Device driver for the Freescale MMA845x family of accelerometers.
Dependents: MMA845x_test KL05_accel-test
MMA845x.h
00001 /** 00002 * @file MMA845x.h 00003 * @brief Device driver - MMA845x 3-axis accelerometer IC 00004 * @author sam grove 00005 * @version 1.0 00006 * @see http://cache.freescale.com/files/sensors/doc/data_sheet/MMA8451Q.pdf 00007 * @see http://cache.freescale.com/files/sensors/doc/data_sheet/MMA8452Q.pdf 00008 * @see http://cache.freescale.com/files/sensors/doc/data_sheet/MMA8453Q.pdf 00009 * 00010 * Copyright (c) 2013 00011 * 00012 * Licensed under the Apache License, Version 2.0 (the "License"); 00013 * you may not use this file except in compliance with the License. 00014 * You may obtain a copy of the License at 00015 * 00016 * http://www.apache.org/licenses/LICENSE-2.0 00017 * 00018 * Unless required by applicable law or agreed to in writing, software 00019 * distributed under the License is distributed on an "AS IS" BASIS, 00020 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00021 * See the License for the specific language governing permissions and 00022 * limitations under the License. 00023 */ 00024 00025 #ifndef MMA845X_H 00026 #define MMA845X_H 00027 00028 #include "mbed.h" 00029 00030 /** Using the Sparkfun SEN-10955 00031 * 00032 * Example: 00033 * @code 00034 * #include "mbed.h" 00035 * #include "MMA845x.h" 00036 * 00037 00038 * 00039 * int main() 00040 * { 00041 00042 * } 00043 * @endcode 00044 */ 00045 00046 00047 /** 00048 * @class MMA845x_DATA 00049 * @brief API abstraction for the MMA845x 3-axis accelerometer IC data 00050 */ 00051 class MMA845x_DATA 00052 { 00053 public: 00054 00055 volatile uint16_t _x ; /*!< volatile data variable */ 00056 volatile uint16_t _y ; /*!< volatile data variable */ 00057 volatile uint16_t _z ; /*!< volatile data variable */ 00058 00059 /** Create the MMA845x_DATA object initialized to the parameter (or 0 if none) 00060 * @param x - the init value of _x 00061 * @param y - the init value of _y 00062 * @param x - the init value of _z 00063 */ 00064 MMA845x_DATA(uint16_t x = 0, uint16_t y = 0, uint16_t z = 0) : _x (x), _y (y), _z (z) {} 00065 00066 /** Overloaded '=' operator to allow shorthand coding, assigning objects to one another 00067 * @param rhs - an object of the same type to assign ourself the same values of 00068 * @return this 00069 */ 00070 MMA845x_DATA &operator= (MMA845x_DATA const &rhs) 00071 { 00072 _x = rhs._x ; 00073 _y = rhs._y ; 00074 _z = rhs._z ; 00075 00076 return *this; 00077 } 00078 00079 /** Overloaded '=' operator to allow shorthand coding, assigning objects to one another 00080 * @param val - Assign each data member (_x, _y, _z) this value 00081 * @return this 00082 */ 00083 MMA845x_DATA &operator= (uint16_t const val) 00084 { 00085 _x = _y = _z = val; 00086 00087 return *this; 00088 } 00089 00090 /** Overloaded '==' operator to allow shorthand coding, test objects to one another 00091 * @param rhs - the object to compare against 00092 * @return 1 if the data members are the same and 0 otherwise 00093 */ 00094 bool operator== (MMA845x_DATA const &rhs) const 00095 { 00096 return ((_x == rhs._x )&&(_y == rhs._y )&&(_z == rhs._z )) ? 1 : 0; 00097 } 00098 00099 }; 00100 00101 /** 00102 * @class MMA845x 00103 * @brief API abstraction for the MMA845x 3-axis accelerometer IC 00104 */ 00105 class MMA845x 00106 { 00107 public: 00108 00109 /** 00110 * @enum MMA845x_SA0 00111 * @brief Possible terminations for the ADDR pin 00112 */ 00113 enum MMA845x_SA0 00114 { 00115 SA0_VSS = 0, /*!