Smart coffee machine with facial recognition and remote control
Dependencies: Camera_LS_Y201 EthernetInterface EthernetNetIf HTTPClient SRF05 TextLCD mbed-rtos mbed-src
ethernet.cpp
00001 #include "ethernet.h" 00002 00003 //const char* ECHO_SERVER_ADDRESS = "194.254.15.213/reco/"; 00004 const char* ECHO_SERVER_ADDRESS = "192.168.1.40"; 00005 const int ECHO_SERVER_PORT = 4242; 00006 00007 EthernetInterface eth; 00008 TCPSocketConnection socket; 00009 HTTPClient http; 00010 int timeOut = HTTP_CLIENT_DEFAULT_TIMEOUT; 00011 00012 bool preparationEthernet() 00013 { 00014 afficherAuCentreDeLEcran("Preparation :", "Ethernet (1/2)"); 00015 wait(0.5); 00016 00017 if(eth.init() == 0) 00018 //if( eth.init("192.168.1.67", "255.255.255.0", ECHO_SERVER_ADDRESS) == 0) // Test en local (Sans DHCP) 00019 { 00020 afficherAuCentreDeLEcran("Preparation :", "Ethernet (2/2)"); 00021 00022 if(eth.connect() == 0) 00023 { 00024 afficherAuCentreDeLEcran("Machine", "connectee"); 00025 allumerLed(1); 00026 return true; 00027 } 00028 00029 else 00030 afficherAuCentreDeLEcran("Erreur :", "Ethernet (2/2)"); 00031 } 00032 00033 else 00034 afficherAuCentreDeLEcran("Erreur :", "Ethernet (1/2)"); 00035 00036 wait(2); 00037 afficherAuCentreDeLEcran("Err ethernet", eth.getMACAddress()); 00038 00039 allumerLed(2); 00040 return false; 00041 } 00042 00043 void deconnexionEthernet() 00044 { 00045 eth.disconnect(); 00046 afficherAuCentreDeLEcran("Machine", "deconnectee"); 00047 } 00048 00049 bool connexionSocket() 00050 { 00051 /*int i = 0; 00052 while (socket.connect(ECHO_SERVER_ADDRESS, ECHO_SERVER_PORT) < 0) 00053 { 00054 printf("Unable to connect to (%s) on port (%d)\r\n\r", ECHO_SERVER_ADDRESS, ECHO_SERVER_PORT); 00055 wait(1); 00056 i++; 00057 if(i>5) 00058 { 00059 return 0; // Échec 00060 } 00061 } 00062 return 1;*/ 00063 for(int i = 0 ; i < 4 ; i ++) 00064 { 00065 if(socket.connect(ECHO_SERVER_ADDRESS, ECHO_SERVER_PORT) < 0) 00066 { 00067 afficherAuCentreDeLEcran("Serveur", "injoignable", "sur", ECHO_SERVER_ADDRESS); 00068 wait(3); 00069 } 00070 00071 else 00072 return true; 00073 } 00074 00075 return false; 00076 } 00077 00078 void deconnexionSocket() 00079 { 00080 socket.close(); 00081 } 00082 00083 bool envoyerRequete(char complement_url[200], char *reponse, int longueur_reponse) 00084 { 00085 char url[500]; 00086 sprintf(url, "http://asi-12-cafetiere.insa-rouen.fr/mbed/%s", complement_url); 00087 //sprintf(url, "http://192.168.1.10/mbed/%s", complement_url); 00088 HTTPResult retour = http.get(url, reponse, longueur_reponse); 00089 00090 if(retour != HTTP_OK) 00091 { 00092 afficherAuCentreDeLEcran("ECHEC", "Envoi requete"); 00093 wait(1); 00094 } 00095 00096 return retour == HTTP_OK; // Succès 00097 } 00098 00099 bool envoyerRequete(char complement_url[200]) 00100 { 00101 char reponse_vide[1]; 00102 return envoyerRequete(complement_url, reponse_vide, 1); 00103 } 00104 00105 void envoyerChaineSocket(char *chaine, int taille_chaine, char *reponse, int longueur_reponse_max) 00106 { 00107 int m; 00108 socket.send_all(chaine, taille_chaine); 00109 m = socket.receive(reponse,longueur_reponse_max); 00110 reponse[m] = '\0'; 00111 } 00112 00113
Generated on Wed Jul 13 2022 03:22:24 by
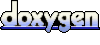