
public Test program for usbhostmsd looking at ridiculous resource usage
Dependencies: test_USBHost mbed
Fork of USBHostMSD_HelloWorld by
ramstats.cpp
00001 #include "ramstats.h" 00002 #include "mbed.h" 00003 #include <stdarg.h> 00004 00005 //extern USBSerial DebugComms; 00006 00007 //_sys_clock(); 00008 00009 /*int myfprintf(FILE *stream, const char *format, const char *arg1) { 00010 int done; 00011 const char *arg; 00012 concat(art1 00013 done=fprintf(stream,format,arg); 00014 return done; 00015 }*/ 00016 int myfprintf(FILE *stream, const char *format, ...) { 00017 va_list arg; 00018 int done; 00019 va_start (arg, format); 00020 done=vfprintf(stream,format,arg); 00021 va_end (arg); 00022 fprintf(stream,"\r"); 00023 fflush(stream); 00024 return done; 00025 } 00026 // Displays the size of static allocations for each RAM bank as indicated by 00027 // ARM linker to stdout. 00028 //static void DisplayRAMBanks(void) 00029 void DisplayRAMBanks(char * header) 00030 { 00031 //void __heapstats(int (*dprint)( void*param, char const *format,...), void* param); 00032 printf("\e[2J\e[1;33;44m %s",header); // clear the screen before starting 00033 printf("\r\n==== Heap Stats ====\r\n"); 00034 __heapstats((__heapprt)myfprintf,stdout); 00035 printf("\r\n==== Heap Valid ====\r\n"); 00036 __heapvalid((__heapprt)myfprintf,stdout, 1); 00037 printf("\r\n==== Current Stack Pointer ===="); 00038 printf("==== %10x ====\r\n\n",__current_sp()); 00039 00040 printf("Static RAM bank allocations\r\n"); 00041 printf(" Main RAM = %5u %04x\r\n", (unsigned int)&Image$$RW_IRAM1$$ZI$$Limit - (unsigned int)&Image$$RW_IRAM1$$Base, (unsigned int)&Image$$RW_IRAM1$$ZI$$Limit - (unsigned int)&Image$$RW_IRAM1$$Base); 00042 printf(" RAM0 = %5u %04x\r\n", (unsigned int)&Image$$RW_IRAM2$$ZI$$Limit - (unsigned int)&Image$$RW_IRAM2$$Base, (unsigned int)&Image$$RW_IRAM2$$ZI$$Limit - (unsigned int)&Image$$RW_IRAM2$$Base); 00043 printf(" RAM1 = %5u %04x\r\n", (unsigned int)&Image$$RW_IRAM3$$ZI$$Limit - (unsigned int)&Image$$RW_IRAM3$$Base, (unsigned int)&Image$$RW_IRAM3$$ZI$$Limit - (unsigned int)&Image$$RW_IRAM3$$Base); 00044 // printf("\r\n\nPress 'M' to resume"); 00045 printf("\r\n\n"); 00046 00047 } 00048 00049 00050 /* 00051 To move a global from main RAM to the RAM0/RAM1 bank, you can declare it something like: 00052 __attribute((section("AHBSRAM0"),aligned)) char LargeBuffer[1024]; 00053 __attribute((section("AHBSRAM1"),aligned)) char LargeBuffer[1024]; 00054 char bufA[10000] __attribute__((section("AHBSRAM1"))); 00055 00056 */ 00057 00058 00059 // *********************************************************************** 00060 // *********************************************************************** 00061 // *********************************************************************** 00062 // Examples of Alternative methods to capture and process this info.... 00063 // *********************************************************************** 00064 // *********************************************************************** 00065 // *********************************************************************** 00066 // the first two are from http://developer.mbed.org/forum/mbed/topic/2702/ 00067 /* 00068 #include <mbed.h> 00069 #include <stdarg.h> 00070 00071 int CaptureLine(void* pBuffer, char const* pFormatString, ...) 00072 { 00073 char* pStringEnd = (char*)pBuffer + strlen((char*)pBuffer); 00074 va_list valist; 00075 00076 va_start(valist, pFormatString); 00077 00078 return vsprintf(pStringEnd, pFormatString, valist); 00079 } 00080 00081 int main() 00082 { 00083 char OutputBuffer[256]; 00084 00085 printf("\r\nBefore malloc.