
Example driver for 4D Systems uOLED-160-G1 library
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "OLED160G1.h" 00003 00004 #define PI 3.1415926535897932384626433832795 00005 00006 OLED160G1 oled(p9, p10, p8); 00007 00008 int main() { 00009 oled.init(); 00010 oled.eraseScreen(); 00011 oled.setTextBackgroundType(OLED_SET_TEXT_OPAQUE); 00012 00013 oled.printf("\n4D Systems 160 x 128 OLED\nmbed driver"); 00014 00015 wait(2); 00016 00017 oled.drawLine(0, 0, 159, 127, oled.toRGB(0, 0, 255)); 00018 wait_ms(100); 00019 oled.drawLine(159, 0, 0, 127, oled.toRGB(0, 0, 255)); 00020 00021 wait(2); 00022 00023 for (int i = 0; i < 50; i += 1) { 00024 oled.drawCircle(80, 60, 3 * i, oled.toRGB(255, 0, 0)); 00025 wait_ms(50); 00026 } 00027 00028 for (int i = 0; i < 50; i += 1) { 00029 oled.drawCircle(80, 60, 3 * i, oled.toRGB(0, 0, 0)); 00030 wait_ms(50); 00031 } 00032 00033 wait(2); 00034 00035 oled.eraseScreen(); 00036 oled.displayControl(OLED_COMMAND_DISPLAY, 0); 00037 oled.displayControl(OLED_COMMAND_POWER, 0); 00038 00039 while (1) { 00040 00041 } 00042 } 00043
Generated on Thu Jul 28 2022 12:51:37 by
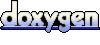