@}*/ /* end of group CMSIS_Core_InstructionInterface */ 00584 00585 #endif /* __CORE_CMINSTR_H */
Generated on Tue Jul 12 2022 11:27:26 by
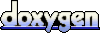