Handles reading and scaling the analog inputs from a simple 2-axis joystick with a pushbutton. Can also attach rise and fall interrupts to the button.
Dependents: joystickdeneme NOKIA_KOMBAT projekat
Joystick.h
00001 /* mbed Joystick Library 00002 * Copyright (c) 2015, rwunderl, http://mbed.org 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef MBED_JOYSTICK_H 00024 #define MBED_JOYSTICK_H 00025 00026 #include "mbed.h" 00027 00028 /** Joystick position structure 00029 * 00030 * Struct to keep track of the joystick x and y positions. 00031 */ 00032 struct joypos { 00033 double x; 00034 double y; 00035 }; 00036 00037 /** Joystick class 00038 * 00039 * Reads a 2-axis analog joystick and keeps track of its positions. 00040 * 00041 * The joystick uses 2 AnalogIns and 1 DigitalIn 00042 * - X AnalogIn 00043 * - Y AnalogIn 00044 * - A DigitalIn (momentary pushbutton; Low is pressed) 00045 */ 00046 class Joystick { 00047 public: 00048 /** Joystick interface 00049 * Create the joystick object and assign pins. 00050 * 00051 * @param x X-axis AnalogIn. 00052 * @param y Y-axis AnalogIn. 00053 * @param a Pushbutton DigitalIn. 00054 */ 00055 Joystick(PinName x, PinName y, PinName a); 00056 00057 /** Read the joystick position 00058 * The position is returned as a joypos value with x and y in the range [-1.0, 1.0]. 00059 * 00060 * @returns Position structure of the joystick. 00061 */ 00062 joypos read(); 00063 00064 /** Get the X position 00065 * Read the joystick's horizontal position, represented as a double value in the range [-1.0, 1.0]. 00066 * 00067 * @returns Horizontal position of the joystick. 00068 */ 00069 double getX(); 00070 00071 /** Get the Y position 00072 * Read the joystick's vertical position, represented as a double value in the range [-1.0, 1.0]. 00073 * 00074 * @returns Vertical position of the joystick. 00075 */ 00076 double getY(); 00077 00078 /** Get the raw X position 00079 * Read the joystick's raw horizontal position, represented as a double value in the range [0.0, 1.0]. 00080 * 00081 * @returns Horizontal position of the joystick. 00082 */ 00083 double getRawX(); 00084 00085 /** Get the raw Y position 00086 * Read the joystick's raw vertical position, represented as a double value in the range [0.0, 1.0]. 00087 * 00088 * @returns Vertical position of the joystick. 00089 */ 00090 double getRawY(); 00091 00092 /** Attach the rise interrupt 00093 * Attach a function pointer to call when a rising edge occurs on the button input. 00094 * 00095 * @param fptr Pointer to a void function. Set to NULL or 0 for none. 00096 */ 00097 void rise(void (*fptr)(void)); 00098 00099 /** Attach the fall interrupt 00100 * Attach a function pointer to call when a falling edge occurs on the button input. 00101 * 00102 * @param fptr Pointer to a void function. Set to NULL or 0 for none. 00103 */ 00104 void fall(void (*fptr)(void)); 00105 00106 protected: 00107 AnalogIn _x; 00108 AnalogIn _y; 00109 InterruptIn _a; 00110 }; 00111 00112 #endif /* MBED_JOYSTICK_H */
Generated on Tue Jul 12 2022 18:44:08 by
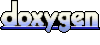