\r\n"); 00086 OutputBuffer[0] = '\0'; 00087 __heapstats(CaptureLine, OutputBuffer); 00088 printf("%s", OutputBuffer); 00089 00090 void* pvTest = malloc(1024); 00091 00092 printf("After malloc.\r\n"); 00093 OutputBuffer[0] = '\0'; 00094 __heapstats(CaptureLine, OutputBuffer); 00095 printf("%s", OutputBuffer); 00096 00097 free(pvTest); 00098 00099 printf("After free.\r\n"); 00100 OutputBuffer[0] = '\0'; 00101 __heapstats(CaptureLine, OutputBuffer); 00102 printf("%s", OutputBuffer); 00103 00104 return 0; 00105 } 00106 */ 00107 00108 // *********************************************************************** 00109 // *********************************************************************** 00110 /* 00111 #include <mbed.h> 00112 #include <stdarg.h> 00113 00114 struct SHeapInfo 00115 { 00116 const char* HighestAllocBlock; 00117 const char* HighestFreeBlock; 00118 }; 00119 00120 int CaptureLine(void* pvHeapInfo, char const* pFormatString, ...) 00121 { 00122 static const char* pAllocFormatString = "alloc block %p size %3lx"; 00123 static const char* pFreeFormatString = "free block %p size %3lx next=%p"; 00124 static const char* pCompleteFormatString = "------- heap validation complete"; 00125 SHeapInfo* pHeapInfo = (SHeapInfo*)pvHeapInfo; 00126 va_list valist; 00127 00128 va_start(valist, pFormatString); 00129 00130 if (pFormatString == strstr(pFormatString, pAllocFormatString)) 00131 { 00132 const char* pBlock = va_arg(valist, const char*); 00133 unsigned long BlockSize = va_arg(valist, unsigned long); 00134 const char* pBlockLastByte = pBlock + BlockSize - 1; 00135 00136 if (pBlockLastByte > pHeapInfo->HighestAllocBlock) 00137 { 00138 pHeapInfo->HighestAllocBlock = pBlockLastByte; 00139 } 00140 } 00141 else if (pFormatString == strstr(pFormatString, pFreeFormatString)) 00142 { 00143 const char* pBlock = va_arg(valist, const char*); 00144 unsigned long BlockSize = va_arg(valist, unsigned long); 00145 const char* pBlockLastByte = pBlock + BlockSize - 1; 00146 00147 if (pBlockLastByte > pHeapInfo->HighestFreeBlock) 00148 { 00149 pHeapInfo->HighestFreeBlock = pBlockLastByte; 00150 } 00151 } 00152 else if (pFormatString == strstr(pFormatString, pCompleteFormatString)) 00153 { 00154 // Ignoring end of dump string. 00155 } 00156 else 00157 { 00158 // Unrecognized format string. 00159 printf("Unrecognized format of %s", pFormatString); 00160 } 00161 00162 return 1; 00163 } 00164 00165 void DisplayAndClearHeapInfo(SHeapInfo* pHeapInfo) 00166 { 00167 printf("Highest allocated block address: %p\r\n", pHeapInfo->HighestAllocBlock); 00168 printf("Highest free block address: %p\r\n", pHeapInfo->HighestFreeBlock); 00169 00170 memset(pHeapInfo, 0, sizeof(*pHeapInfo)); 00171 } 00172 00173 int main() 00174 { 00175 SHeapInfo HeapInfo = { 0, 0 }; 00176 00177 printf("\r\nBefore malloc.\r\n"); 00178 __heapvalid(CaptureLine, &HeapInfo, 1); 00179 DisplayAndClearHeapInfo(&HeapInfo); 00180 00181 void* pvTest = malloc(1024); 00182 00183 printf("After malloc.\r\n"); 00184 __heapvalid(CaptureLine, &HeapInfo, 1); 00185 DisplayAndClearHeapInfo(&HeapInfo); 00186 00187 free(pvTest); 00188 00189 printf("After free.\r\n"); 00190 __heapvalid(CaptureLine, &HeapInfo, 1); 00191 DisplayAndClearHeapInfo(&HeapInfo); 00192 00193 return 0; 00194 } 00195 */ 00196 00197 // *********************************************************************** 00198 // ***********************************************************************
Generated on Sat Jul 16 2022 21:37:10 by
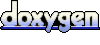