Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
InterruptIn.h
00001 /* mbed Microcontroller Library - InterruptIn 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_INTERRUPTIN_H 00006 #define MBED_INTERRUPTIN_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_INTERRUPTIN 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 #include "FunctionPointer.h" 00017 00018 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00019 #define CHANNEL_NUM 48 00020 #elif defined(TARGET_LPC11U24) 00021 #define CHANNEL_NUM 8 00022 #endif 00023 00024 namespace mbed { 00025 00026 /** A digital interrupt input, used to call a function on a rising or falling edge 00027 * 00028 * Example: 00029 * @code 00030 * // Flash an LED while waiting for events 00031 * 00032 * #include "mbed.h" 00033 * 00034 * InterruptIn event(p16); 00035 * DigitalOut led(LED1); 00036 * 00037 * void trigger() { 00038 * printf("triggered!\n"); 00039 * } 00040 * 00041 * int main() { 00042 * event.rise(&trigger); 00043 * while(1) { 00044 * led = !led; 00045 * wait(0.25); 00046 * } 00047 * } 00048 * @endcode 00049 */ 00050 class InterruptIn : public Base { 00051 00052 public: 00053 00054 /** Create an InterruptIn connected to the specified pin 00055 * 00056 * @param pin InterruptIn pin to connect to 00057 * @param name (optional) A string to identify the object 00058 */ 00059 InterruptIn(PinName pin, const char *name = NULL); 00060 #if defined(TARGET_LPC11U24) 00061 virtual ~InterruptIn(); 00062 #endif 00063 00064 int read(); 00065 #ifdef MBED_OPERATORS 00066 operator int(); 00067 00068 #endif 00069 00070 /** Attach a function to call when a rising edge occurs on the input 00071 * 00072 * @param fptr A pointer to a void function, or 0 to set as none 00073 */ 00074 void rise(void (*fptr)(void)); 00075 00076 /** Attach a member function to call when a rising edge occurs on the input 00077 * 00078 * @param tptr pointer to the object to call the member function on 00079 * @param mptr pointer to the member function to be called 00080 */ 00081 template<typename T> 00082 void rise(T* tptr, void (T::*mptr)(void)) { 00083 _rise.attach(tptr, mptr); 00084 setup_interrupt(1, 1); 00085 } 00086 00087 /** Attach a function to call when a falling edge occurs on the input 00088 * 00089 * @param fptr A pointer to a void function, or 0 to set as none 00090 */ 00091 void fall(void (*fptr)(void)); 00092 00093 /** Attach a member function to call when a falling edge occurs on the input 00094 * 00095 * @param tptr pointer to the object to call the member function on 00096 * @param mptr pointer to the member function to be called 00097 */ 00098 template<typename T> 00099 void fall(T* tptr, void (T::*mptr)(void)) { 00100 _fall.attach(tptr, mptr); 00101 setup_interrupt(0, 1); 00102 } 00103 00104 /** Set the input pin mode 00105 * 00106 * @param mode PullUp, PullDown, PullNone 00107 */ 00108 void mode(PinMode pull); 00109 00110 static InterruptIn *_irq_objects[CHANNEL_NUM]; 00111 00112 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00113 static void _irq(); 00114 #elif defined(TARGET_LPC11U24) 00115 static void handle_interrupt_in(unsigned int channel); 00116 static void _irq0(); static void _irq1(); 00117 static void _irq2(); static void _irq3(); 00118 static void _irq4(); static void _irq5(); 00119 static void _irq6(); static void _irq7(); 00120 #endif 00121 00122 protected: 00123 PinName _pin; 00124 #if defined(TARGET_LPC11U24) 00125 Channel _channel; 00126 #endif 00127 FunctionPointer _rise; 00128 FunctionPointer _fall; 00129 00130 void setup_interrupt(int rising, int enable); 00131 00132 }; 00133 00134 } // namespace mbed 00135 00136 #endif 00137 00138 #endif
Generated on Tue Jul 12 2022 11:27:26 by
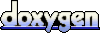