Device driver
Embed:
(wiki syntax)
Show/hide line numbers
Adis16488.h
Go to the documentation of this file.
00001 /** 00002 * @file Adis16488.h 00003 * @brief Device driver - ADIS16488 IMU 00004 * @author sam grove 00005 * @version 1.0 00006 * @see http://www.analog.com/static/imported-files/data_sheets/ADIS16488.pdf 00007 * 00008 * Copyright (c) 2013 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef ADIS16488_H 00024 #define ADIS16488_H 00025 00026 #include "LogUtil.h" 00027 #include "mbed.h" 00028 00029 /** Using the Analog Devices ADIS16488/PCBZ 00030 * 00031 * Example: 00032 * @code 00033 * #include "mbed.h" 00034 * 00035 * int main() 00036 * { 00037 * } 00038 * @endcode 00039 */ 00040 00041 /** 00042 * @class Adis16488 00043 * @brief API abstraction for the ADIS16488 IMU 00044 */ 00045 class Adis16488 00046 { 00047 public: 00048 00049 struct{ uint16_t data[6]; } gyro, accel, magn, deltang, deltvel; 00050 00051 /** Create the Adis16488 object 00052 * @param spi - A defined SPI object 00053 * @param cs - A defined DigitalOut object 00054 * @param rst - A defined DigitalOut object 00055 * @param dr - A defined InterruptIn object 00056 */ 00057 Adis16488(SPI &spi, DigitalOut &cs, DigitalOut &rst, InterruptIn &dr); 00058 00059 /** Clear state vars and initilize the dependant objects 00060 */ 00061 void init(void); 00062 00063 /** Read from a register (exposed for debugging reasons) 00064 * @param reg - The register to be written 00065 * @param data - Data read from the device is stored here 00066 */ 00067 void readRegister(uint16_t const reg, uint16_t &data); 00068 00069 /** Write to a register (exposed for debugging reasons) 00070 * @param reg - The register to be written 00071 */ 00072 void writeRegister(uint16_t const reg); 00073 00074 /** Allow the IC to run and collect user input 00075 */ 00076 void enable(void); 00077 00078 /** Stop the IC and put into low power mode 00079 */ 00080 void disable(void); 00081 00082 private: 00083 SPI *_spi; 00084 DigitalOut *_cs; 00085 DigitalOut *_rst; 00086 InterruptIn *_dr; 00087 00088 void drHandler(void); 00089 }; 00090 00091 #endif 00092
Generated on Fri Jul 15 2022 18:47:53 by
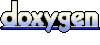