This driver is meant for the monochrome LCD display (model no: LS013B4DN04) from Sharp; but it should be easily adaptable to other Sharp displays.
SharpLCD.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "SharpLCD.hpp" 00018 00019 void 00020 SharpLCD::writeBuffer(const uint8_t *buffer, unsigned len) 00021 { 00022 chipSelect = 1; 00023 while (len--) { 00024 spi.write(*buffer++); 00025 } 00026 chipSelect = 0; 00027 } 00028 00029 void 00030 SharpLCD::clear(void) 00031 { 00032 const uint8_t buf[2] = {M2_FLAG, DUMMY8}; 00033 00034 writeBuffer(buf, sizeof(buf)); 00035 } 00036 00037 void 00038 SharpLCD::drawFrameBuffer(const FrameBuffer &fb) 00039 { 00040 writeBuffer(fb.getBuffer(), SIZEOF_FRAMEBUFFER_FOR_ALLOC); 00041 } 00042 00043 void 00044 SharpLCD::toggleVCOM(void) 00045 { 00046 static bool frameInversion = false; 00047 uint8_t buf[2] = {0, DUMMY8}; 00048 00049 uint8_t mode = 0x0; 00050 if (frameInversion) { 00051 mode |= M1_FLAG; 00052 } 00053 frameInversion = !frameInversion; /* toggle frameInversion in 00054 * preparation for the next call */ 00055 00056 buf[0] = mode; 00057 writeBuffer(buf, sizeof(buf)); 00058 }
Generated on Tue Jul 12 2022 19:17:11 by
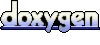