< SA0 connected to VSS */ 00116 SA0_VDD /*!< SA0 connected to VDD */ 00117 }; 00118 00119 /** 00120 * @enum MMA845x_WHO_AM_I 00121 * @brief Device ID's that this class is compatible with 00122 */ 00123 enum MMA845x_WHO_AM_I 00124 { 00125 MMA8451 = 0x1a, /*!< MMA8451 WHO_AM_I register content */ 00126 MMA8452 = 0x2a, /*!< MMA8452 WHO_AM_I register content */ 00127 MMA8453 = 0x3a, /*!< MMA8453 WHO_AM_I register content */ 00128 }; 00129 00130 /** 00131 * @enum MMA845x_REGISTER 00132 * @brief The device register map 00133 */ 00134 enum MMA845x_REGISTER 00135 { 00136 STATUS = 0x0, 00137 OUT_X_MSB, OUT_X_LSB, OUT_Y_MSB, OUT_Y_LSB, OUT_Z_MSB, OUT_Z_LSB, 00138 00139 F_SETUP = 0x9, TRIG_CFG, // only available on the MMA8451 variant 00140 00141 SYSMOD = 0xb, 00142 INT_SOURCE, WHO_AM_I, XYZ_DATA_CFG, HP_FILTER_CUTOFF, PL_STATUS, 00143 PL_CFG, PL_COUNT, PL_BF_ZCOMP, P_L_THS_REG, FF_MT_CFG, FF_MT_SRC, 00144 FF_MT_THS, FF_MT_COUNT, 00145 00146 TRANSIENT_CFG = 0x1d, 00147 TRANSIENT_SRC, TRANSIENT_THS, TRANSIENT_COUNT, PULSE_CFG, PULSE_SRC, 00148 PULSE_THSX, PULSE_THSY, PULSE_THSZ, PULSE_TMLT, PULSE_LTCY, PULSE_WIND, 00149 ASLP_COUNT, CTRL_REG1, CTRL_REG2, CTRL_REG3, CTRL_REG4, CTRL_REG5, 00150 OFF_X, OFF_Y, OFF_Z 00151 }; 00152 00153 /** Create the MMA845x object 00154 * @param i2c - A defined I2C object 00155 * @param int1 - A defined InterruptIn object 00156 * @param int2 - A defined InterruptIn object 00157 * @param i2c_addr - Connection of the address line 00158 */ 00159 MMA845x(I2C &i2c, InterruptIn &int1, InterruptIn &int2, MMA845x_SA0 const i2c_addr); 00160 00161 /** Get the X data 00162 * @return The last valid reading from the accelerometer 00163 */ 00164 uint16_t getX(void) const; 00165 00166 /** Get the Y data 00167 * @return The last valid reading from the accelerometer 00168 */ 00169 uint16_t getY(void) const; 00170 00171 /** Get the Z data 00172 * @return The last valid reading from the accelerometer 00173 */ 00174 uint16_t getZ(void) const; 00175 00176 /** Get the XYZ data structure 00177 * @return The last valid reading from the accelerometer 00178 */ 00179 MMA845x_DATA getXYZ(void) const; 00180 00181 void enableDataReadyMode(void) const; 00182 void enableMotionMode(void) const; 00183 void enablePulseMode(void) const; 00184 void enableOrientationMode(void) const; 00185 void enableTransitMode(void) const; 00186 void enableAutoSleepMode(void) const; 00187 void enableFIFOMode(void) const; 00188 00189 /** Put the MMA845x in the lowest possible power mode and suspend operation 00190 */ 00191 void disable(void); 00192 00193 /** Write to a register (exposed for debugging reasons) 00194 * Note: most writes are only valid in stop mode 00195 * @param reg - The register to be written 00196 * @param data - The data to be written 00197 */ 00198 void writeRegister(uint8_t const reg, uint8_t const data) const; 00199 00200 /** Read from a register (exposed for debugging reasons) 00201 * @param reg - The register to read from 00202 * @return The register contents 00203 */ 00204 uint8_t readRegister(uint8_t const reg) const; 00205 00206 /** print the register map and values to the console 00207 */ 00208 void registerDump(void) const; 00209 00210 private: 00211 00212 I2C *_i2c; 00213 InterruptIn *_int1; 00214 InterruptIn *_int2; 00215 uint8_t _i2c_addr; 00216 MMA845x_DATA _data; 00217 00218 void init(void) const; 00219 }; 00220 00221 #endif
Generated on Tue Jul 12 2022 13:55:36 by
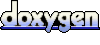