This driver is meant for the monochrome LCD display (model no: LS013B4DN04) from Sharp; but it should be easily adaptable to other Sharp displays.
SharpLCD Class Reference
This driver is meant for the monochrome LCD display (model no: LS013B4DN04) from Sharp. More...
#include <SharpLCD.hpp>
Public Member Functions | |
void | enableDisplay (void) |
Turn on the LCD's display. | |
void | disableDisplay (void) |
Turn off the LCD's display---i.e. | |
void | clear (void) |
Clear the LCD's display. | |
void | drawFrameBuffer (const FrameBuffer &fb) |
Update LCD using a given framebuffer. | |
void | toggleVCOM (void) |
Toggle the VCOM mode of the LCD; it is recommended to trigger this periodically. | |
Static Public Attributes | |
static const unsigned | LCD_WIDTH = 96 |
Constant defining the LCD's geometry. | |
static const unsigned | LCD_HEIGHT = 96 |
Constant defining the LCD's geometry. |
Detailed Description
This driver is meant for the monochrome LCD display (model no: LS013B4DN04) from Sharp.
The LCD has the following pixel dimensions: width=96pixels, height=96pixels. This is a monochrome display with an inbuilt memory of 1 bit per pixel. If a pixel-bit is set to one, the corresponding pixel will show as black.
The LCD memory is accessible to the micro-controller only through a serial interface; and only for write operations. It is necessary for the application to maintain its own frame-buffer memory in the micro-controller's SRAM (see fb_alloc())---the application is not restricted to a single framebuffer; if SRAM size permits, multiple buffers may be employed. In order to update the LCD, the application first draws (bitmaps or text) into some framebuffer memory, and then flushes the framebuffer to the LCD over the serial interface.
Here's some sample code to drive the LCD display:
DigitalOut led1(LED1); SharpLCD lcd(p9, MBED_SPI0);
uint8_t framebuffer[SharpLCD::SIZEOF_FRAMEBUFFER_FOR_ALLOC];
int main(void) { SharpLCD::FrameBuffer fb(framebuffer);
lcd.enableDisplay(); lcd.clear(); fb.printString(lookupFontFace("DejaVu Serif", 8), 20, 40, BLACK, "Rohit"); lcd.drawFrameBuffer(fb);
led1 = 1; while (true) { wait(0.5); led1 = !led1; } }
Definition at line 71 of file SharpLCD.hpp.
Member Function Documentation
void clear | ( | void | ) |
Clear the LCD's display.
Write all-white to the LCD's memory. If a frameBuffer is passed in then it is re-initialized as well; otherwise this function does not operate on any global frame-buffer and updating any application-specific frameBuffer is still the application's responsibility.
Definition at line 30 of file SharpLCD.cpp.
void disableDisplay | ( | void | ) |
Turn off the LCD's display---i.e.
make it go blank.
- Note:
- The LCD will retain its memory even when the display is disabled.
This is different from re-initializing the LCD's display, since it does not affect the LCD memory. When the display is re-enabled, the LCD will show the contents of its memory.
Definition at line 358 of file SharpLCD.hpp.
void drawFrameBuffer | ( | const FrameBuffer & | fb ) |
Update LCD using a given framebuffer.
The entire contents of the framebuffer will be DMA'd to the LCD; the calling thread will loose the CPU during the transfer, but other threads may remain active in that duration.
- Parameters:
-
[in] fb The frame buffer to send to the LCD hardware.
Definition at line 38 of file SharpLCD.cpp.
void enableDisplay | ( | void | ) |
Turn on the LCD's display.
- Note:
- Updates to the LCD's memory won't show up on the display until the display is enabled through this function.
Definition at line 353 of file SharpLCD.hpp.
void toggleVCOM | ( | void | ) |
Toggle the VCOM mode of the LCD; it is recommended to trigger this periodically.
Check the datasheet.
Definition at line 44 of file SharpLCD.cpp.
Field Documentation
const unsigned LCD_HEIGHT = 96 [static] |
Constant defining the LCD's geometry.
Definition at line 328 of file SharpLCD.hpp.
const unsigned LCD_WIDTH = 96 [static] |
Constant defining the LCD's geometry.
Definition at line 327 of file SharpLCD.hpp.
Generated on Tue Jul 12 2022 19:17:11 by
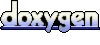