
Example main.c code for the Maxim Integrated DS7505 low voltage high accurate temperature sensor. Hosted on the MAX32630FTHR. C and C++ sample source code provided.
Dependencies: DS7505_Low_Voltage_Temperature_Sensor max32630fthr USBDevice
main.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2019 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "mbed.h" 00034 #include "max32630fthr.h" 00035 #include "ds7505.h" 00036 #include "ds7505_cpp.h" 00037 #include "USBSerial.h" 00038 00039 Serial pc(USBTX, USBRX); // Use USB debug probe for serial link 00040 Serial uart(P2_1, P2_0); 00041 void wait_sec_prompt(uint8_t time) 00042 { 00043 // Ports and serial connections 00044 uint32_t i; 00045 for (i = 0; i < time; i++) { 00046 pc.printf("."); 00047 wait(1); 00048 } 00049 pc.printf("\r\n"); 00050 } 00051 00052 00053 MAX32630FTHR pegasus(MAX32630FTHR::VIO_1V8); 00054 00055 DigitalOut rLED(LED1); 00056 DigitalOut gLED(LED2); 00057 DigitalOut bLED(LED3); 00058 00059 I2C i2cBus(P3_4, P3_5); 00060 00061 void blink_timer(void) { 00062 gLED = !gLED; /* blink the green LED */ 00063 } 00064 00065 // main() runs in its own thread in the OS 00066 // (note the calls to Thread::wait below for delays) 00067 /** 00068 * @brief Sample main program for DS7505 00069 * @version 1.0000.0000 00070 * 00071 * @details Sample main program for DS7505 00072 * The prints are sent to the terminal window (9600, 8n1). 00073 * The program sets the GPIOs to 1.8V and the program 00074 * configures the chip and reads temperatures. 00075 * To run the program, drag and drop the .bin file into the 00076 * DAPLINK folder. After it finishes flashing, cycle the power or 00077 * reset the Pegasus (MAX32630FTHR) after flashing by pressing the button on 00078 * the Pegasus next to the battery connector or the button 00079 * on the MAXREFDES100HDK. 00080 */ 00081 int main() 00082 { 00083 #define WAIT_TIME 8 00084 uint32_t i; 00085 float temperature; 00086 uint8_t cfg; 00087 DigitalOut rLED(LED1, LED_OFF); 00088 DigitalOut gLED(LED2, LED_OFF); 00089 DigitalOut bLED(LED3, LED_OFF); 00090 gLED = LED_ON; 00091 Ticker ticker; // calls a callback repeatedly with a timeout 00092 ticker.attach(callback(&blink_timer), 0.5f); /* set timer for 0.5 second */ 00093 pc.baud(9600); // Baud rate = 115200 00094 pc.printf("DS7505 Digital Thermometer and " 00095 "Thermostat example source code.\r\n"); 00096 pc.printf("\r\n"); 00097 uint8_t i2c_addr = DS7505_I2C_SLAVE_ADR_00; 00098 DS7505 temp_sensor(i2cBus, i2c_addr); 00099 i2cBus.frequency(400000); 00100 /* Configure for 9 bit, fault filter 6, 00101 active low polarity, comparator mode, continuous 00102 */ 00103 temp_sensor.write_cfg_reg(uint8_t( 00104 DS7505_CFG_RESOLUTION_9BIT | DS7505_CFG_FAULT_FILTER_6 | 00105 DS7505_CFG_OS_POLARITY_ACT_LOW | DS7505_CFG_COMPARATOR_MODE | 00106 DS7505_CFG_CONTINUOUS)); 00107 for (i = 0; i < 10; i++) { 00108 wait(DS7505_WAIT_CONV_TIME_9BIT); 00109 temperature = 00110 temp_sensor.read_reg_as_temperature(DS7505_REG_TEMPERATURE); 00111 pc.printf("Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00112 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00113 } 00114 temp_sensor.read_cfg_reg(&cfg); 00115 pc.printf("Configuration Register = 0x%02Xh \r\n", cfg); 00116 #if 0 00117 temp_sensor.write_trip_low_thyst(-63.9375); 00118 temperature = 00119 temp_sensor.read_reg_as_temperature(DS7505_REG_THYST_LOW_TRIP); 00120 pc.printf("Thyst Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00121 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00122 00123 temp_sensor.write_trip_high_tos(64.0625f); 00124 temperature = temp_sensor.read_reg_as_temperature(DS7505_REG_TOS_HIGH_TRIP); 00125 pc.printf("TOS Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00126 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00127 pc.printf("\r\n\r\n"); 00128 #endif 00129 00130 pc.printf("\r\n"); 00131 for (i = 0; i < 8; i++) { 00132 /* Configure to shutdown mode */ 00133 temp_sensor.write_cfg_reg(uint8_t( 00134 DS7505_CFG_RESOLUTION_12BIT | DS7505_CFG_FAULT_FILTER_4 | 00135 DS7505_CFG_OS_POLARITY_ACT_LOW | DS7505_CFG_INTERRUPT_MODE | 00136 DS7505_CFG_SHUTDOWN)); 00137 wait_sec_prompt(WAIT_TIME); /* leave it in shutdown mode for a while */ 00138 /* Configure for 12 BIT, fault filter 4, 00139 active low polarity, interrupt mode, continuous 00140 */ 00141 temp_sensor.write_cfg_reg(uint8_t( 00142 DS7505_CFG_RESOLUTION_12BIT | DS7505_CFG_FAULT_FILTER_4 | 00143 DS7505_CFG_OS_POLARITY_ACT_LOW | DS7505_CFG_INTERRUPT_MODE | 00144 