
konashi/SBBLEのテスト
Fork of BLE_LoopbackUART by
Embed:
(wiki syntax)
Show/hide line numbers
nRF51822n.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "nRF51822n.h" 00019 #include "nrf_soc.h" 00020 00021 #include "btle/btle.h" 00022 00023 /** 00024 * The singleton which represents the nRF51822 transport for the BLEDevice. 00025 */ 00026 static nRF51822n deviceInstance; 00027 00028 /** 00029 * BLE-API requires an implementation of the following function in order to 00030 * obtain its transport handle. 00031 */ 00032 BLEDeviceInstanceBase * 00033 createBLEDeviceInstance(void) 00034 { 00035 return (&deviceInstance); 00036 } 00037 00038 /**************************************************************************/ 00039 /*! 00040 @brief Constructor 00041 */ 00042 /**************************************************************************/ 00043 nRF51822n::nRF51822n(void) 00044 { 00045 } 00046 00047 /**************************************************************************/ 00048 /*! 00049 @brief Destructor 00050 */ 00051 /**************************************************************************/ 00052 nRF51822n::~nRF51822n(void) 00053 { 00054 } 00055 00056 const char *nRF51822n::getVersion(void) 00057 { 00058 static char versionString[10]; 00059 static bool versionFetched = false; 00060 00061 if (!versionFetched) { 00062 ble_version_t version; 00063 if (sd_ble_version_get(&version) == NRF_SUCCESS) { 00064 snprintf(versionString, sizeof(versionString), "%u.%u", version.version_number, version.subversion_number); 00065 versionFetched = true; 00066 } else { 00067 strncpy(versionString, "unknown", sizeof(versionString)); 00068 } 00069 } 00070 00071 return versionString; 00072 } 00073 00074 /* (Valid values are -40, -20, -16, -12, -8, -4, 0, 4) */ 00075 ble_error_t nRF51822n::setTxPower(int8_t txPower) 00076 { 00077 unsigned rc; 00078 if ((rc = sd_ble_gap_tx_power_set(txPower)) != NRF_SUCCESS) { 00079 switch (rc) { 00080 case NRF_ERROR_BUSY: 00081 return BLE_STACK_BUSY; 00082 case NRF_ERROR_INVALID_PARAM: 00083 default: 00084 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00085 } 00086 } 00087 00088 return BLE_ERROR_NONE; 00089 } 00090 00091 /**************************************************************************/ 00092 /*! 00093 @brief Initialises anything required to start using BLE 00094 00095 @returns ble_error_t 00096 00097 @retval BLE_ERROR_NONE 00098 Everything executed properly 00099 00100 @section EXAMPLE 00101 00102 @code 00103 00104 @endcode 00105 */ 00106 /**************************************************************************/ 00107 ble_error_t nRF51822n::init(void) 00108 { 00109 /* ToDo: Clear memory contents, reset the SD, etc. */ 00110 btle_init(); 00111 00112 reset(); 00113 00114 return BLE_ERROR_NONE; 00115 } 00116 00117 /**************************************************************************/ 00118 /*! 00119 @brief Resets the BLE HW, removing any existing services and 00120 characteristics 00121 00122 @returns ble_error_t 00123 00124 @retval BLE_ERROR_NONE 00125 Everything executed properly 00126 00127 @section EXAMPLE 00128 00129 @code 00130 00131 @endcode 00132 */ 00133 /**************************************************************************/ 00134 ble_error_t nRF51822n::reset(void) 00135 { 00136 wait(0.5); 00137 00138 /* Wait for the radio to come back up */ 00139 wait(1); 00140 00141 return BLE_ERROR_NONE; 00142 } 00143 00144 void 00145 nRF51822n::waitForEvent(void) 00146 { 00147 sd_app_evt_wait(); 00148 }
Generated on Tue Jul 12 2022 20:35:46 by
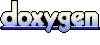