This is a VERY low-level library for controlling the TSI hardware module in a KL25 microcontroller. The programmer creates the TSI object passing basic parameters, and selects the active channels. Then, a scan on a given channel can be started, and the raw result can be read.
TSIHW.h
00001 #ifndef TSIHW_H 00002 #define TSIHW_H 00003 00004 #include "mbed.h" 00005 /** 00006 * KL25 TSI low-level library with very basic functions 00007 * 00008 * @code 00009 * #include "mbed.h" 00010 * #include "TSIHW.h" 00011 * 00012 * TSI touch(0,0,0,2,2); 00013 * Serial pc(USBTX, USBRX); 00014 * 00015 * int main() { 00016 * uint16_t s; 00017 * 00018 * pc.printf("BEGIN\r\n"); 00019 * touch.ActivateChannel(5); // Electrode connected to PTA4 00020 * pc.printf("ACTIVATE\r\n"); 00021 * while(1) { 00022 * s = 0; 00023 * touch.Start(5); 00024 * pc.printf("START\r\n"); 00025 * while(!s) { 00026 * s = touch.Read(); 00027 * } 00028 * pc.printf("TOUCH: %d\r\n", s); 00029 * wait(1); 00030 * } 00031 * } 00032 * @endcode 00033 */ 00034 00035 00036 class TSI 00037 { 00038 public: 00039 TSI(char rchg, char echg, char dvolt, char ps, char nscn); 00040 00041 ~TSI(); 00042 00043 void ActivateChannel(char ch); 00044 00045 void Start(char ch); 00046 00047 uint16_t Read(void); 00048 00049 }; 00050 #endif
Generated on Thu Jul 14 2022 13:17:10 by
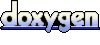