XBee API operation library for mbed for miniprojects
Dependencies: SmartLabXBeeCore
Fork of SmartLabXBeeAPI2 by
XBeeAPI Class Reference
XBee API library entry poiont. More...
#include <XBeeAPI.h>
Public Member Functions | |
XBeeAPI (PinName tx, PinName rx) | |
Create a XBeeAPI instance, with baud rate 9600 and no escape. | |
XBeeAPI (PinName tx, PinName rx, bool isEscape) | |
Create a XBeeAPI instance, with baud rate 9600. | |
XBeeAPI (PinName tx, PinName rx, int baudRate, bool isEscape) | |
Create a XBeeAPI instance. | |
XBeeAPI (ISerial *serial, bool isEscape) | |
Create a SerialData instance with other serial interface. |
Detailed Description
XBee API library entry poiont.
Example:
#include "mbed.h" #include "XBeeAPI.h" XBeeAPI xbee(p9, p10, true); Address add(0x0013A200,0x406CABD,0x0456); XBeeTx16Request tx16(0x00, &add, OptionsBase::DEFAULT, "Hello From XBEE API", 0, 19); XBeeRx16Indicator rx16(NULL); int main() { xbee.setVerifyChecksum(false); xbee.start(); // option 1 while(1) { XBeeRx16Indicator * response = xbee.getXBeeRx16(); if (response != NULL) { tx16.setPayload(response->getReceivedData(), 0, response->getReceivedDataLength()); xbee.send(&tx16); } } // option 2 while(1) { APIFrame * frame = xbee.getResponse(); while(frame != NULL) { switch (frame->getFrameType()) { case APIFrame::Tx64_Request : break; case APIFrame::Tx16_Request : break; case APIFrame::AT_Command: break; case APIFrame::AT_Command_Queue_Parameter_Value: break; case APIFrame::ZigBee_Transmit_Request: break; case APIFrame::Explicit_Addressing_ZigBee_Command_Frame: break; case APIFrame::Remote_Command_Request : break; case APIFrame::Create_Source_Route : break; case APIFrame::Register_Joining_Device: break; case APIFrame::Rx64_Receive_Packet : break; case APIFrame::Rx16_Receive_Packet: rx16.convert(frame); tx16.setPayload(rx16.getReceivedData(), 0, rx16.getReceivedDataLength()); xbee.send(&tx16); break; case APIFrame::Rx64_IO_Data_Sample_Rx_Indicator: break; case APIFrame::Rx16_IO_Data_Sample_Rx_Indicator : xbee.send(&tx16); break; case APIFrame::AT_Command_Response: break; case APIFrame::XBee_Transmit_Status : break; case APIFrame::Modem_Status: break; case APIFrame::ZigBee_Transmit_Status : break; case APIFrame::ZigBee_Receive_Packet: break; case APIFrame::ZigBee_Explicit_Rx_Indicator : break; case APIFrame::ZigBee_IO_Data_Sample_Rx_Indicator : break; case APIFrame::XBee_Sensor_Read_Indicato : break; case APIFrame::Node_Identification_Indicator: break; case APIFrame::Remote_Command_Response: break; case APIFrame::Over_the_Air_Firmware_Update_Status : break; case APIFrame::Route_Record_Indicator : break; case APIFrame::Device_Authenticated_Indicator: break; case APIFrame::Many_to_One_Route_Request_Indicator: break; } frame = xbee.getResponse(); } } }
Definition at line 105 of file XBeeAPI.h.
Constructor & Destructor Documentation
XBeeAPI | ( | PinName | tx, |
PinName | rx | ||
) |
Create a XBeeAPI instance, with baud rate 9600 and no escape.
- Parameters:
-
tx data transmission line rx data receiving line
Definition at line 3 of file XBeeAPI.cpp.
XBeeAPI | ( | PinName | tx, |
PinName | rx, | ||
bool | isEscape | ||
) |
Create a XBeeAPI instance, with baud rate 9600.
- Parameters:
-
tx data transmission line rx data receiving line isEscape API escaped operating mode (AP = 2) works similarly to API mode. The only difference is that when working in API escaped mode, some bytes of the API frame specific data must be escaped.
Definition at line 7 of file XBeeAPI.cpp.
XBeeAPI | ( | PinName | tx, |
PinName | rx, | ||
int | baudRate, | ||
bool | isEscape | ||
) |
Create a XBeeAPI instance.
- Parameters:
-
tx data transmission line rx data receiving line baudRate baud rate isEscape API escaped operating mode (AP = 2) works similarly to API mode. The only difference is that when working in API escaped mode, some bytes of the API frame specific data must be escaped.
Definition at line 11 of file XBeeAPI.cpp.
XBeeAPI | ( | ISerial * | serial, |
bool | isEscape | ||
) |
Create a SerialData instance with other serial interface.
- Parameters:
-
serial class that implementes ISerial interface. baudRate baud rate isEscape API escaped operating mode (AP = 2) works similarly to API mode. The only difference is that when working in API escaped mode, some bytes of the API frame specific data must be escaped.
Definition at line 15 of file XBeeAPI.cpp.
Generated on Sun Jul 17 2022 19:50:18 by
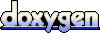