4x4 Keypad easy to use library that pollis the interface width pullups
Dependents: 4x4KeyBoardExample xoxokey 4x4KeyBoardExample ProgettoCassaforte ... more
Fork of keypad by
Keypad Class Reference
An simple polling-based interface to read a 4x4 keypad. More...
#include <keypad.h>
Public Member Functions | |
Keypad (PinName col0, PinName col1, PinName col2, PinName col3, PinName row0, PinName row1, PinName row2, PinName row3) | |
Create a 4x4 (col, row) or 4x4 keypad interface . | |
char | getKey () |
Returns the letter of the pressed key . | |
bool | getKeyPressed () |
Detects if any key was pressed. | |
void | enablePullUp () |
Enables internal PullUp resistors on the coloums pins. | |
Protected Member Functions | |
int | getKeyIndex () |
return the index value representating the pressed key |
Detailed Description
An simple polling-based interface to read a 4x4 keypad.
The function getKey() reads the index of the pressed key and returns the letter of the pressed key
This work is a derivative of the works done by: Dimiter Kentri in 2010 https://developer.mbed.org/users/DimiterK/code/keypad/ and Yoong Hor Meng in 2012 https://developer.mbed.org/users/yoonghm/code/keypad/
Example:
#include "mbed.h" #include "keypad.h" Serial pc(USBTX, USBRX); Keypad keypad(D3,D4,D5,D6,D7,D8,D9,D10); // Keypad keypad( PC_3,PC_2,PA_0,PA_1,PA_4,PB_0,PC_1,PC_0 ); // Tested on Nucleo303RE card int main(void) { keypad.enablePullUp(); char key; pc.printf("Please touch a key on the keypad\r\n"); while(1) { key = keypad.getKey(); if(key != KEY_RELEASED) { pc.printf("%c\r\n",key); wait(0.2); } } }
Definition at line 82 of file keypad.h.
Constructor & Destructor Documentation
Keypad | ( | PinName | col0, |
PinName | col1, | ||
PinName | col2, | ||
PinName | col3, | ||
PinName | row0, | ||
PinName | row1, | ||
PinName | row2, | ||
PinName | row3 | ||
) |
Create a 4x4 (col, row) or 4x4 keypad interface
.
| Col0 | Col1 | Col2 | Col3
-------+------+------+------+-----
Row 0 | x | x | x | x
Row 1 | x | x | x | x
Row 2 | x | x | x | x
Row 3 | x | x | x | x
- Parameters:
-
col<0..3> Row data lines row<0..3> Column data lines
Definition at line 32 of file keypad.cpp.
Member Function Documentation
void enablePullUp | ( | ) |
Enables internal PullUp resistors on the coloums pins.
- Returns:
- void
Definition at line 36 of file keypad.cpp.
char getKey | ( | ) |
Returns the letter of the pressed key
.
- Returns:
- char
-
The pressed character
'\0' or NO_KEY if no keys was pressed
Definition at line 65 of file keypad.cpp.
int getKeyIndex | ( | ) | [protected] |
return the index value representating the pressed key
- Returns:
- int
-
The index representing the pushed key used in table keys
-1 if no key was pressed
Definition at line 50 of file keypad.cpp.
bool getKeyPressed | ( | ) |
Detects if any key was pressed.
- Returns:
- bool
- Return values:
-
true a key is pressed false no keys was not pressed
Definition at line 41 of file keypad.cpp.
Generated on Tue Jul 12 2022 11:29:00 by
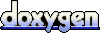