Handles reading and scaling the analog inputs from a simple 2-axis joystick with a pushbutton. Can also attach rise and fall interrupts to the button.
Dependents: joystickdeneme NOKIA_KOMBAT projekat
Joystick.cpp
00001 /* mbed Joystick Library 00002 * Copyright (c) 2015, rwunderl, http://mbed.org 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #include "Joystick.h" 00024 00025 // apply the deadzone to the axis extremes (comment if you don't want to use this feature) 00026 #define JOYSTICK_DEADZONE_EXTREMES 00027 00028 // adjust the deadzone value for your application, if required 00029 const double JOYSTICK_DEADZONE = 0.01; 00030 // set up these constants so they don't have to be calculated every time 00031 #ifdef JOYSTICK_DEADZONE_EXTREMES 00032 const double JOYSTICK_MIN = 0.0 + JOYSTICK_DEADZONE; // effective minimum joystick value 00033 const double JOYSTICK_MINMID = 0.5 - JOYSTICK_DEADZONE; // minimum-side midpoint value 00034 const double JOYSTICK_MAXMID = 0.5 + JOYSTICK_DEADZONE; // maximum-side midpoint value 00035 const double JOYSTICK_MAX = 1.0 - JOYSTICK_DEADZONE; // effective maximum joystick value 00036 #else 00037 const double JOYSTICK_MIN = 0.0; // minimum joystick value 00038 const double JOYSTICK_MINMID = 0.5 - JOYSTICK_DEADZONE; // minimum-side midpoint value 00039 const double JOYSTICK_MAXMID = 0.5 + JOYSTICK_DEADZONE; // maximum-side midpoint value 00040 const double JOYSTICK_MAX = 1.0; // maximum joystick value 00041 #endif 00042 00043 double _map(double value, double in_min, double in_max, double out_min, double out_max) { 00044 return (value - in_min) * (out_max - out_min) / (in_max - in_min) + out_min; 00045 } 00046 00047 double _scale(double pos) { 00048 double scaled = 0; 00049 00050 if (pos < JOYSTICK_MINMID) { 00051 scaled = _map(pos, JOYSTICK_MIN, JOYSTICK_MINMID, -1, 0); 00052 } else if (pos > JOYSTICK_MAXMID) { 00053 scaled = _map(pos, JOYSTICK_MAXMID, JOYSTICK_MAX, 0, 1); 00054 } 00055 00056 // check out of bounds [-1.0, 1.0] (happens at the extremes) 00057 if (scaled < -1) { 00058 scaled = -1; 00059 } else if (scaled > 1) { 00060 scaled = 1; 00061 } 00062 00063 return scaled; 00064 } 00065 00066 Joystick::Joystick(PinName x, PinName y, PinName a) : _x(x), _y(y), _a(a) { 00067 } 00068 00069 joypos Joystick::read() { 00070 joypos pos; 00071 00072 pos.x = getX(); 00073 pos.y = getY(); 00074 00075 return pos; 00076 } 00077 00078 double Joystick::getX() { 00079 double val = _x.read(); 00080 val = _scale(val); 00081 return val; 00082 } 00083 00084 double Joystick::getY() { 00085 double val = _y.read(); 00086 val = _scale(val); 00087 return val; 00088 } 00089 00090 double Joystick::getRawX() { 00091 return _x.read(); 00092 } 00093 00094 double Joystick::getRawY() { 00095 return _y.read(); 00096 } 00097 00098 void Joystick::rise(void (*fptr)(void)) { 00099 _a.rise(fptr); 00100 } 00101 00102 void Joystick::fall(void (*fptr)(void)) { 00103 _a.fall(fptr); 00104 }
Generated on Tue Jul 12 2022 18:44:08 by
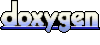