
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "lwip/dhcp.h" 00003 #include "lwip/tcpip.h" 00004 #include "lwip/api.h" 00005 #include "eth_arch.h" 00006 00007 //#define USE_DHCP /* enable DHCP, if disabled static address is used*/ 00008 00009 /*Static IP ADDRESS*/ 00010 #define IP_ADDR0 192 00011 #define IP_ADDR1 168 00012 #define IP_ADDR2 0 00013 #define IP_ADDR3 10 00014 00015 /*NETMASK*/ 00016 #define NETMASK_ADDR0 255 00017 #define NETMASK_ADDR1 255 00018 #define NETMASK_ADDR2 255 00019 #define NETMASK_ADDR3 0 00020 00021 /*Gateway Address*/ 00022 #define GW_ADDR0 192 00023 #define GW_ADDR1 168 00024 #define GW_ADDR2 0 00025 #define GW_ADDR3 1 00026 00027 #define DHCP_OFF (uint8_t) 0 00028 #define DHCP_START (uint8_t) 1 00029 #define DHCP_WAIT_ADDRESS (uint8_t) 2 00030 #define DHCP_ADDRESS_ASSIGNED (uint8_t) 3 00031 #define DHCP_TIMEOUT_APP (uint8_t) 4 00032 #define DHCP_LINK_DOWN (uint8_t) 5 00033 00034 #ifdef USE_DHCP 00035 #define MAX_DHCP_TRIES 4 00036 __IO uint8_t DHCP_state = DHCP_OFF; 00037 #endif 00038 00039 struct netif gnetif; /* network interface structure */ 00040 00041 DigitalOut led1(LED1); 00042 DigitalOut led3(LED3); 00043 00044 Thread thread1; 00045 00046 static void Netif_Config(void); 00047 void User_notification(struct netif *netif); 00048 #ifdef USE_DHCP 00049 void DHCP_thread(void); 00050 #endif 00051 static void http_server_netconn_thread(void *arg); 00052 00053 int main() 00054 { 00055 printf("\n\n*** Welcome STM32F767ZI !!! ***\n\r"); 00056 printf("CLOCK: %d\n\r", OS_Tick_GetClock()); 00057 00058 /* Create tcp_ip stack thread */ 00059 tcpip_init(NULL, NULL); 00060 00061 /* Initialize the LwIP stack */ 00062 Netif_Config(); 00063 00064 #ifdef USE_DHCP 00065 /* Start DHCPClient */ 00066 thread1.start(DHCP_thread); 00067 #endif 00068 00069 sys_thread_new("HTTP", http_server_netconn_thread, NULL, DEFAULT_THREAD_STACKSIZE, osPriorityAboveNormal); 00070 00071 /* Notify user about the network interface config */ 00072 User_notification(&gnetif); 00073 00074 while (true) { 00075 00076 00077 } 00078 } 00079 00080 00081 /** 00082 * @brief Initializes the lwIP stack 00083 * @param None 00084 * @retval None 00085 */ 00086 static void Netif_Config(void) 00087 { 00088 ip_addr_t ipaddr; 00089 ip_addr_t netmask; 00090 ip_addr_t gw; 00091 00092 #ifdef USE_DHCP 00093 ip_addr_set_zero_ip4(&ipaddr); 00094 ip_addr_set_zero_ip4(&netmask); 00095 ip_addr_set_zero_ip4(&gw); 00096 #else 00097 IP_ADDR4(&ipaddr,IP_ADDR0,IP_ADDR1,IP_ADDR2,IP_ADDR3); 00098 IP_ADDR4(&netmask,NETMASK_ADDR0,NETMASK_ADDR1,NETMASK_ADDR2,NETMASK_ADDR3); 00099 IP_ADDR4(&gw,GW_ADDR0,GW_ADDR1,GW_ADDR2,GW_ADDR3); 00100 #endif /* USE_DHCP */ 00101 00102 /* add the network interface */ 00103 netif_add(&gnetif, &ipaddr, &netmask, &gw, NULL, ð_arch_enetif_init, &tcpip_input); 00104 00105 /* Registers the default network interface. */ 00106 netif_set_default(&gnetif); 00107 00108 if (netif_is_link_up(&gnetif)) 00109 { 00110 /* When the netif is fully configured this function must be called.*/ 00111 netif_set_up(&gnetif); 00112 printf("\n\rEth Configured\n\r"); 00113 } 00114 else 00115 { 00116 /* When the netif link is down this function must be called */ 00117 netif_set_down(&gnetif); 00118 printf("\n\rLink is down\n\r"); 00119 } 00120 00121 } 00122 00123 00124 /** 00125 * @brief Notify the User about the network interface config status 00126 * @param netif: the network interface 00127 * @retval None 00128 */ 00129 void User_notification(struct netif *netif) 00130 { 00131 if (netif_is_up(netif)) 00132 { 00133 #ifdef