A device driver for the Freescale MPR121 capactive touch IC. Not optimized for any particular system, just a starting point to get the chip up in running in no time. Changes to registers init() method will tailor the library for end system use.
Dependents: Seeed_Grove_I2C_Touch_Example MPR121_HelloWorld mbed_petbottle_holder_shikake test_DEV-10508 ... more
MPR121 Class Reference
Using the Sparkfun SEN-10250 BoB. More...
#include <MPR121.h>
Public Types | |
enum | MPR121_ADDR { ADDR_VSS = 0x5A, ADDR_VDD, ADDR_SCL, ADDR_SDA } |
Possible terminations for the ADDR pin. More... | |
enum | MPR121_REGISTER |
The device register map. More... | |
Public Member Functions | |
MPR121 (I2C &i2c, InterruptIn &pin, MPR121_ADDR i2c_addr) | |
Create the MPR121 object. | |
void | init (void) |
Clear state variables and initilize the dependant objects. | |
void | enable (void) |
Allow the IC to run and collect user input. | |
void | disable (void) |
Stop the IC and put into low power mode. | |
uint32_t | isPressed (void) |
Determine if a new button press event occured Upon calling the state is cleared until another press is detected. | |
uint16_t | buttonPressed (void) |
Get the electrode status (ELE12 ... | |
void | registerDump (Serial &obj) const |
print the register map and values to the console | |
void | writeRegister (MPR121_REGISTER const reg, uint8_t const data) const |
Write to a register (exposed for debugging reasons) Note: most writes are only valid in stop mode. | |
uint8_t | readRegister (MPR121_REGISTER const reg) const |
Read from a register (exposed for debugging reasons) |
Detailed Description
Using the Sparkfun SEN-10250 BoB.
Example:
#include "mbed.h" #include "MPR121.h" Serial pc(USBTX, USBRX); DigitalOut myled(LED1); #if defined TARGET_LPC1768 || TARGET_LPC11U24 I2C i2c(p28, p27); InterruptIn irq(p26); MPR121 touch_pad(i2c, irq, MPR121::ADDR_VSS ); #elif defined TARGET_KL25Z I2C i2c(PTC9, PTC8); InterruptIn irq(PTA5); MPR121 touch_pad(i2c, irq, MPR121::ADDR_VSS ); #else #error TARGET NOT TESTED #endif int main() { touch_pad.init(); touch_pad.enable(); while(1) { if(touch_pad.isPressed()) { uint16_t button_val = touch_pad.buttonPressed(); printf("button = 0x%04x\n", button_val); myled = (button_val>0) ? 1 : 0; } } }
API for the MPR121 capacitive touch IC
Definition at line 74 of file MPR121.h.
Member Enumeration Documentation
enum MPR121_ADDR |
enum MPR121_REGISTER |
Constructor & Destructor Documentation
MPR121 | ( | I2C & | i2c, |
InterruptIn & | pin, | ||
MPR121_ADDR | i2c_addr | ||
) |
Create the MPR121 object.
- Parameters:
-
i2c - A defined I2C object pin - A defined InterruptIn object i2c_addr - Connection of the address line
Definition at line 28 of file MPR121.cpp.
Member Function Documentation
uint16_t buttonPressed | ( | void | ) |
Get the electrode status (ELE12 ...
ELE0 -> b15 xxx b11 ... b0 The buttons are bit mapped. ELE0 = b0 ... ELE11 = b11 b12 ... b15 undefined
- Returns:
- The state of all buttons
Definition at line 123 of file MPR121.cpp.
void disable | ( | void | ) |
Stop the IC and put into low power mode.
Definition at line 104 of file MPR121.cpp.
void enable | ( | void | ) |
Allow the IC to run and collect user input.
Definition at line 91 of file MPR121.cpp.
void init | ( | void | ) |
Clear state variables and initilize the dependant objects.
Definition at line 37 of file MPR121.cpp.
uint32_t isPressed | ( | void | ) |
Determine if a new button press event occured Upon calling the state is cleared until another press is detected.
- Returns:
- 1 if a press has been detected since the last call, 0 otherwise
Definition at line 118 of file MPR121.cpp.
uint8_t readRegister | ( | MPR121_REGISTER const | reg ) | const |
Read from a register (exposed for debugging reasons)
- Parameters:
-
reg - The register to read from
- Returns:
- The register contents
Definition at line 182 of file MPR121.cpp.
void registerDump | ( | Serial & | obj ) | const |
print the register map and values to the console
- Parameters:
-
obj - a Serial object that prints to a console
Definition at line 129 of file MPR121.cpp.
void writeRegister | ( | MPR121_REGISTER const | reg, |
uint8_t const | data | ||
) | const |
Write to a register (exposed for debugging reasons) Note: most writes are only valid in stop mode.
- Parameters:
-
reg - The register to be written data - The data to be written
Definition at line 167 of file MPR121.cpp.
Generated on Wed Jul 13 2022 12:22:31 by
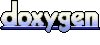