DS7505_CFG_CONTINUOUS)); 00145 wait(DS7505_WAIT_CONV_TIME_12BIT); 00146 temperature = 00147 temp_sensor.read_reg_as_temperature(DS7505_REG_TEMPERATURE); 00148 pc.printf("Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00149 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00150 } 00151 temp_sensor.read_cfg_reg(&cfg); 00152 pc.printf("Configuration Register = 0x%02Xh \r\n", cfg); 00153 00154 pc.printf("\r\n\r\n"); 00155 00156 #if 0 00157 temp_sensor.write_trip_low_thyst(-55.0f); 00158 temperature = 00159 temp_sensor.read_reg_as_temperature(DS7505_REG_THYST_LOW_TRIP); 00160 pc.printf("Thyst Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00161 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00162 00163 temp_sensor.write_trip_high_tos(125.0f); 00164 temperature = temp_sensor.read_reg_as_temperature(DS7505_REG_TOS_HIGH_TRIP); 00165 pc.printf("TOS Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00166 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00167 pc.printf("\r\n\r\n"); 00168 #endif 00169 00170 /*************************************************************************** 00171 * Call the C code version of the driver 00172 *************************************************************************** 00173 */ 00174 #include "ds7505_c.h" 00175 pc.printf("C implementation of the code\r\n"); 00176 ds7505_init(i2c_addr); 00177 /* Configure for 9 bit, fault filter 6, 00178 active low polarity, comparator mode, continuous 00179 */ 00180 ds7505_write_cfg_reg(uint8_t( 00181 DS7505_CFG_RESOLUTION_9BIT | DS7505_CFG_FAULT_FILTER_6 | 00182 DS7505_CFG_OS_POLARITY_ACT_LOW | DS7505_CFG_COMPARATOR_MODE | 00183 DS7505_CFG_CONTINUOUS), i2cBus); 00184 for (i = 0; i < 10; i++) { 00185 wait(DS7505_WAIT_CONV_TIME_9BIT); 00186 temperature = ds7505_read_reg_as_temperature(DS7505_REG_TEMPERATURE, 00187 i2cBus); 00188 pc.printf("Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00189 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00190 } 00191 00192 ds7505_read_cfg_reg(&cfg, i2cBus); 00193 pc.printf("Configuration Register = 0x%02Xh \r\n", cfg); 00194 00195 #if 0 00196 ds7505_write_trip_low_thyst(-63.9375, i2cBus); 00197 temperature = ds7505_read_reg_as_temperature(DS7505_REG_THYST_LOW_TRIP, 00198 i2cBus); 00199 pc.printf("Thyst Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00200 temperature, ds7505_celsius_to_fahrenheit(temperature)); 00201 00202 ds7505_write_trip_high_tos(64.0625f, i2cBus); 00203 temperature = ds7505_read_reg_as_temperature(DS7505_REG_TOS_HIGH_TRIP, 00204 i2cBus); 00205 pc.printf("TOS Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00206 temperature, ds7505_celsius_to_fahrenheit(temperature)); 00207 #endif 00208 00209 pc.printf("\r\n"); 00210 for (i = 0; i < 8; i++) { 00211 /* Configure for shutdown mode */ 00212 ds7505_write_cfg_reg(uint8_t( 00213 DS7505_CFG_RESOLUTION_12BIT | DS7505_CFG_FAULT_FILTER_4 | 00214 DS7505_CFG_OS_POLARITY_ACT_LOW | DS7505_CFG_INTERRUPT_MODE | 00215 DS7505_CFG_SHUTDOWN), i2cBus); 00216 wait_sec_prompt(WAIT_TIME); /* leave it in shutdown mode for a while */ 00217 /* Configure for 12 bits, fault filter 4, 00218 active low polarity, interrupt mode, continuous 00219 */ 00220 ds7505_write_cfg_reg(uint8_t( 00221 DS7505_CFG_RESOLUTION_12BIT | DS7505_CFG_FAULT_FILTER_4 | 00222 DS7505_CFG_OS_POLARITY_ACT_LOW | DS7505_CFG_INTERRUPT_MODE | 00223 DS7505_CFG_CONTINUOUS), i2cBus); 00224 wait(DS7505_WAIT_CONV_TIME_12BIT); 00225 temperature = ds7505_read_reg_as_temperature(DS7505_REG_TEMPERATURE, 00226 i2cBus); 00227 pc.printf("Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00228 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00229 } 00230 ds7505_read_cfg_reg(&cfg, i2cBus); 00231 pc.printf("Configuration Register = 0x%02Xh \r\n", cfg); 00232 00233 #if 0 00234 ds7505_write_trip_low_thyst(-55, i2cBus); 00235 temperature = ds7505_read_reg_as_temperature(DS7505_REG_THYST_LOW_TRIP, 00236 i2cBus); 00237 pc.printf("Thyst Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00238 temperature, ds7505_celsius_to_fahrenheit(temperature)); 00239 00240 ds7505_write_trip_high_tos(125.0f, i2cBus); 00241 temperature = ds7505_read_reg_as_temperature(DS7505_REG_TOS_HIGH_TRIP, 00242 i2cBus); 00243 pc.printf("TOS Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00244 temperature, ds7505_celsius_to_fahrenheit(temperature)); 00245 #endif 00246 pc.printf("\r\n\r\n\r\n"); 00247 00248 00249 while (true) { 00250 } 00251 } 00252 00253
Generated on Wed Jul 20 2022 12:56:14 by
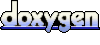