USE_DHCP 00134 /* Update DHCP state machine */ 00135 DHCP_state = DHCP_START; 00136 #else 00137 /* Turn On LED 1 to indicate ETH and LwIP init success*/ 00138 led1 = 1; 00139 #endif /* USE_DHCP */ 00140 } 00141 else 00142 { 00143 #ifdef USE_DHCP 00144 /* Update DHCP state machine */ 00145 DHCP_state = DHCP_LINK_DOWN; 00146 #endif /* USE_DHCP */ 00147 /* Turn On LED 3 to indicate ETH and LwIP init error */ 00148 led3 = 1; 00149 } 00150 } 00151 00152 00153 #ifdef USE_DHCP 00154 /** 00155 * @brief DHCP Process 00156 * @param argument: network interface 00157 * @retval None 00158 */ 00159 void DHCP_thread(void) 00160 { 00161 struct netif *netif = &gnetif; 00162 ip_addr_t ipaddr; 00163 ip_addr_t netmask; 00164 ip_addr_t gw; 00165 struct dhcp *dhcp; 00166 00167 for (;;) 00168 { 00169 switch (DHCP_state) 00170 { 00171 case DHCP_START: 00172 { 00173 ip_addr_set_zero_ip4(&netif->ip_addr); 00174 ip_addr_set_zero_ip4(&netif->netmask); 00175 ip_addr_set_zero_ip4(&netif->gw); 00176 dhcp_start(netif); 00177 DHCP_state = DHCP_WAIT_ADDRESS; 00178 } 00179 break; 00180 00181 case DHCP_WAIT_ADDRESS: 00182 { 00183 if (dhcp_supplied_address(netif)) 00184 { 00185 DHCP_state = DHCP_ADDRESS_ASSIGNED; 00186 00187 led3 = 0; 00188 led1 = 1; 00189 00190 printf("\n\rSTM32 IP: %d.%d.%d.%d\n\r",(((const u8_t*)(&(ipaddr).addr))[0]),(((const u8_t*)(&(ipaddr).addr))[1]), 00191 (((const u8_t*)(&(ipaddr).addr))[2]),(((const u8_t*)(&(ipaddr).addr))[3])); 00192 } 00193 else 00194 { 00195 dhcp = (struct dhcp *)netif_get_client_data(netif, LWIP_NETIF_CLIENT_DATA_INDEX_DHCP); 00196 00197 /* DHCP timeout */ 00198 if (dhcp->tries > MAX_DHCP_TRIES) 00199 { 00200 DHCP_state = DHCP_TIMEOUT_APP; 00201 00202 /* Stop DHCP */ 00203 dhcp_stop(netif); 00204 00205 /* Static address used */ 00206 IP_ADDR4(&ipaddr, IP_ADDR0 ,IP_ADDR1 , IP_ADDR2 , IP_ADDR3 ); 00207 IP_ADDR4(&netmask, NETMASK_ADDR0, NETMASK_ADDR1, NETMASK_ADDR2, NETMASK_ADDR3); 00208 IP_ADDR4(&gw, GW_ADDR0, GW_ADDR1, GW_ADDR2, GW_ADDR3); 00209 netif_set_addr(netif, ip_2_ip4(&ipaddr), ip_2_ip4(&netmask), ip_2_ip4(&gw)); 00210 00211 led3 = 0; 00212 led1 = 1; 00213 } 00214 else 00215 { 00216 led3 = 1; 00217 } 00218 } 00219 } 00220 break; 00221 00222 case DHCP_LINK_DOWN: 00223 { 00224 /* Stop DHCP */ 00225 dhcp_stop(netif); 00226 DHCP_state = DHCP_OFF; 00227 } 00228 break; 00229 00230 default: break; 00231 00232 } 00233 00234 /* wait 250 ms */ 00235 wait_ms(250); 00236 } 00237 } 00238 #endif /* USE_DHCP */ 00239 00240 00241 /** 00242 * @brief Notify the User about the network interface config status 00243 * @param netif: the network interface 00244 * @retval None 00245 */ 00246 static void http_server_netconn_thread(void *arg) 00247 { 00248 struct netconn *conn, *newconn; 00249 err_t err, accept_err; 00250 00251 /* Create a new TCP connection handle */ 00252 conn = netconn_new(NETCONN_TCP); 00253 00254 if (conn!= NULL) 00255 { 00256 /* Bind to port 80 (HTTP) with default IP address */ 00257 err = netconn_bind(conn, NULL, 80); 00258 00259 if (err == ERR_OK) 00260 { 00261 /* Put the connection into LISTEN state */ 00262 netconn_listen(conn); 00263 00264 while(1) 00265 { 00266 /* accept any icoming connection */ 00267 accept_err = netconn_accept(conn, &newconn); 00268 if(accept_err == ERR_OK) 00269 { 00270 /* serve connection */ 00271 // Write your code here 00272 printf("\n\rNew HTTP Connection"); 00273 00274 /* delete connection */ 00275 netconn_delete(newconn); 00276 } 00277 } 00278 } 00279 } 00280 }
Generated on Tue Jul 12 2022 14:48:52 by
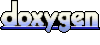